
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
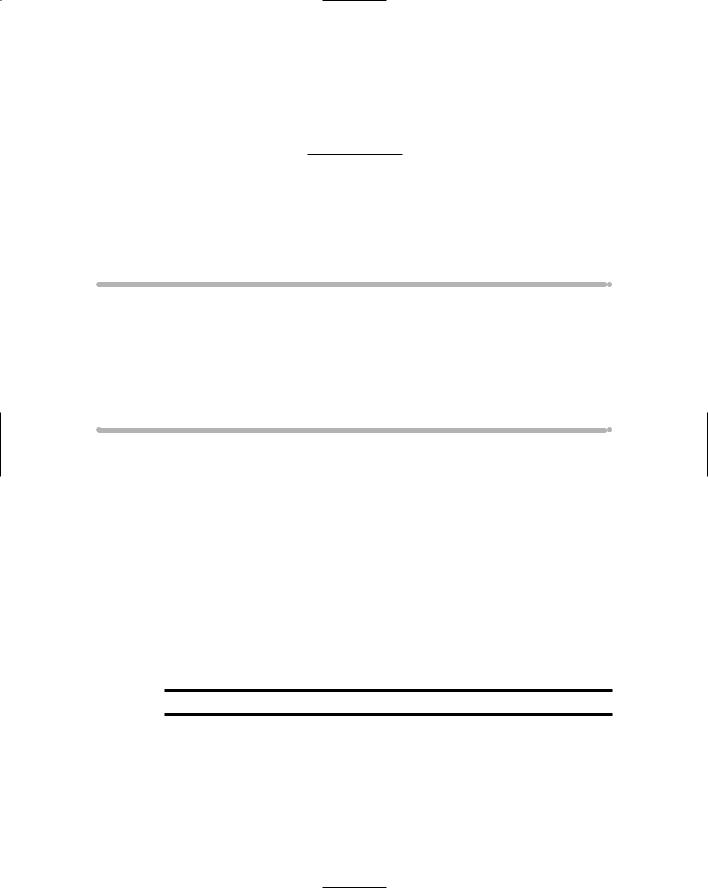
Chapter 9
Taking a Second Look
at C++ Pointers
In This Chapter
Performing arithmetic operations on character pointers
Examining the relationship between pointers and arrays
Applying this relationship to increase program performance
Extending pointer operations to different pointer types
Explaining the arguments to main() in our C++ program template
C++ allows the programmer to operate on pointer variables much as she would on simple types of variables. (The concept of pointer variables is
introduced in Chapter 8.) How and why this is done along with its implica tions are the subjects of this chapter.
Defining Operations on Pointer Variables
Some of the same operators I cover in Chapter 3 can be applied to pointer types. This section examines the implications of applying these operators to both to pointers and to the array types (I discuss arrays in Chapter 7). Table 9-1 lists the three fundamental operations that are defined on pointers.
Table 9-1 The Three Operations Defined on Pointer Types
Operation |
Result |
Meaning |
pointer |
pointer |
Calculate the address of the object |
+ offset |
|
integer entries from pointer |
pointer - offset |
pointer |
The opposite of addition |
|
|
|
pointer2 |
offset |
Calculate the number of entries |
- pointer1 |
|
between pointer2 and pointer1 |
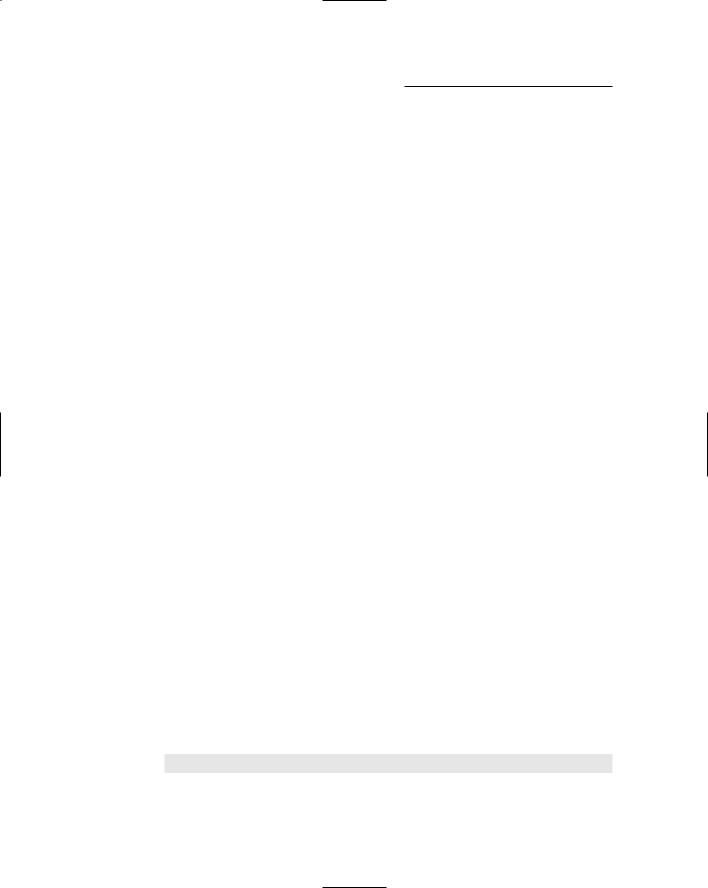
126 Part II: Becoming a Functional C++ Programmer
In this case, offset is of type long. (Although not listed in Table 9-1, C++ also supports operators related to addition and subtraction, such as ++ and +=.)
The real estate memory model (which I use so effectively in Chapter 8, if I do say so myself) is useful to explain how pointer arithmetic works. Consider a city block in which all houses are numbered sequentially. The house next to 123 Main Street has the address 124 Main Street (or 122 if you go backward, like Hebrew and Arabic).
Now it’s pretty clear that the house four houses down from 123 Main Street must be 127 Main Street; thus, you can say 123 Main + 4 = 127 Main. Similarly, if I were to ask how many houses are there from 123 Main to 127 Main, the answer would be four — 127 Main - 123 Main = 4. (Just as an aside, a house is zero houses from itself: 123 Main - 123 Main = 0.)
Extending this concept one step further, it makes no sense to add 123 Main Street to 127 Main Street. In similar fashion, you can’t add two addresses, nor can you multiply an address, divide an address, square an address, or take the square root — you get the idea.
Re-examining arrays in light of pointer variables
Now return to the wonderful array for just a moment. Once again, my neigh borhood comes to mind. An array is just like my city block. Each element of the array corresponds to a house on that block. Here, however, the array ele ments are measured by the number of houses from the beginning of the block (the street corner). Say that the house right on the corner is 123 Main Street, which means that the house one house from the corner is 124 Main Street, and so on. Using array terminology, you would say cityBlock[0] is 123 Main Street, cityBlock[1] is 124 Main Street, and so on.
Take that same model back to the world of computer memory. Consider the case of an array of 32 1-byte characters called charArray. If the first byte of this array is stored at address 0x110, the array will extend over the range 0x110 through 0x12f. charArray[0] is located at address 0x110, charArray[1] is at 0x111, charArray[2] at 0x112, and so on.
Take this model one step further to the world of pointer variables. After exe cuting the expression
ptr = &charArray[0];
the pointer ptr contains the address 0x110. The addition of an integer offset to a pointer is defined such that the relationships shown in Table 9-2 are true. Table 9-2 also demonstrates why adding an offset n to ptr calculates the address of the nth element in charArray.
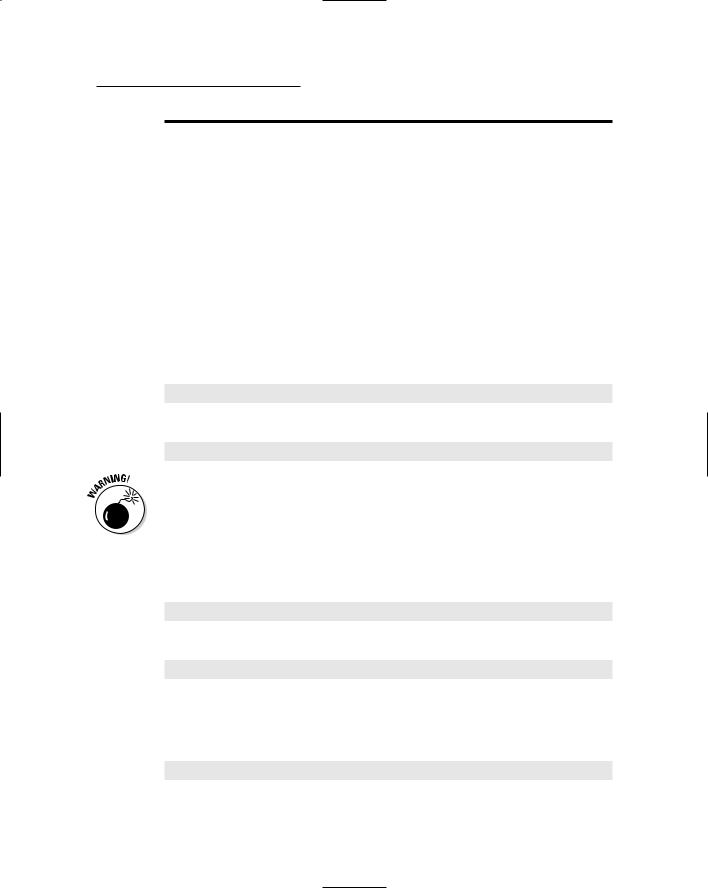
Chapter 9: Taking a Second Look at C++ Pointers 127
Table 9-2 |
|
Adding Offsets |
Offset |
Result |
Is the Address of |
+ 0 |
0x110 |
charArray[0] |
|
|
|
+ 1 |
0x111 |
charArray[1] |
|
|
|
+ 2 |
0x112 |
charArray[2] |
|
|
|
... |
... |
... |
|
|
|
+ n |
0x110 + n |
charArray[n] |
|
|
|
The addition of an offset to a pointer is similar to applying an index to an array.
Thus, if
char* ptr = &charArray[0];
then
*(ptr + n) ← corresponds with → charArray[n]
Because * has higher precedence than addition, * ptr + n adds n to the character that ptr points to. The parentheses are needed to force the addi tion to occur before the indirection. The expression *(ptr + n) retrieves the character pointed at by the pointer ptr plus the offset n.
In fact, the correspondence between the two forms of expression is so strong that C++ considers array[n] nothing more than a simplified version of *(ptr + n), where ptr points to the first element in array.
array[n] -- C++ interprets as → *(&array[0] + n)
In order to complete the association, C++ takes a second shortcut. If given
char charArray[20];
charArray is defined as &charArray[0];.
That is, the name of an array without a subscript present is the address of the array itself. Thus, you can further simplify the association to
array[n] --> C++ interprets as --> *(array + n)

128 Part II: Becoming a Functional C++ Programmer
Applying operators to the address of an array
The correspondence between indexing an array and pointer arithmetic is useful.
For example, a displayArray() function used to display the contents of an array of integers can be written as follows:
// displayArray - display the members of an // array of length nSize void displayArray(int intArray[], int nSize)
{
cout << “The value of the array is:\n”;
for(int n; n < nSize; n++)
{
cout << n << “: “ << intArray[n] << “\n”;
}
cout << “\n”;
}
This version uses the array operations with which you are familiar. A pointer version of the same appears as follows:
// displayArray - display the members of an // array of length nSize void displayArray(int intArray[], int nSize)
{
cout << “The value of the array is:\n”;
int* pArray = intArray;
for(int n = 0; n < nSize; n++, pArray++)
{
cout << n << “: “ << *pArray << “\n”;
}
cout << “\n”;
}
The new displayArray() begins by creating a pointer to an integer pArray that points at the first element of intArray.
The p in the variable name indicates that the variable is a pointer, but this is just a convention, not a part of the C++ language.
The function then loops through each element of the array. On each loop, displayArray() outputs the current integer (that is, the integer pointed at by pArray) before incrementing the pointer to the next entry in intArray. displayArray() can be tested using the following version of main():