
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
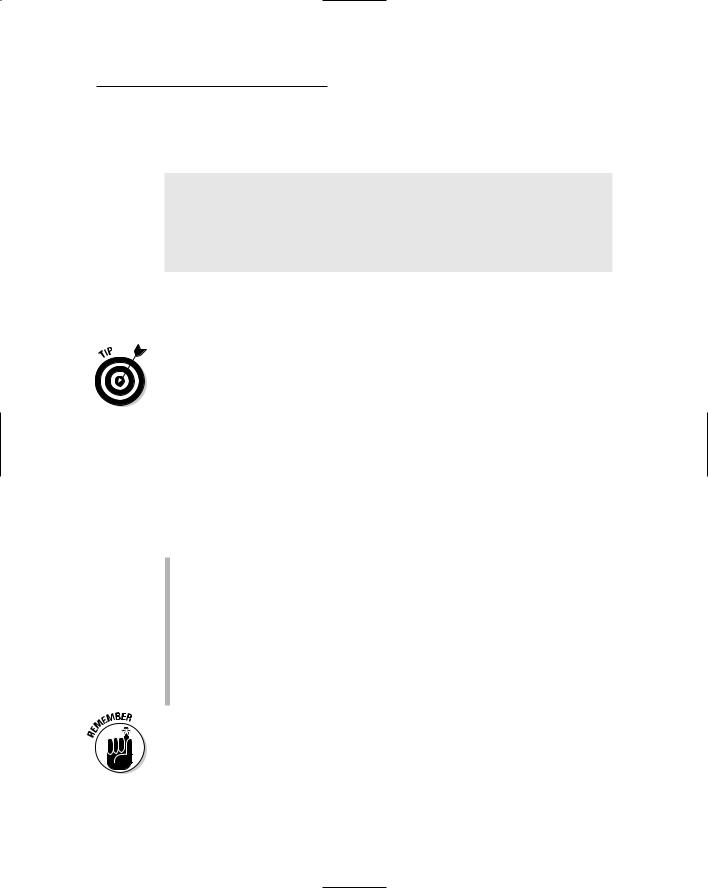
Chapter 17: Making Constructive Arguments 233
be changed thereafter. How can the constructor assign a const data member a value? The problem is solved with the same “colon syntax” used to initialize complex objects.
class Mammal
{
public:
Mammal(int nof) : numberOfFeet(nof) {} protected:
const int numberOfFeet;
};
Ostensibly, a given Mammal has a fixed number of feet (barring amputation). The number of feet can, and should, be declared const. This declaration assigns a value to the variable numberOfFeet when the object is created. The numberOfFeet cannot be modified once it’s been declared and initialized.
Programmers commonly use the “colon syntax” to initialize even non-const data members. Doing so isn’t necessary, but it’s common practice.
Constructing the Order of Construction
When there are multiple objects, all with constructors, programmers usually don’t care about the order in which things are built. If one or more of the con structors has side effects, however, the order can make a difference.
The rules for the order of construction are as follows:
Local and static objects are constructed in the order in which their dec larations are invoked.
Static objects are constructed only once.
All global objects are constructed before main().
Global objects are constructed in no particular order.
Members are constructed in the order in which they are declared in the class.
Destructors are invoked in the reverse order from constructors.
A static variable is a variable that is local to a function but retains its value from one function invocation to the next. A global variable is a variable declared outside a function.
Now, consider each of the preceding rules in turn.
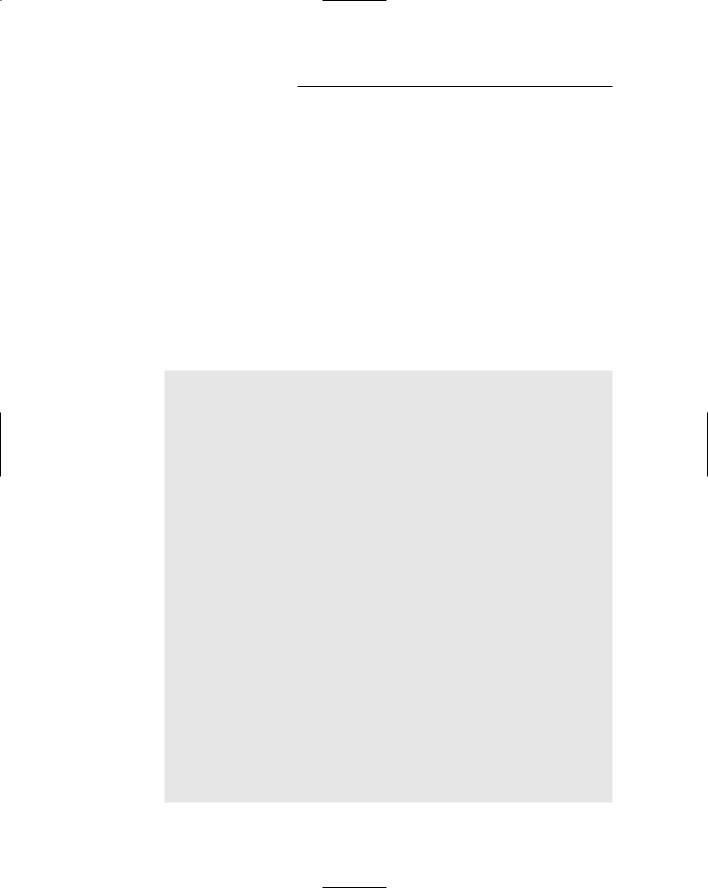
234 Part III: Introduction to Classes
Local objects construct in order
Local objects are constructed in the order in which the program encounters their declaration. Normally, this is the same as the order in which the objects appear in the function, unless the function jumps around particular declara tions. (By the way, jumping around declarations is a bad thing. It confuses the reader and the compiler.)
Static objects construct only once
Static objects are similar to local variables, except that they are constructed only once. C++ must wait until the first time control passes through the static’s before constructing the object. Consider the following trivial ConstructStatic program:
//
//ConstructStatic - demonstrate that statics are only
// |
constructed once |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
#include <iostream> |
|
using namespace std; |
|
class DoNothing
{
public:
DoNothing(int initial)
{
cout << “DoNothing constructed with a value of “
<<initial
<<endl;
}
};
void fn(int i)
{
cout << “Function fn passed a value of “ << i << endl; static DoNothing dn(i);
}
int main(int argcs, char* pArgs[])
{
fn(10);
fn(20);
system(“PAUSE”); return 0;
}
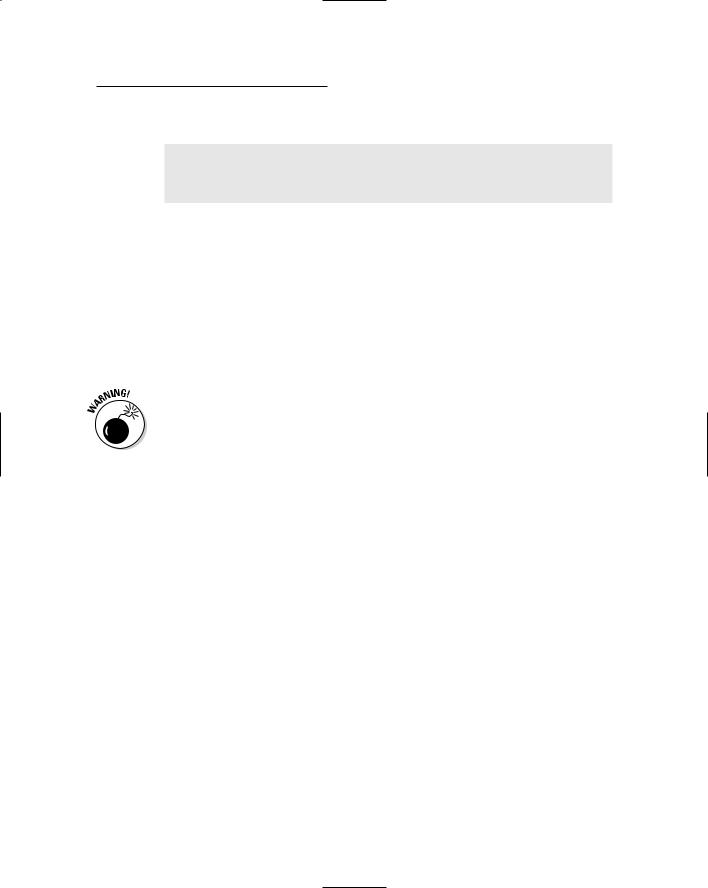
Chapter 17: Making Constructive Arguments 235
Executing this program generates the following results:
Function fn passed a value of 10
DoNothing constructed with a value of 10
Function fn passed a value of 20
Press any key to continue . . .
Notice that the message from the function fn() appears twice, but the mes sage from the constructor for DoNothing appears only the first time fn() is called. This indicates that the object is constructed the first time that fn() is called, but not thereafter.
All global objects construct before main()
All global variables go into scope as soon as the program starts. Thus, all global objects are constructed before control is passed to main().
Initializing global variables can cause real debugging headaches. Some debug gers try to execute up to main() as soon as the program is loaded and before they hand over control to the user. This can be a problem because the con structor code for all global objects has already been executed by the time you can wrest control of your program. If one of these constructors has a fatal bug, you never even get a chance to find the problem. In this case, the program appears to die before it even starts!
You can approach this problem in several ways. One is to test each construc tor on local objects before using it on globals. If that doesn’t solve the prob lem, you can try adding output statements to the beginning of all suspected constructors. The last output statement you see probably came from the flawed constructor.
Global objects construct in no particular order
Figuring out the order of construction of local objects is easy. An order is implied by the flow of control. With globals, no such flow is available to give order. All globals go into scope simultaneously — remember? Okay, you argue, why can’t the compiler just start at the top of the file and work its way down the list of global objects?
That would work fine for a single file (and I presume that’s what most compil ers do). Unfortunately, most programs in the real world consist of several files that are compiled separately and then linked. Because the compiler has no control over the order in which these files are linked, it cannot affect the order in which global objects are constructed from file to file.
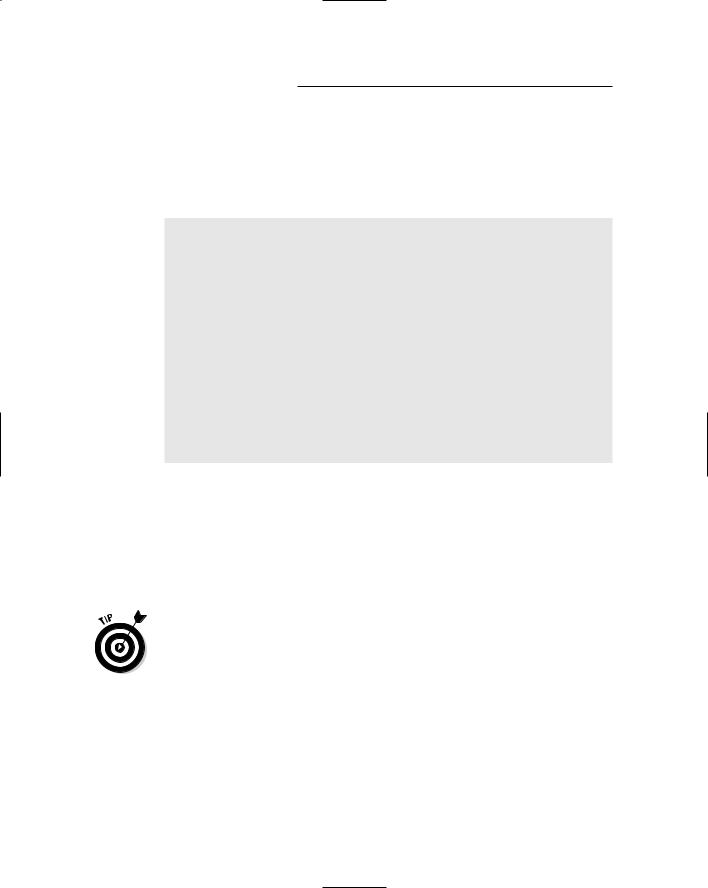
236 Part III: Introduction to Classes
Most of the time, the order of global construction is pretty ho-hum stuff. Once in a while, though, global variables generate bugs that are extremely difficult to track down. (It happens just often enough to make it worth mentioning in a book.)
Consider the following example:
class Student
{
public:
Student (int id) : studentId(id) {} const int studentId;
};
class Tutor
{
public:
Tutor(Student& s) : tutoredId(s.studentId) {} int tutoredId;
};
//set up a student Student randy(1234);
//assign that student a tutor Tutor jenny(randy);
Here the constructor for Student assigns a student ID. The constructor for Tutor records the ID of the student to help. The program declares a student randy and then assigns that student a tutor jenny.
The problem is that the program makes the implicit assumption that randy is constructed before jenny. Suppose that it were the other way around. Then jenny would be constructed with a block of memory that had not yet been turned into a Student object and, therefore, had garbage for a student ID.
The preceding example is not too difficult to figure out and more than a little contrived. Nevertheless, problems deriving from global objects being con structed in no particular order can appear in subtle ways. To avoid this prob lem, don’t allow the constructor for one global object to refer to the contents of another global object.
Members construct in the order in which they are declared
Members of a class are constructed according to the order in which they’re declared within the class. This isn’t quite as obvious as it may sound. Consider the following example: