
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
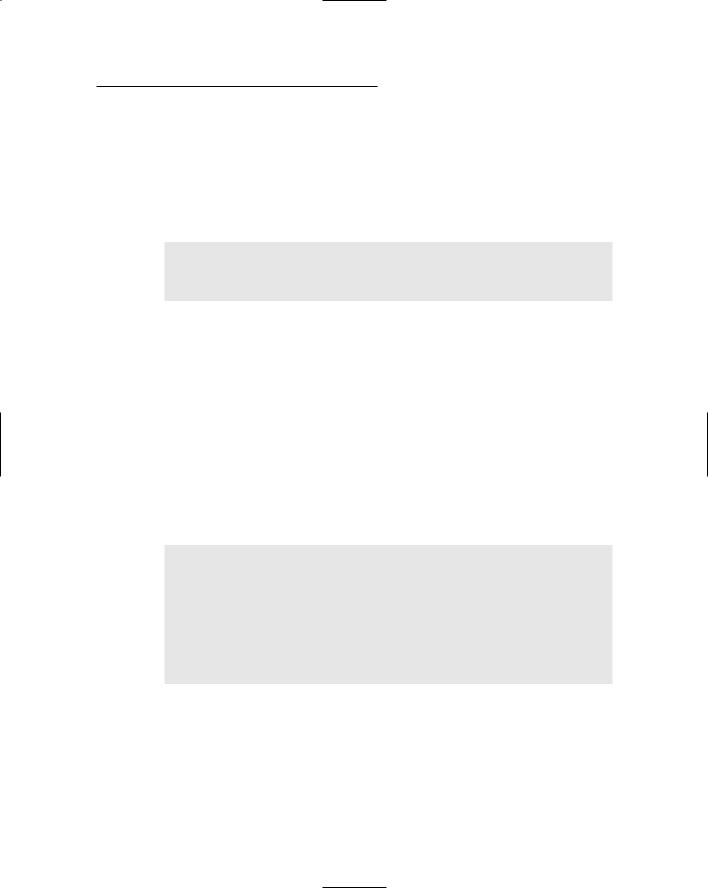
Chapter 5: Controlling Program Flow |
67 |
Using the for loop
The most common form of loop is the for loop. The for loop is preferred over the more basic while loop because it’s generally easier to read (there’s really no other advantage).
The for loop has the following format:
for (initialization; conditional; increment)
{
// ...body of the loop
}
Execution of the for loop begins with the initialization clause, which got its name because it’s normally where counting variables are initialized. The ini tialization clause is only executed once when the for loop is first encountered.
Execution continues with the conditional clause. This clause works a lot like the while loop: as long as the conditional clause is true, the for loop con tinues to execute.
After the code in the body of the loop finishes executing, control passes to the increment clause before returning to check the conditional clause — thereby repeating the process. The increment clause normally houses the autoincrement or autodecrement statements used to update the counting variables.
The following while loop is equivalent to the for loop:
{
initialization;
while(conditional)
{
{
// ...body of the loop
}
increment;
}
}
All three clauses are optional. If the initialization or increment clauses are missing, C++ ignores them. If the conditional clause is missing, C++ performs the for loop forever (or until something else passes control outside the loop).
The for loop is best understood by example. The following ForDemo program is nothing more than the WhileDemo converted to use the for loop construct:
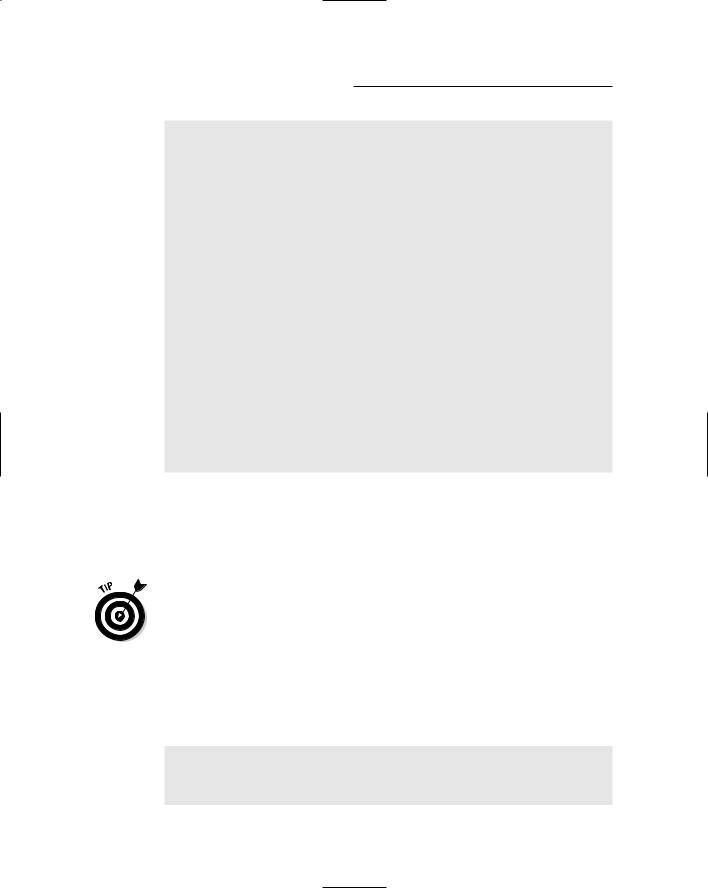
68 |
Part I: Introduction to C++ Programming |
//ForDemo1 - input a loop count. Loop while
//outputting astring arg number of times. #include <cstdio>
#include <cstdlib> #include <iostream> using namespace std;
int main(int nNumberofArgs, char* pszArgs[])
{
//input the loop count int loopCount;
cout << “Enter loopCount: “; cin >> loopCount;
//count up to the loop count limit for (; loopCount > 0;)
{
loopCount = loopCount - 1;
cout << “Only “ << loopCount << “ loops to go\n”;
}
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
The program reads a value from the keyboard into the variable loopCount. The for starts out comparing loopCount to zero. Control passes into the for loop if loopCount is greater than zero. Once inside the for loop, the pro
gram decrements loopCount and displays the result. That done, the program returns to the for loop control. Control skips to the next line after the for loop as soon as loopCount has been decremented to zero.
All three sections of a for loop may be empty. An empty initialization or increment section does nothing. An empty comparison section is treated like a comparison that returns true.
This for loop has two small problems. First, it’s destructive — not in the sense of what my puppy does to a slipper, but in the sense that it changes the value of loopCount, “destroying” the original value. Second, this for loop counts “backward” from large values down to smaller values. These two problems are addressed if you add a dedicated counting variable to the for loop. Here’s what it looks like:
//ForDemo2 - input a loop count. Loop while
//outputting astring arg number of times. #include <cstdio>
#include <cstdlib>

Chapter 5: Controlling Program Flow |
69 |
#include <iostream> using namespace std;
int main(int nNumberofArgs, char* pszArgs[])
{
//input the loop count int loopCount;
cout << “Enter loopCount: “; cin >> loopCount;
//count up to the loop count limit for (int i = 1; i <= loopCount; i++)
{
cout << “We’ve finished “ << i << “ loops\n”;
}
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
This modified version of WhileDemo loops the same as it did before. Instead of modifying the value of loopCount, however, this ForDemo2 version uses a counter variable.
Control begins by declaring a variable and initializing it to the value contained in loopCount. It then checks the variable i to make sure that it is positive. If so, the program executes the output statement, decrements i and starts over.
When declared within the initialization portion of the for loop, the index variable is only known within the for loop itself. Nerdy C++ programmers say that the scope of the variable is the for loop. In the example just given, the variable i is not accessible from the return statement because that state ment is not within the loop. Note, however, that not all compilers are strict about sticking to this rule. The Dev-C++ compiler (for example) generates a warning if you use i outside the for loop — but it uses the variable anyway.
Avoiding the dreaded infinite loop
An infinite loop is an execution path that continues forever. An infinite loop occurs any time the condition that would otherwise terminate the loop can’t occur — usually the result of a coding error.
Consider the following minor variation of the earlier loop:
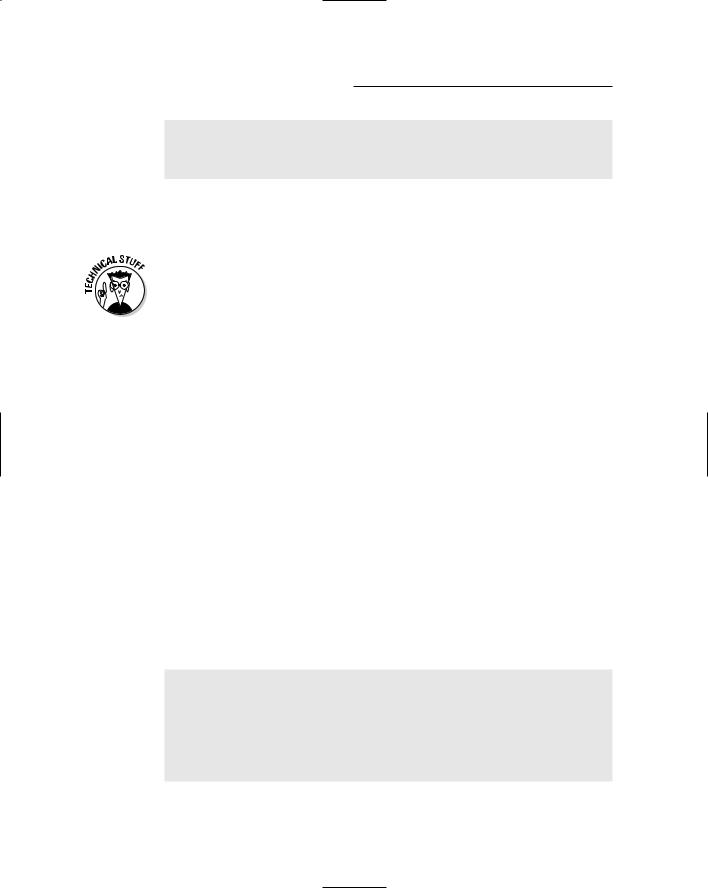
70 |
Part I: Introduction to C++ Programming |
while (loopCount > 0)
{
cout << “Only “ << loopCount << “ loops to go\n”;
}
The programmer forgot to decrement the variable loopCount as in the loop example below. The result is a loop counter that never changes. The test con dition is either always false or always true. The program executes in a neverending (infinite) loop.
I realize that nothing’s infinite. Eventually the power will fail, the computer will break, Microsoft will go bankrupt, and dogs will sleep with cats. . . . Either the loop will stop executing, or you won’t care anymore.
You can create an infinite loop in many more ways than shown here, most of which are much more difficult to spot than this one.
Applying special loop controls
C++ defines two special flow-control commands known as break and continue. Sometimes the condition for terminating the loop occurs at neither the beginning nor the end of the loop, but in the middle. Consider a program that accumulates numbers of values entered by the user. The loop terminates when the user enters a negative number.
The challenge with this problem is that the program can’t exit the loop until the user has entered a value, but must exit before the value is added to the sum.
For these cases, C++ defines the break command. When encountered, the break causes control to exit the current loop immediately. Control passes from the break statement to the statement immediately following the closed brace at the end of the loop.
The format of the break commands is as follows:
while(condition) // break works equally well in for loop
{
if (some other condition)
{
break; |
// exit the loop |
} |
|
} |
// control passes here when the |
|
// program encounters the break |
Armed with this new break command, my solution to the accumulator prob lem appears as the program BreakDemo.
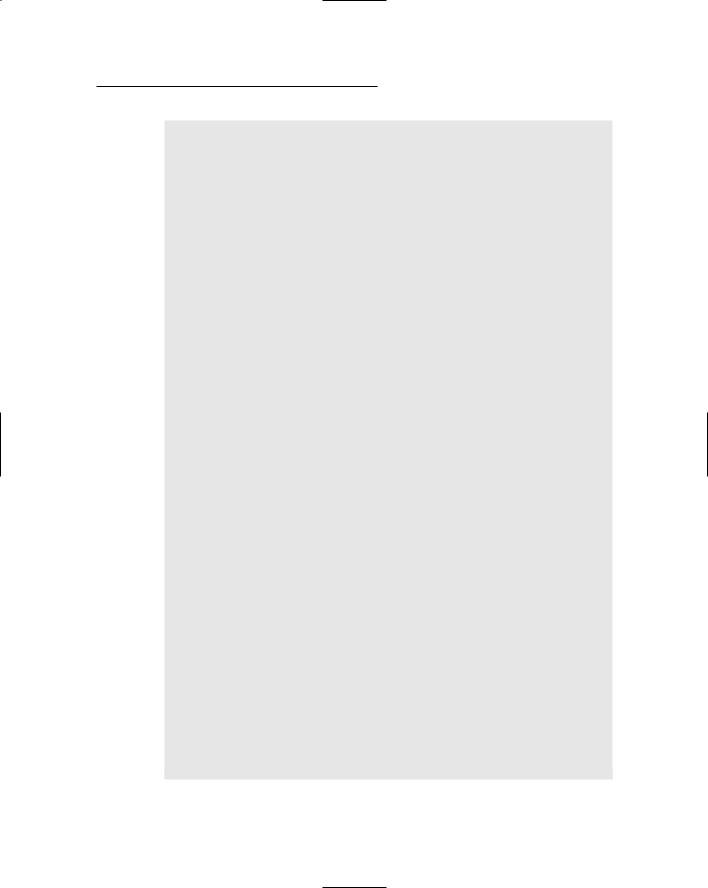
Chapter 5: Controlling Program Flow |
71 |
//BreakDemo - input a series of numbers.
//Continue to accumulate the sum
//of these numbers until the user
//enters a negative number. #include <cstdio>
#include <cstdlib> #include <iostream> using namespace std;
int main(int nNumberofArgs, char* pszArgs[])
{
// input the loop count int accumulator = 0;
cout << “This program sums values entered” << “by the user\n”;
cout << “Terminate the loop by entering “
<<“a negative number\n”;
//loop “forever”
for(;;)
{
//fetch another number int value = 0;
cout << “Enter next number: “; cin >> value;
//if it’s negative...
if (value < 0)
{
// ...then exit break;
}
//...otherwise add the number to the
//accumulator
accumulator = accumulator + value;
}
//now that we’ve exited the loop
//output the accumulated result cout << “\nThe total is “
<<accumulator
<<“\n”;
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
After explaining the rules to the user (entering a negative number to termi nate, and so on), the program enters what looks like an infinite for loop.
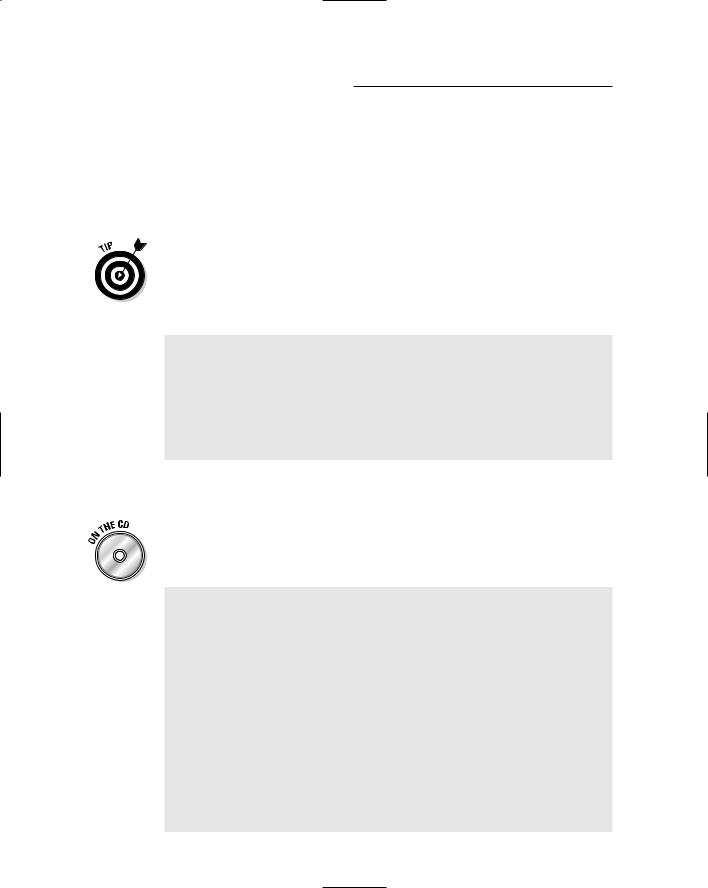
72 |
Part I: Introduction to C++ Programming |
Once within the loop, BreakDemo retrieves a number from the keyboard. Only after the program has read a number can it test to see whether the number it just read matches the exit criteria. If the input number is negative, control passes to the break, causing the program to exit the loop. If the input number is not negative, control skips over the break command to the expression that sums the new value into the accumulator. After the program exits the loop, it outputs the accumulated value and then exits.
When performing an operation on a variable repeatedly in a loop, make sure that the variable is initialized properly before entering the loop. In this case, the program zeros accumulator before entering the loop where value is added to it.
The result of an example run appears as follows:
This program sums values entered by the user
Terminate the loop by entering a negative number
Enter next number: 1
Enter next number: 2
Enter next number: 3
Enter next number: -1
The total is 6
Press any key to continue . . .
The continue command is used less frequently. When the program encoun ters the continue command, it immediately moves back to the top of the loop. The rest of the statements in the loop are ignored for the current iteration.
The following example snippet ignores negative numbers that the user might input. Only a zero terminates this version (the complete program appears on the CD-ROM as ContinueDemo):
while(true)// this while() has the same effect as for(;;)
{
// input a value
cout << “Input a value:”; cin >> value;
// if the value is negative...
if (value < 0)
{
// ...output an error message...
cout << “Negative numbers are not allowed\n”;
// ...and go back to the top of the loop continue;
}
// ...continue to process input like normal
}