
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
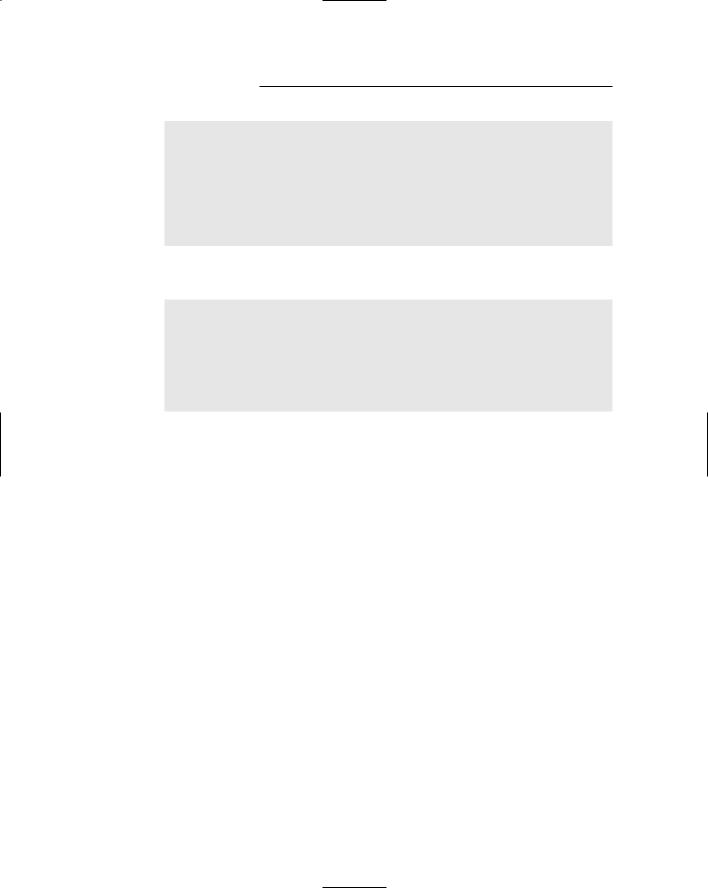
342 Part V: Optional Features
#include <iostream.h>
void fn()
{
SleeperSofa ss; cout << “weight = “
<< ss.weight // illegal - which weight? << “\n”;
}
The program must now indicate one of the two weights by specifying the desired base class. The following code snippet is correct:
#include <iostream.h> void fn()
{
SleeperSofa ss;
cout << “sofa weight = “
<<ss.Sofa::weight // specify which weight
<<“\n”;
}
Although this solution corrects the problem, specifying the base class in the application function isn’t desirable because it forces class information to leak outside the class into application code. In this case, fn() has to know that SleeperSofa inherits from Sofa. These types of so-called name collisions weren’t possible with single inheritance but are a constant danger with multi ple inheritance.
Adding Virtual Inheritance
In the case of SleeperSofa, the name collision on weight was more than a mere accident. A SleeperSofa doesn’t have a bed weight separate from its sofa weight. The collision occurred because this class hierarchy does not completely describe the real world. Specifically, the classes have not been completely factored.
Thinking about it a little more, it becomes clear that both beds and sofas are special cases of a more fundamental concept: furniture. (I suppose I could get even more fundamental and use something like object with mass, but furni ture is fundamental enough.) Weight is a property of all furniture. This rela tionship is shown in Figure 26-2.
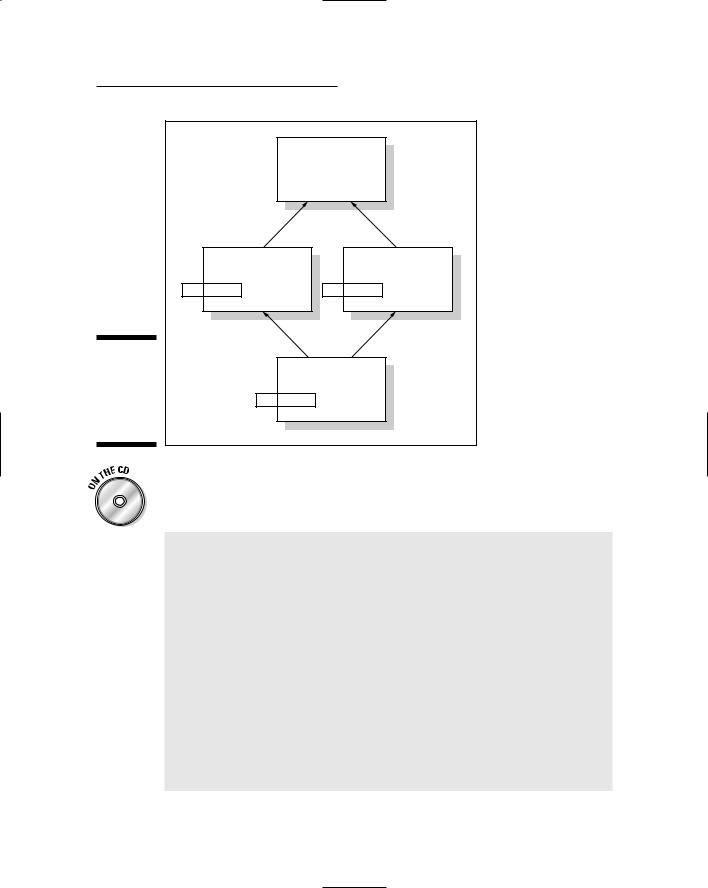
Chapter 26: Inheriting Multiple Inheritance 343
|
|
Furniture |
|
|
weight |
|
Bed |
Sofa |
|
sleep( ) |
watchTV( ) |
Figure 26-2: |
|
|
Further |
|
SleeperSofa |
factoring of |
|
|
|
|
|
beds and |
foldOut( ) |
|
sofas (by |
|
|
weight). |
|
|
Factoring out the class Furniture should relieve the name collision. With much relief and great anticipation of success, I generate the C++ class hierar chy shown in the following program, MultipleInheritanceFactoring:
//
//MultipleInheritanceFactoring - a single class can
// inherit from more than one base class
//
#include <cstdio> #include <cstdlib> #include <iostream> using namespace std;
//Furniture - more fundamental concept; this class
//has “weight” as a property
class Furniture
{
public:
Furniture(int w) : weight(w) {} int weight;
};
class Bed : public Furniture
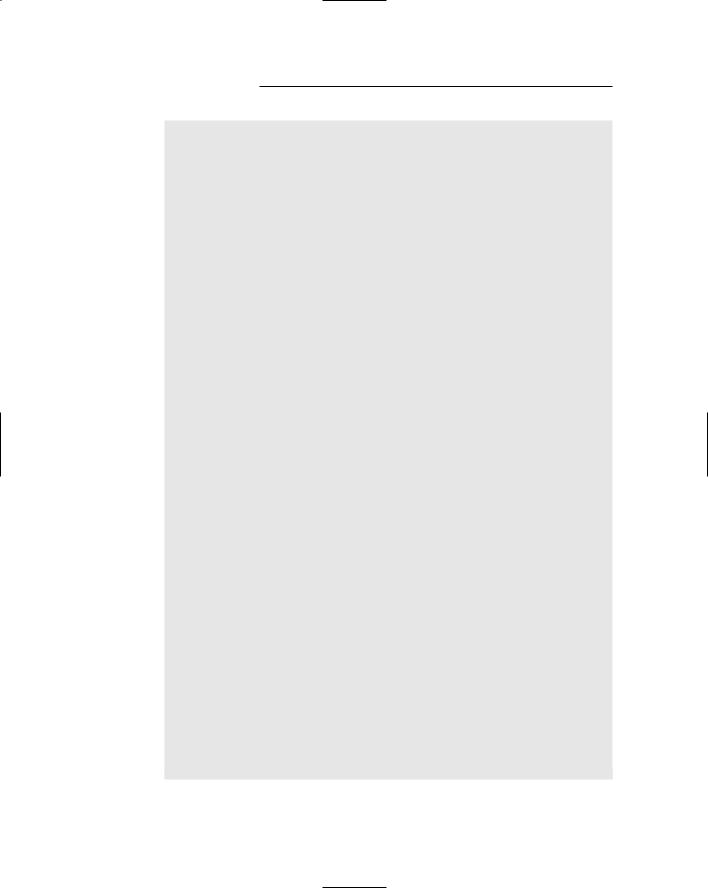
344 Part V: Optional Features
{
public:
Bed(int weight) : Furniture(weight) {} void sleep(){ cout << “Sleep” << endl; }
};
class Sofa : public Furniture
{
public:
Sofa(int weight) : Furniture(weight) {}
void watchTV(){ cout << “Watch TV” << endl; }
};
// SleeperSofa - is both a Bed and a Sofa class SleeperSofa : public Bed, public Sofa
{
public:
SleeperSofa(int weight) : Sofa(weight), Bed(weight) {} void foldOut(){ cout << “Fold out” << endl; }
};
int main(int nNumberofArgs, char* pszArgs[])
{
SleeperSofa ss(10);
//Section 1 -
//the following is ambiguous; is this a
//Furniture::Sofa or a Furniture::Bed?
/*
cout << “Weight = “
<<ss.weight
<<endl;
*/
//Section 2 -
//the following specifies the inheritance path
//unambiguously - sort of ruins the effect SleeperSofa* pSS = &ss;
Sofa* pSofa = (Sofa*)pSS;
Furniture* pFurniture = (Furniture*)pSofa; cout << “Weight = “
<<pFurniture->weight
<<endl;
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
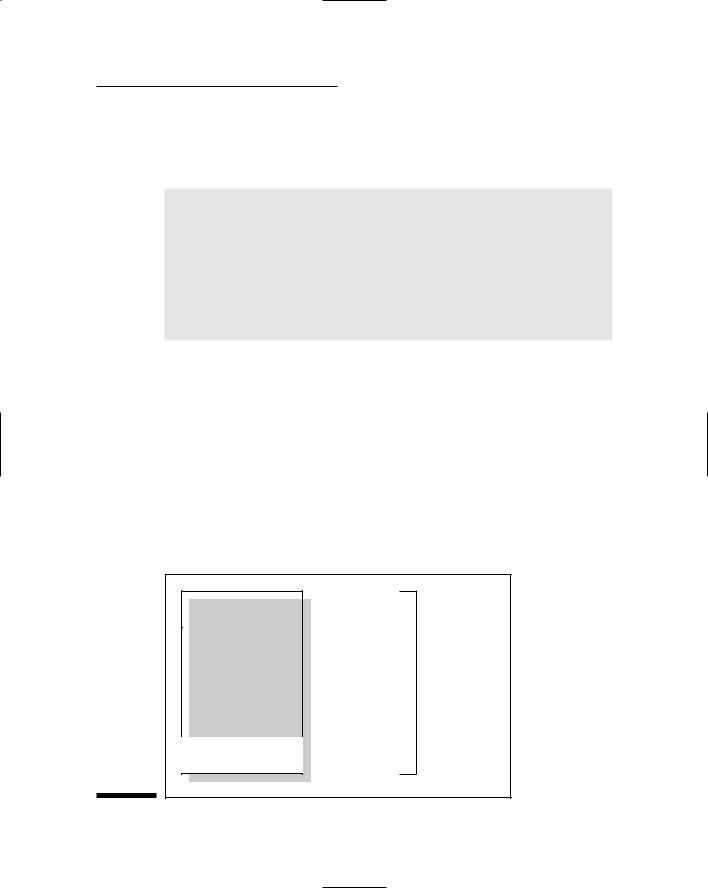
Chapter 26: Inheriting Multiple Inheritance 345
Imagine my dismay when I find that this doesn’t help at all — the reference to weight in Section 1 of main() is still ambiguous. (I wish my weight were as ambiguous!) “Okay,” I say (not really understanding why weight is still ambigu ous), “I’ll try casting ss to a Furniture.”
#include <iostream.h>
void fn()
{
SleeperSofa ss; Furniture* pF;
pF = (Furniture*)&ss; // use a Furniture pointer...
cout << “weight = “ // ...to get at the weight
<<pF->weight
<<“\n”;
};
Casting ss to a Furniture doesn’t work either. Now, I get some strange mes sage that the cast of SleeperSofa* to Furniture* is ambiguous. What’s going on?
The explanation is straightforward. SleeperSofa doesn’t inherit from
Furniture directly. Both Bed and Sofa inherit from Furniture and then
SleeperSofa inherits from them. In memory, a SleeperSofa looks like
Figure 26-3.
You can see that a SleeperSofa consists of a complete Bed followed by a complete Sofa followed by some SleeperSofa unique stuff. Each of these subobjects in SleeperSofa has its own Furniture part, because each inher its from Furniture. Thus, a SleeperSofa contains two Furniture objects!
|
|
Furniture |
|
|
|
|
|
|
|
|
|
|
|
|
the Bed part |
||
|
|
Bed stuff |
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
(without Furniture) |
|
|
|
|
|
a complete |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Furniture |
|
|
|
|
|
SleeperSofa |
|
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
object |
|
|
|
|
|
|
the Sofa part |
||
|
|
Sofa stuff |
||||||
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Figure 26-3: |
(without Furniture) |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
|
|
||
Memory |
SleeperSofa |
|
|
|
|
|
|
|
layout of a |
unique stuff |
|
|
|
|
|
|
SleeperSofa.
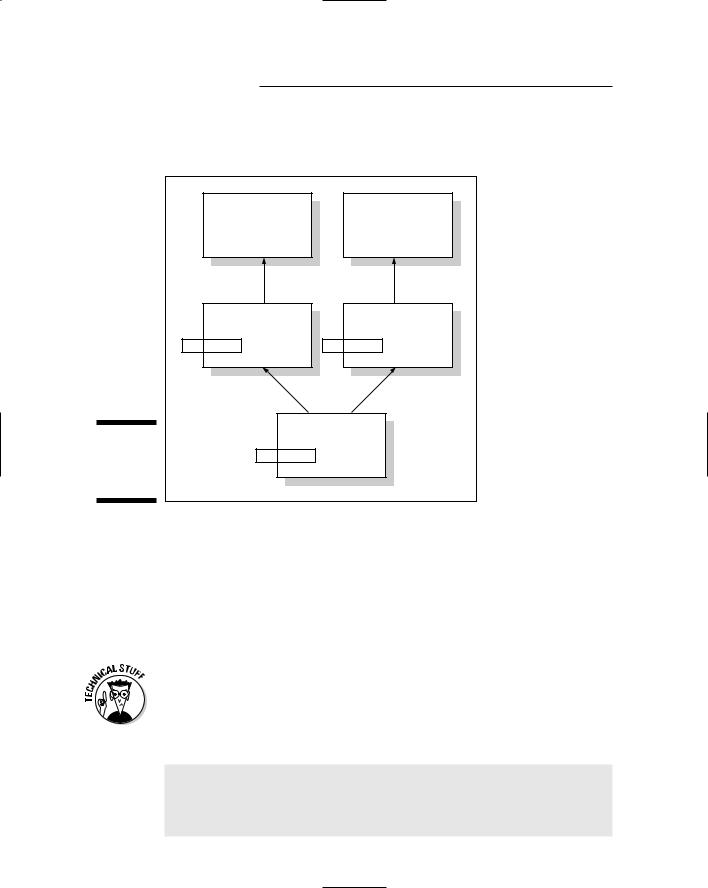
346 Part V: Optional Features
I haven’t created the hierarchy shown in Figure 26-2 after all. The inheritance hierarchy I have actually created is the one shown in Figure 26-4.
|
Furniture |
Furniture |
|
weight |
weight |
|
Bed |
Sofa |
|
sleep( ) |
watchTV( ) |
Figure 26-4: |
|
SleeperSofa |
|
|
|
Actual |
foldOut( ) |
|
result of my |
|
|
first attempt. |
|
|
The MultipleInheritanceFactoring program demonstrates this duplication of the base class. Section 2 specifies exactly which weight object by recasting the pointer SleeperSofa first to a Sofa* and then to a Furniture*.
But SleeperSofa containing two Furniture objects is nonsense.
SleeperSofa needs only one copy of Furniture. I want SleeperSofa to inherit only one copy of Furniture, and I want Bed and Sofa to share that one copy. C++ calls this virtual inheritance because it uses the virtual keyword.
I hate this overloading of the term virtual because virtual inheritance has nothing to do with virtual functions.
Armed with this new knowledge, I return to class SleeperSofa and imple ment it as follows:
//
//VirtualInheritance - using virtual inheritance the
//Bed and Sofa classes can share a common base
#include <cstdio>
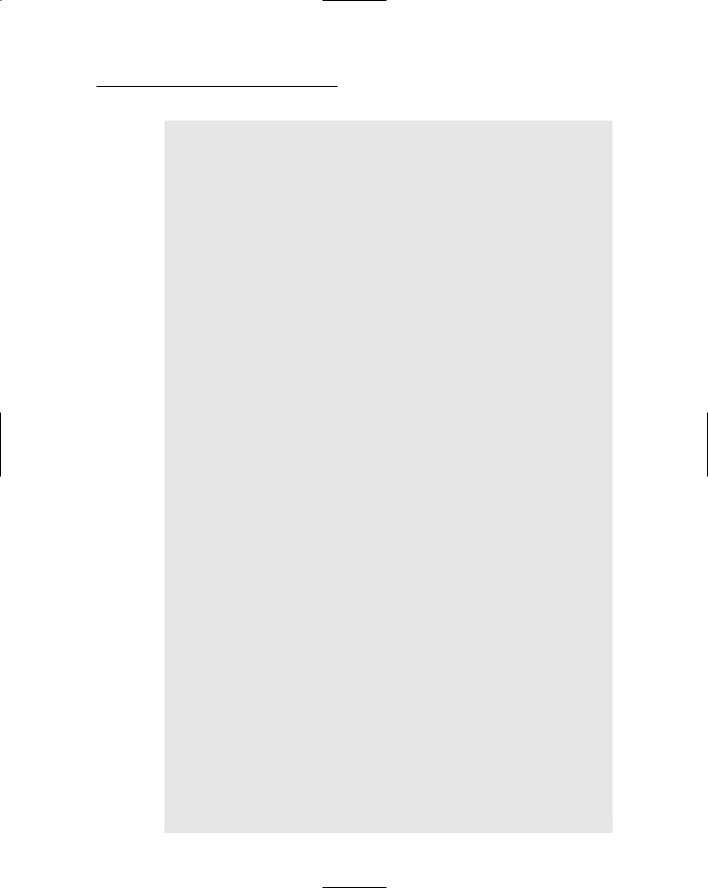
Chapter 26: Inheriting Multiple Inheritance 347
#include <cstdlib> #include <iostream> using namespace std;
//Furniture - more fundamental concept; this class
//has “weight” as a property
class Furniture
{
public:
Furniture(int w = 0) : weight(w) {} int weight;
};
class Bed : virtual public Furniture
{
public: Bed() {}
void sleep(){ cout << “Sleep” << endl; }
};
class Sofa : virtual public Furniture
{
public:
Sofa(){}
void watchTV(){ cout << “Watch TV” << endl; }
};
// SleeperSofa - is both a Bed and a Sofa class SleeperSofa : public Bed, public Sofa
{
public:
SleeperSofa(int weight) : Furniture(weight) {} void foldOut(){ cout << “Fold out” << endl; }
};
int main(int nNumberofArgs, char* pszArgs[])
{
SleeperSofa ss(10);
//Section 1 -
//the following is no longer ambiguous;
//there’s only one weight shared between Sofa and Bed
//Furniture::Sofa or a Furniture::Bed?
cout << “Weight = “
<<ss.weight
<<endl;
//Section 2 -
//the following specifies the inheritance path
//unambiguously - sort of ruins the effect SleeperSofa* pSS = &ss;
Sofa* pSofa = (Sofa*)pSS;
Furniture* pFurniture = (Furniture*)pSofa;
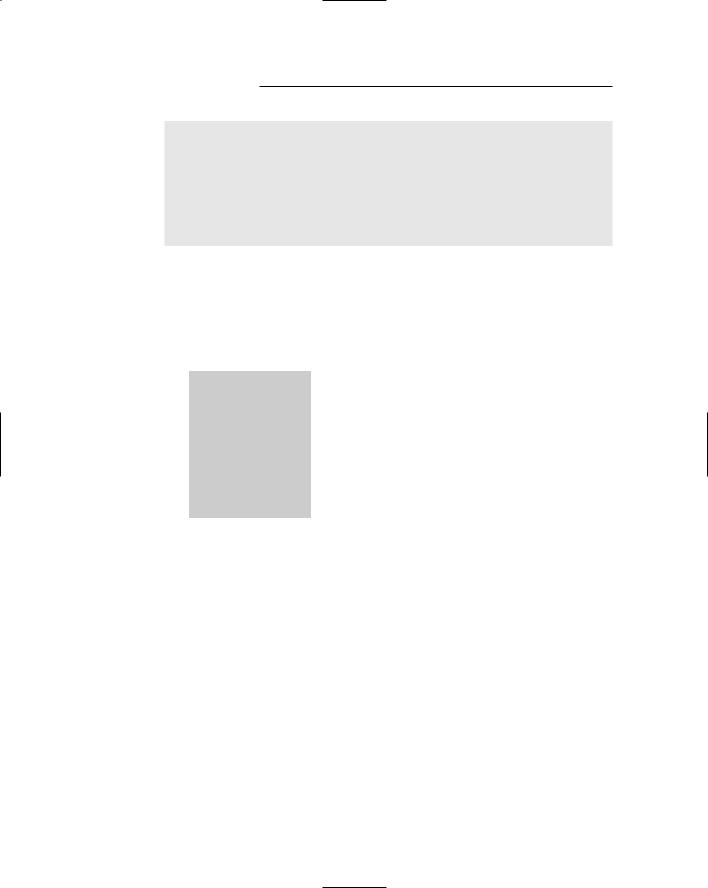
348 Part V: Optional Features
cout << “Weight = “
<<pFurniture->weight
<<endl;
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
Notice the addition of the keyword virtual in the inheritance of Furniture in Bed and Sofa. This says, “Give me a copy of Furniture unless you already have one somehow, in which case I’ll just use that one.” A SleeperSofa ends up looking like Figure 26-5 in memory.
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Furniture |
|
|
|
|
|
|
|
|
|
stuff |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Bed stuff |
|
|
|
|
a complete |
|
|
|
|
(without Furniture) |
|
|
|
|
|
|
|
|
|
|
|
||||
Figure 26-5: |
|
|
|
|
|
|
|
SleeperSofa |
|
|
|
|
|
|
|
|
|
||
|
|
|
Sofa stuff |
|
|
|
|
||
|
|
|
|
|
|
|
object |
||
Memory |
|
|
|
|
|
|
|
||
|
|
|
(without Furniture) |
|
|
|
|
|
|
layout of |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
SleeperSofa |
|
|
|
SleeperSofa |
|
|
|
|
|
with virtual |
|
|
|
unique stuff |
|
|
|
|
|
inheritance. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Here you can see that a SleeperSofa inherits Furniture, and then Bed minus the Furniture part, followed by Sofa minus the Furniture part. Bringing up the rear are the members unique to SleeperSofa. (Note that this may not be the order of the elements in memory, but that’s not important for the purpose of this discussion.)
Now the reference in fn() to weight is not ambiguous because a SleeperSofa contains only one copy of Furniture. By inheriting Furniture virtually, you get the desired inheritance relationship as expressed in Figure 26-2.
If virtual inheritance solves this problem so nicely, why isn’t it the norm? The first reason is that virtually inherited base classes are handled internally much differently than normally inherited base classes, and these differences involve extra overhead. The second reason is that sometimes you want two copies of the base class (although this is unusual).