
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
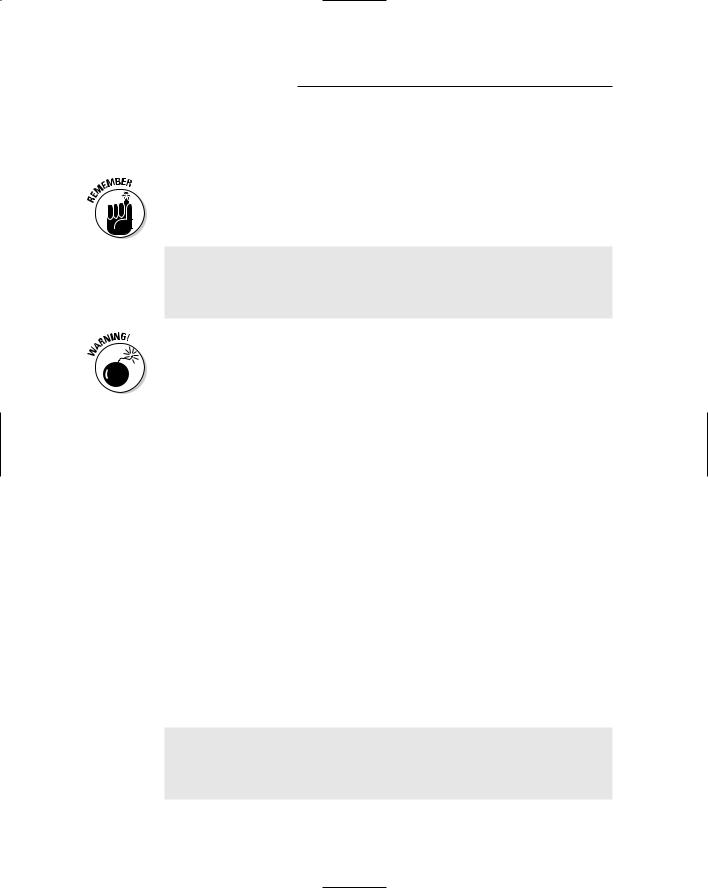
210 Part III: Introduction to Classes
Different types of objects are created at different times. Global objects are cre ated when the program first begins execution. Local objects are created when the program encounters their declaration.
A global object is one that is declared outside of a function. A local object is one that is declared within a function and is, therefore, local to the function. In the following example, the variable me is global, and the variable notMe is local to the function pickOne():
int me = 0; void pickOne()
{
int notMe;
}
According to the rules, global objects are initialized to all zeros when the program starts executing. Objects declared local to a function have no particular initial value. Having all data members have a random state may not be a valid condition for all classes.
C++ allows the class to define a special member function that is invoked auto matically when an object of that class is created. This member function, called the constructor, must initialize the object to a valid initial state. In addi tion, the class can define a destructor to handle the destruction of the object. These two functions are the topics of this chapter.
Using Constructors
The constructor is a member function that is called automatically when an object is created. Its primary job is to initialize the object to a legal initial value for the class. (It’s the job of the remaining member functions to ensure that the state of the object stays legal.)
Why you need constructors
You could initialize an object as part of the declaration — that’s the way the
C programmer would do it — for example:
struct Student
{
int semesterHours; float gpa;
};
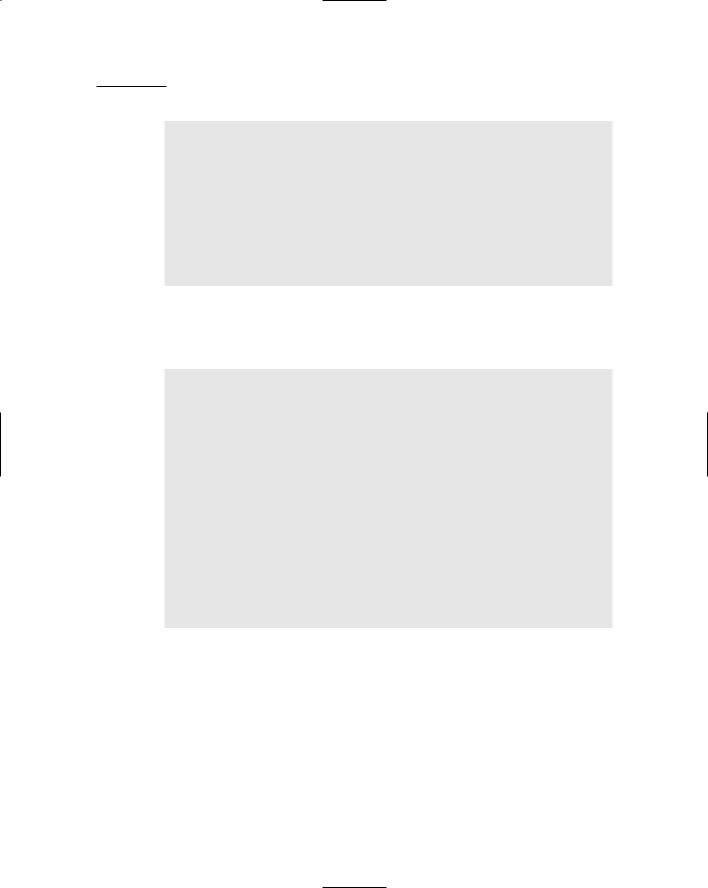
Chapter 16: “Why Do You Build Me Up, Just to Tear Me Down, Baby?” 211
void fn()
{
Student s1 = {0, 0.0};
// or Student s2;
s2.semesterHours = 0; s2.gpa = 0.0;
// ...function continues...
}
You could outfit the class with an initialization function that the application calls as soon as the object is created. Because this initialization function is a member of the class, it would have access to the protected members. This solution appears as follows:
class Student
{
public:
void init()
{
semesterHours = 0; gpa = 0.0;
}
// ...other public members...
protected:
int semesterHours; float gpa;
}; |
|
void fn() |
|
{ |
|
Student s; |
// create the object... |
s.init(); |
// ...then initialize it |
// ...function continues...
}
The only problem with this solution is that it abrogates the responsibility of the class to look after its own data members. In other words, the class must rely on the application to call the init() function. If it does not, the object is full of garbage, and who knows what might happen.
What is needed is a way to take the responsibility for calling the init() func tion away from the application code and give it to the compiler. Every time an object is created, the compiler can insert a call to the special init() function to initialize it. That’s a constructor!

212 Part III: Introduction to Classes
Making constructors work
The constructor is a special member function that’s called automatically when an object is created. It carries the same name as the class to differentiate it from the other members of the class. The designers of C++ could have made up a different rule, such as: “The constructor must be called init().” It wouldn’t have made any difference, as long as the compiler could recognize the constructor. In addition, the constructor has no return type, not even void, because it is only called automatically — if the constructor did return something, there would be no place to put it. A constructor cannot be invoked manually.
Constructing a single object
With a constructor, the class Student appears as follows:
//
// Constructor - example that invokes a constructor
//
#include <cstdio> #include <cstdlib> #include <iostream> using namespace std;
class Student
{
public:
Student()
{
cout << “constructing student” << endl; semesterHours = 0;
gpa = 0.0;
}
protected:
int semesterHours; float gpa;
};
int main(int nNumberofArgs, char* pszArgs[])
{
cout << “Creating a new Student object” << endl; Student s;
cout << “Creating a new object off the heap” << endl; Student* pS = new Student;
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}

Chapter 16: “Why Do You Build Me Up, Just to Tear Me Down, Baby?” 213
At the point of the declaration of s, the compiler inserts a call to the con structor Student::Student(). Allocating a new Student object from the heap has the same effect as demonstrated by the output from the program:
Creating a new Student object constructing student
Creating a new object off the heap constructing student
Press any key to continue . . .
This simple constructor was written as an inline member function. Constructors can be written also as outline functions, as shown here:
class Student
{
public:
Student();
// ...other public members...
protected:
int semesterHours; float gpa;
};
Student::Student()
{
cout << “constructing student” << endl; semesterHours = 0;
gpa = 0.0;
}
The output from this program can “prove” to you that constructors work as advertised, but to get the real effect, you really should single-step this simple program in your debugger. (See Chapter 10 for instructions on using the debugger.)
Single-step through this example until control comes to rest at the Student’s declaration. Select Step Into and control magically jumps to Student:: Student(). Continue single-stepping through the constructor. When the function has finished, control returns to the statement after the declaration.
In some cases, Step Into will actually execute the entire constructor without stopping. You may have to set a breakpoint in the constructor to get the effect. Setting a breakpoint always works.
Constructing multiple objects
Each element of an array must be constructed on its own. Making the follow ing simple change to the Constructor program contained in ConstructArray:
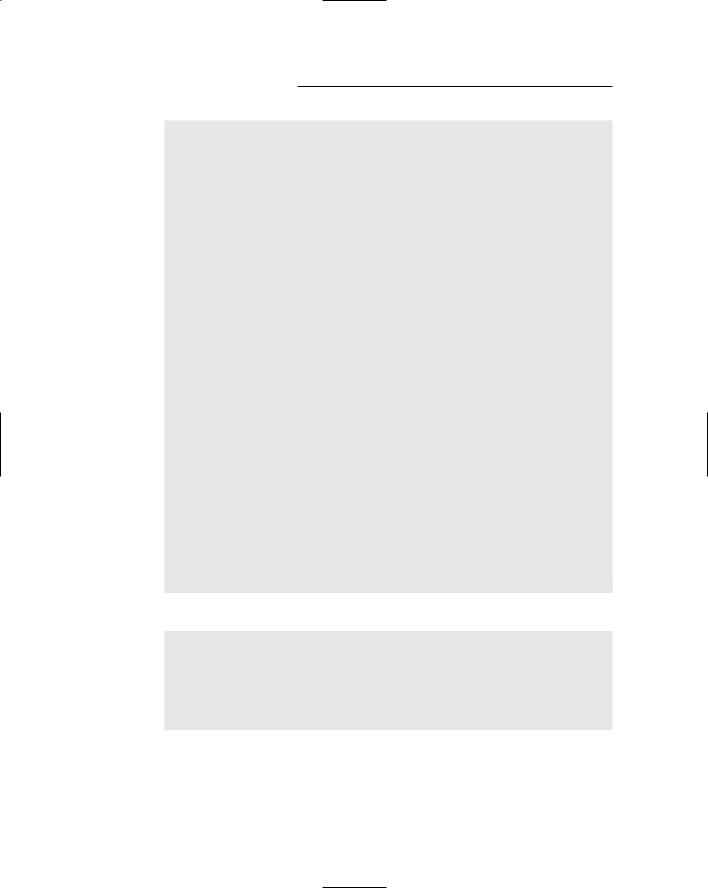
214 Part III: Introduction to Classes
//
//ConstructArray - example that invokes a constructor
// |
on an array of objects |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
#include <iostream> |
|
using namespace std; |
|
class Student |
|
{ |
|
public: |
|
Student() |
|
{
cout << “constructing student” << endl; semesterHours = 0;
gpa = 0.0;
}
// ...other public members...
protected:
int semesterHours; float gpa;
};
int main(int nNumberofArgs, char* pszArgs[])
{
cout << “Creating an array of 5 Student objects” << endl; Student s[5];
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
generates the following output:
Creating an array of 5 Student objects constructing student
constructing student constructing student constructing student constructing student
Press any key to continue . . .
Constructing a duplex
If a class contains a data member that is an object of another class, the con structor for that class is called automatically as well. Consider the following ConstructMembers example program. I added output statements so that you can see the order in which the objects are invoked.
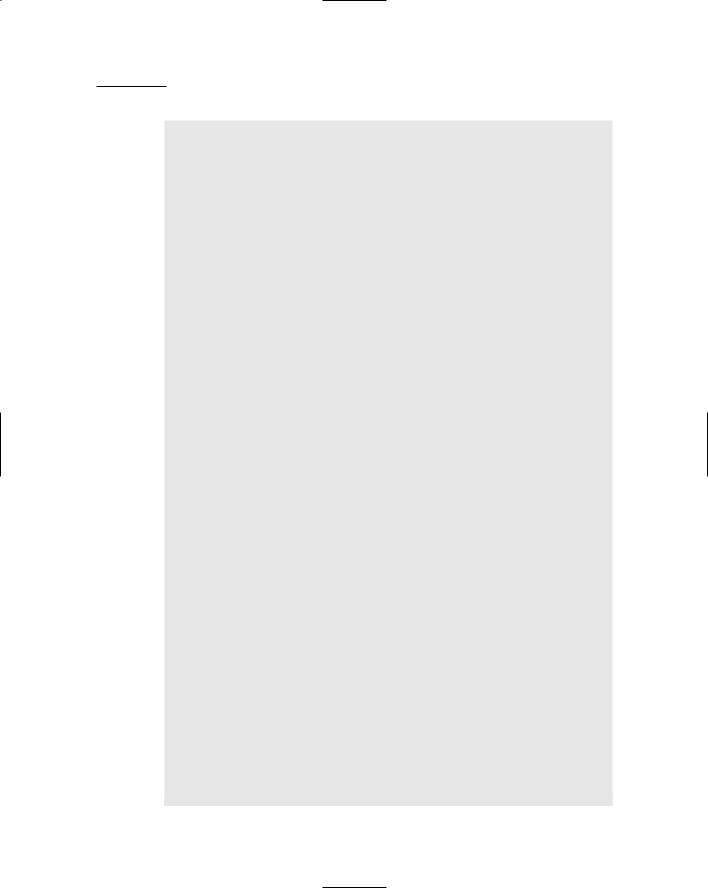
Chapter 16: “Why Do You Build Me Up, Just to Tear Me Down, Baby?” 215
//
//ConstructMembers - the member objects of a class
// |
are each constructed before the |
// |
container class constructor gets |
// |
a shot at it |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
#include <iostream> |
|
using namespace std; |
|
class Course |
|
{ |
|
public: |
|
Course() |
|
{ |
|
cout << “constructing course” << endl;
}
};
class Student
{
public:
Student()
{
cout << “constructing student” << endl; semesterHours = 0;
gpa = 0.0;
}
protected:
int semesterHours; float gpa;
};
class Teacher
{
public:
Teacher()
{
cout << “constructing teacher” << endl;
}
protected: Course c;
};
class TutorPair
{
public:
TutorPair()
{
cout << “constructing tutorpair” << endl; noMeetings = 0;
}

216 Part III: Introduction to Classes
protected: Student student; Teacher teacher;
int noMeetings;
};
int main(int nNumberofArgs, char* pszArgs[])
{
cout << “Creating TutorPair object” << endl; TutorPair tp;
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
Executing this program generates the following output:
Creating TutorPair object constructing student constructing course
constructing teacher constructing tutorpair
Press any key to continue . . .
Creating the object tp in main automatically invokes the constructor for TutorPair. Before control passes into the body of the TutorPair construc tor, however, the constructors for the two-member objects, student and teacher, are invoked.
The constructor for Student is called first because it is declared first. Then the constructor for Teacher is called.
The member Teacher.c of class Course is constructed as part of building the Teacher object. The Course constructor gets a shot first. Each object within a class must construct itself before the class constructor can be invoked. Otherwise, the main constructor would not know the state of its data members.
After all member data objects have been constructed, control returns to the open brace, and the constructor for TutorPair is allowed to construct the remainder of the object.
It would not do for TutorPair to be responsible for initializing Student and Teacher. Each class is responsible for initializing its own objects.