
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
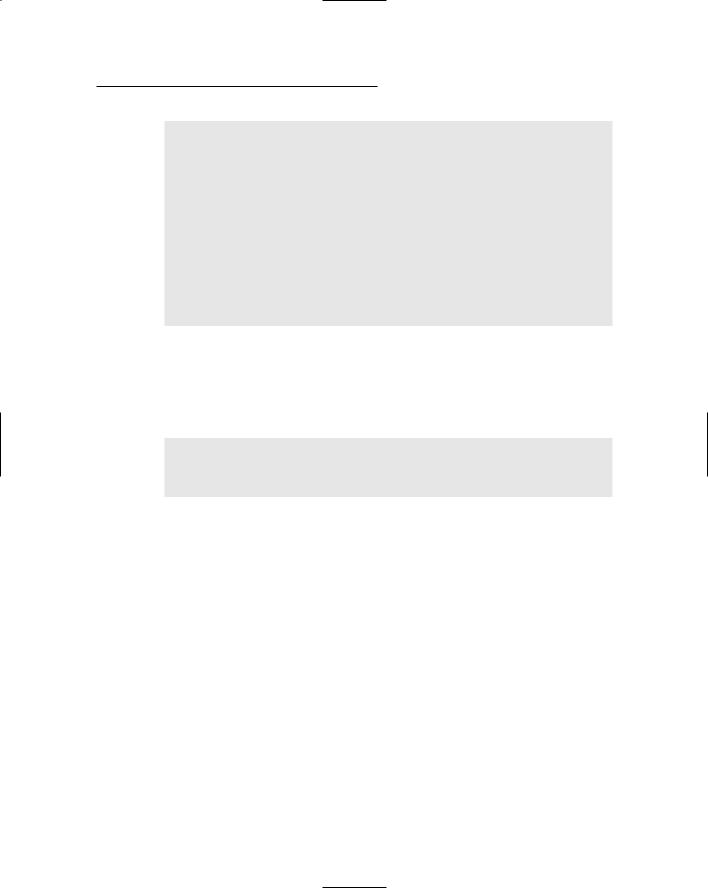
Chapter 5: Controlling Program Flow |
63 |
{
cout << “Argument 1 is greater than argument 2” << endl;
}
else
{
cout << “Argument 1 is not greater than argument 2” << endl;
}
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
Here the program reads two integers from the keyboard and compares them. If the expression “arg1 is greater than arg2” is true, control flows to the output statement cout << “Argument 1 is greater than argument 2”. If arg1 is not greater than arg2, control flows to the else clause where the statement cout << “Argument 1 is not greater than argument 2\n” is executed. Here’s what that operation looks like:
Enter arg1: 5
Enter arg2: 6
Argument 1 is not greater than argument 2
Press any key to continue . . .
Executing Loops in a Program
Branch statements allow you to control the flow of a program’s execution from one path of a program or another. This is a big improvement, but still not enough to write full-strength programs.
Consider the problem of updating the computer display. On the typical PC display, 1 million pixels are drawn to update the entire display. A program that can’t execute the same code repetitively would need to include the same set of instructions over and over 1,000 times.
What we really need is a way for the computer to execute the same sequence of instructions thousands and millions of times. Executing the same command multiple times requires some type of looping statements.
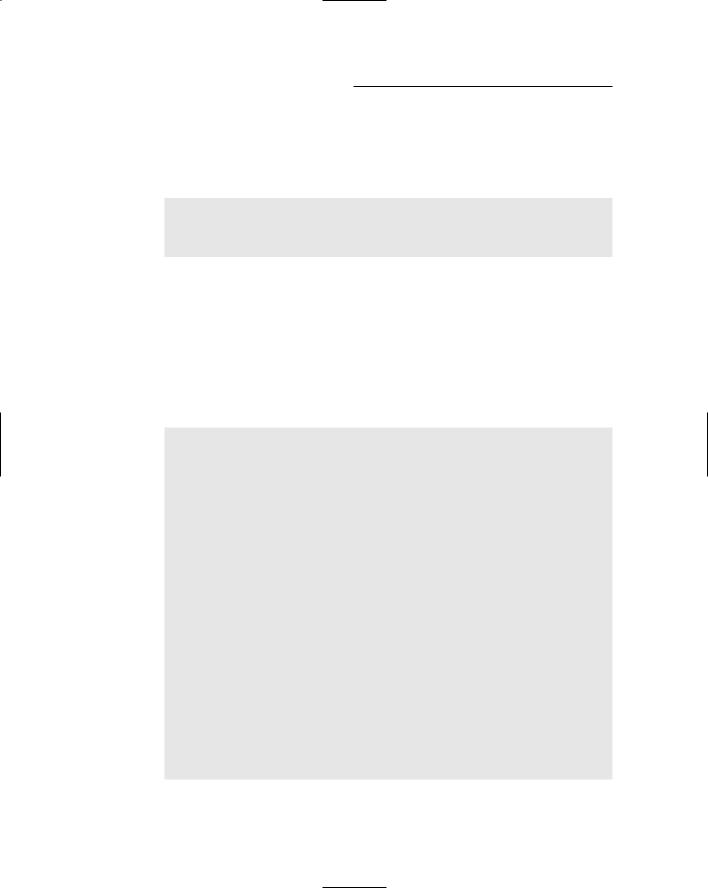
64 |
Part I: Introduction to C++ Programming |
Looping while a condition is true
The simplest form of looping statement is the while loop. Here’s what the while loop looks like:
while(condition)
{
// ... repeatedly executed as long as condition is true
}
The condition is tested. This condition could be if var > 10 or if var1 == var2 or anything else you might think of. If it is true, the state ments within the braces are executed. Upon encountering the closed brace, C++ returns control to the beginning, and the process starts over. The effect is that the C++ code within the braces is executed repeatedly as long as the condition is true. (Kind of reminds me of how I get to walk around the yard with my dog until she . . . well, until we’re done.)
If the condition were true the first time, what would make it be false in the future? Consider the following example program:
//WhileDemo - input a loop count. Loop while
//outputting astring arg number of times. #include <cstdio>
#include <cstdlib> #include <iostream> using namespace std;
int main(int nNumberofArgs, char* pszArgs[])
{
//input the loop count int loopCount;
cout << “Enter loopCount: “; cin >> loopCount;
//now loop that many times while (loopCount > 0)
{
loopCount = loopCount - 1;
cout << “Only “ << loopCount << “ loops to go\n”;
}
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
WhileDemo begins by retrieving a loop count from the user, which it stores in the variable loopCount. The program then executes a while loop. The while first tests loopCount. If loopCount is greater than zero, the program enters
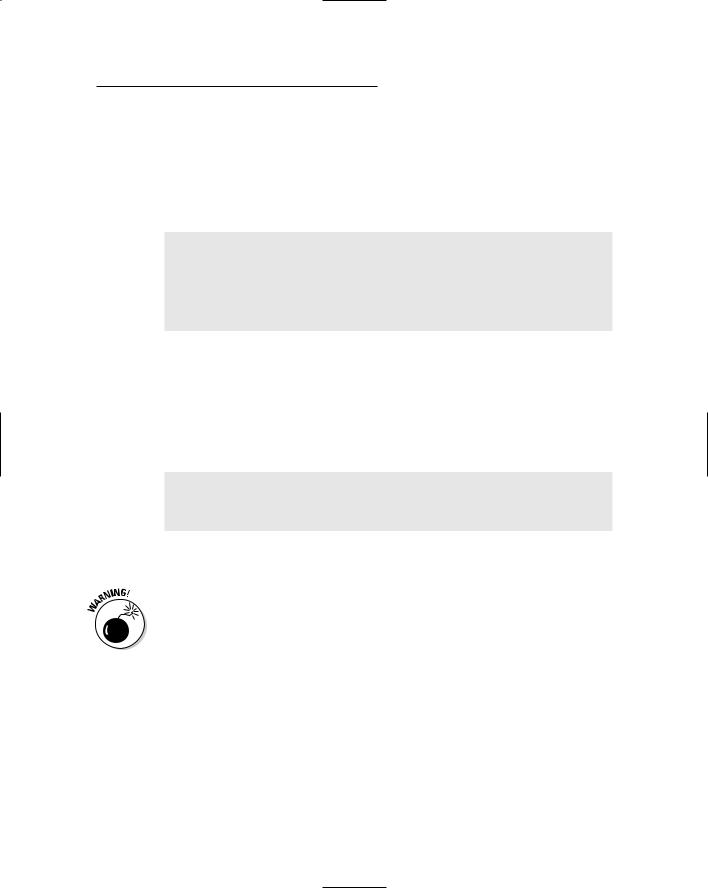
Chapter 5: Controlling Program Flow |
65 |
the body of the loop (the body is the code between the braces) where it decre ments loopCount by 1 and outputs the result to the display. The program then returns to the top of the loop to test whether loopCount is still positive.
When executed, the program WhileDemo outputs the results shown in this next snippet. Here I entered a loop count of 5. The result is that the program loops 5 times, each time outputting a countdown.
Enter loopCount: 5
Only 4 loops to go
Only 3 loops to go
Only 2 loops to go
Only 1 loops to go
Only 0 loops to go
Press any key to continue . . .
If the user enters a negative loop count, the program skips the loop entirely. That’s because the specified condition is never true, so control never enters the loop. In addition, if the user enters a very large number, the program loops for a long time before completing.
A separate, less frequently used version of the while loop known as the do . . . while appears identical except the condition isn’t tested until the bottom of the loop:
do
{
//...the inside of the loop
}while (condition);
Because the condition isn’t tested until the end, the body of the do . . .
while is always executed at least once.
The condition is only checked at the beginning of the while loop or at the end of the do . . . while loop. Even if the condition ceases to be true at some time during the execution of the loop, control does not exit the loop until the condition is retested.
Using the autoincrement/ autodecrement feature
Programmers very often use the autoincrement ++ or the autodecrement -- operators with loops that count something. Notice, from the following snip pet extracted from the WhileDemo example, that the program decrements the loop count by using assignment and subtraction statements, like this:
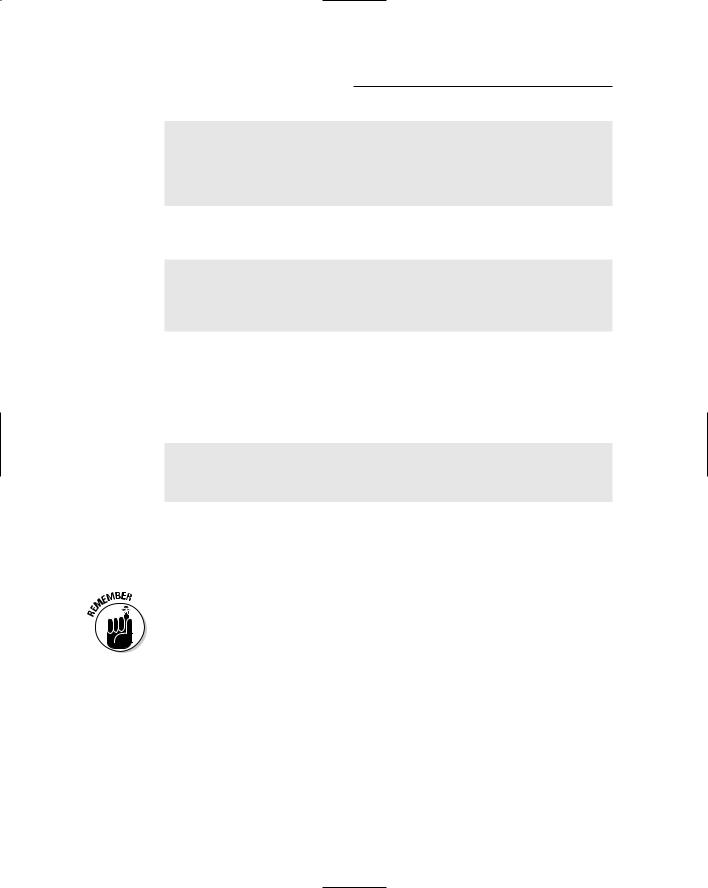
66 |
Part I: Introduction to C++ Programming |
// now loop that many times while (loopCount > 0)
{
loopCount = loopCount - 1;
cout << “Only “ << loopCount << “ loops to go\n”;
}
A more compact version uses the autodecrement feature, which does what you may well imagine:
while (loopCount > 0)
{
loopCount--;
cout << “Only “ << loopCount << “ loops to go\n”;
}
The logic in this version is the same as in the original. The only difference is the way that loopCount is decremented.
Because the autodecrement both decrements its argument and returns its value, the decrement operation can actually be combined with the while loop. In particular, the following version is the smallest loop yet.
while (loopCount-- > 0)
{
cout << “Only “ << loopCount << “ loops to go\n”;
}
Believe it or not, the loopcount— > 0 is the version that most C++ program mers would use. It’s not that C++ programmers like being cute (although they do). In fact, the more compact version (which embeds the autoincrement or autodecrement feature in the logical comparison) is easier to read, especially as you gain experience.
Both loopCount— and —loopCount expressions decrement loopCount. The former expression, however, returns the value of loopCount before being decremented; the latter expression does so after being decremented.
How often should the autodecrement version of WhileDemo execute when the user enters a loop count of 1? If you use the pre-decrement version, the value of —loopCount is 0, and the body of the loop is never entered. With the postdecrement version, the value of loopCount— is 1, and control enters the loop.
Beware thinking that the version of the program with the autodecrement command executes faster (since it contains fewer statements). It probably executes exactly the same. Modern compilers are pretty good at getting the number of machine-language instructions down to a minimum, no matter which of the decrement instructions shown here you actually use.