
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
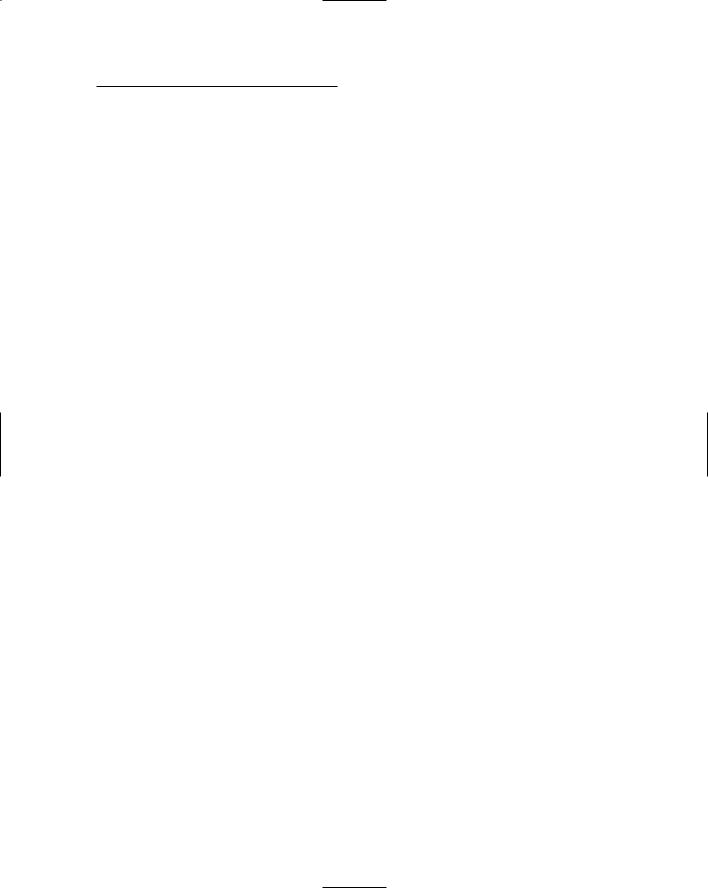
Chapter 26: Inheriting Multiple Inheritance 349
As an example of the latter, consider a TeacherAssistant who is both a Student and a Teacher, both of which are subclasses of Academician. If the university gives its teaching assistants two IDs — a student ID and a separate teacher ID — the class TeacherAssistant will need to contain two copies of class Academician.
Constructing the Objects
of Multiple Inheritance
The rules for constructing objects need to be expanded to handle multiple inheritance. The constructors are invoked in the following order:
1.First, the constructor for any virtual base classes is called in the order in which the classes are inherited.
2.Then the constructor for all nonvirtual base classes is called in the order in which the classes are inherited.
3.Next, the constructor for all member objects is called in the order in which the member objects appear in the class.
4.Finally, the constructor for the class itself is called.
Notice that base classes are constructed in the order in which they are inher ited and not in the order in which they appear on the constructor line.
Voicing a Contrary Opinion
I should point out that not all object-oriented practitioners think that multi ple inheritance is a good idea. In addition, many object-oriented languages don’t support multiple inheritance.
Multiple inheritance is not an easy thing for the language to implement. This is mostly the compiler’s problem (or the compiler writer’s problem). But mul tiple inheritance adds overhead to the code when compared to single inheri tance, and this overhead can become the programmer’s problem.
More importantly, multiple inheritance opens the door to additional errors. First, ambiguities such as those mentioned in the earlier section “Straightening Out Inheritance Ambiguities” pop up. Second, in the presence of multiple inher itance, casting a pointer from a subclass to a base class often involves chang ing the value of the pointer in sophisticated and mysterious ways. Let me leave the details to the language lawyers and compiler writers.
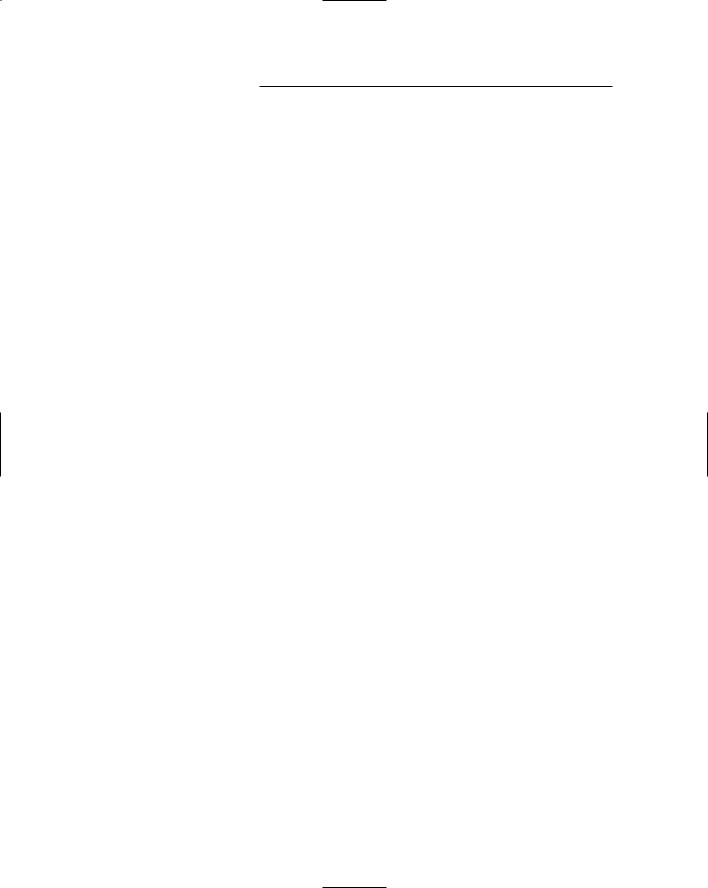
350 Part V: Optional Features
I suggest that you avoid using multiple inheritance until you’re comfortable with C++. Single inheritance provides enough expressive power to get used to. Later, you can study the manuals until you’re sure that you understand exactly what’s going on when you use multiple inheritance. One exception is the use of commercial libraries such as Microsoft’s Foundation Classes (MFC), which use multiple inheritance quite a bit. These classes have been checked out and are safe.
Don’t get me wrong. I’m not out and out against multiple inheritance. The fact that Microsoft and others use multiple inheritance effectively in their class libraries proves that it can be done. However, multiple inheritance is a fea ture that you want to hold off on using until you’re ready for it.
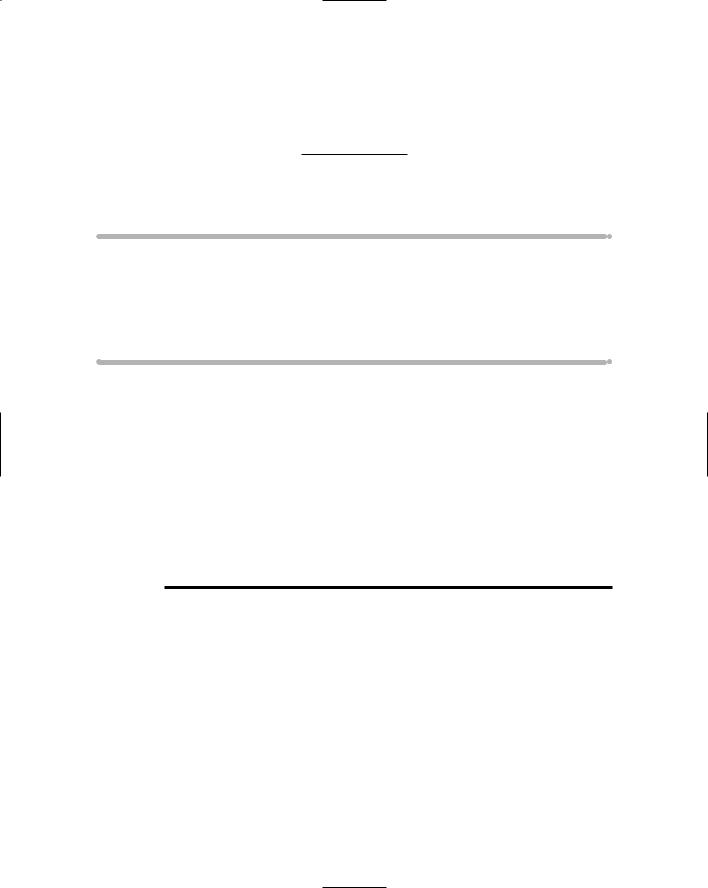
Chapter 27
Tempting C++ Templates
In This Chapter
Examining how templates can be applied to functions
Combining common functions into a single template definition
Defining a template or class
Reviewing the advantages of a template over the more generic “void” approach
The Standard C++ Library provides a set of basic functions. The C++ library presents a complete set of math, time, input/output, and DOS operations, to name just a few. Many of the earlier programs in this book use the so-called
character string functions defined in the include file strings.h. The argument types for many of these functions are fixed. For example, both of the arguments to strcpy(char*, char*) must be a pointer to a null-terminated character string — nothing else makes sense.
There are functions that are applicable to multiple types. Consider the example of the lowly max() function, which returns the maximum of two arguments. The function declarations in Table 27-1 all make sense.
Table 27-1 |
Possible Variants of maximum() Function |
|
Function Name |
|
Operation Performed |
maximum(int, int): |
Returns the maximum of two integers. |
|
|
|
|
maximum (unsigned |
|
Returns the maximum of two unsigned values. |
int, unsigned int) |
Because there are no negative numbers, the |
|
|
|
expression (0 - 1) “rolls over” to become a very |
|
|
large unsigned value, rather than the “normal” or |
|
|
signed value –1. |
|
|
|
Maximum |
|
Performs the same comparison operation but on |
(double, double) |
|
floating numbers. |
|
|
|
Maximum |
|
Returns the character that occurs later in the alpha- |
(char, char) |
|
bet (including all special characters). |
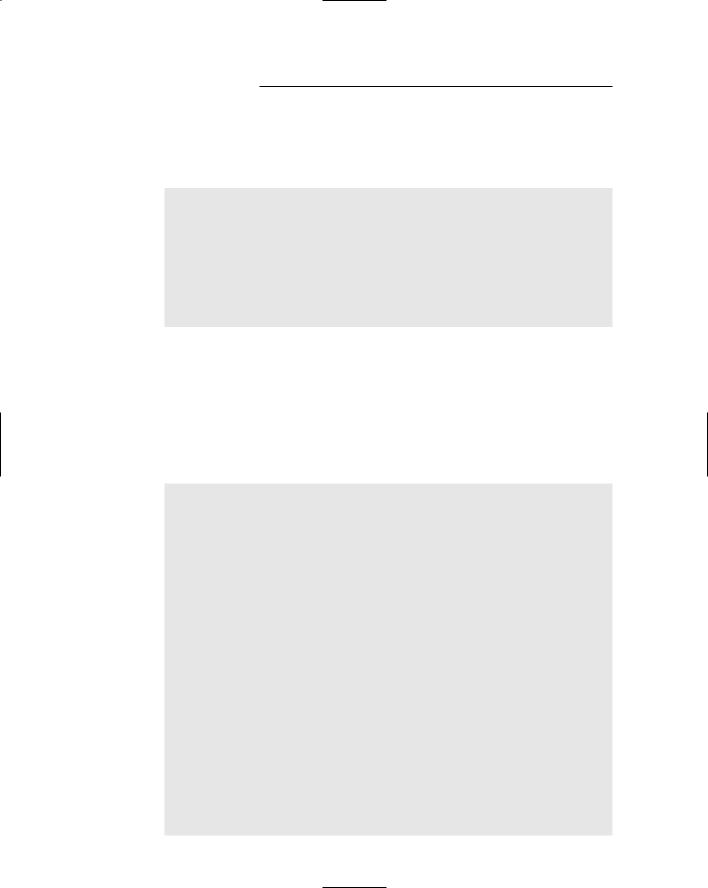
352 Part V: Optional Features
I would like to implement maximum() for all four cases. Of course, C++ can pro mote all of the types specified into double. Thus, you could argue that the maximum(double, double) version is all that is actually needed. Consider, however, what that would mean to the following expression:
//prototype a max() function double maximum(double, double);
//user function
void fn(int nArg1, int nArg2)
{
int nLarger = (int)maximum((double)nArg1, (double)nArg2);
// ...continue...
}
In this case, both nArg1 and nArg2 must be promoted to double with the accompanying loss of accuracy. This maximum() function returns a double. This value must be demoted from double back to an int via the cast before it can be assigned to nLarger. The function might work without loss of accu racy, but the numerous conversions take much more computer time than a silly maximum() function should. In any case, the function doesn’t work the way the user would expect or hope.
Of course, you could overload maximum() with all the possible versions:
double maximum(double d1, double d2)
{
if (d1 > d2)
{
return d1;
}
return d2;
}
int maximum(int n1, int n2)
{
if (n1 > n2)
{
return n1;
}
return n2;
}
char maximum(char c1, char c2)
{
if (c1 > c2)
{
return c1;
}
return c2;
}
// ...repeat for all other numeric types...