
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

Chapter 11: Examining Object-Oriented Programming 159
Preparing object-oriented nachos
In an object-oriented approach to making nachos, I would first identify the types of objects in the problem: chips, beans, cheese, and an oven. Then I would begin the task of modeling these objects in software, without regard to the details of how they will be used in the final program.
While I am doing this, I’m said to be working (and thinking) at the level of the basic objects. I need to think about making a useful oven, but I don’t have to think about the logical process of making nachos yet. After all, the microwave designers didn’t think about the specific problem of my making a snack. Rather, they set about the problem of designing and building a useful microwave.
After the objects I need have been successfully coded and tested, I can ratchet up to the next level of abstraction. I can start thinking at the nacho-making level, rather than the microwave-making level. At this point, I can pretty much translate my son’s instructions directly into C++ code.
Classifying Microwave Ovens
Critical to the concept of abstraction is that of classification. If I were to ask my son, “What’s a microwave?” he would probably say, “It’s an oven that . . .” If I then asked, “What’s an oven?” he might reply, “It’s a kitchen appliance that
. . .” (If I then asked, “What’s a kitchen appliance?” he would probably say, “Why are you asking so many stupid questions?”)
The answers my son gave to my questions stem from his understanding of our particular microwave as an example of the type of things called microwave
ovens. In addition, my son sees microwave ovens as just a special type of oven, which itself is just a special type of kitchen appliance.
In object-oriented computerese, my microwave is an instance of the class microwave. The class microwave is a subclass of the class oven, and the class oven is a subclass of the class kitchen appliances.
Humans classify. Everything about our world is ordered into taxonomies. We do this to reduce the number of things we have to remember. Take, for exam ple, the first time you saw an SUV. The advertisement probably called the SUV “revolutionary, the likes of which have never been seen.” But you and I know that that just isn’t so. I like the looks of some SUVs (others need to go back to take another crack at it), but, hey, an SUV is a car. As such, it shares all of (or at least most of) the properties of other cars. It has a steering wheel, seats, a motor, brakes, and so on. I bet I could even drive one without first reading the owner’s manual.

160 Part III: Introduction to Classes
I don’t have to clutter my limited storage with all the things that an SUV has in common with other cars. All I have to remember is “an SUV is a car that . . .” and tack on those few things that are unique to an SUV (like the price tag). I can go further. Cars are a subclass of wheeled vehicles along with other mem bers, such as trucks and pickups. Maybe wheeled vehicles are a subclass of vehicles, which includes boats and planes. And on and on and on.
Why Classify?
Why do we classify? It sounds like a lot of trouble. Besides, people have been using the functional approach for so long, why change now?
It may seem easier to design and build a microwave oven specifically for this one problem, rather than build a separate, more generic oven object. Suppose, for example, that I want to build a microwave to cook nachos and nachos only. I wouldn’t need to put a front panel on it, other than a START button. I always cook nachos the same amount of time, so I could dispense with all that DEFROST and TEMP COOK nonsense. My nachos-only microwave needs to hold only one flat little plate. Three cubic feet of space would be wasted on nachos.
For that matter, I can dispense with the concept of “microwave oven” alto gether. All I really need is the guts of the oven. Then, in the recipe, I put the instructions to make it work: “Put nachos in the box. Connect the red wire to the black wire. Bring the radar tube up to about 3,000 volts. Notice a slight hum. Try not to stand too close if you intend to have children.” Stuff like that.
But the functional approach has some problems:
Too complex. I don’t want the details of oven building mixed into the details of nacho building. If I can’t define the objects and pull them out of the morass of details to deal with separately, I must deal with all the complexities of the problem at the same time.
Not flexible. Someday I may need to replace the microwave oven with some other type of oven. I should be able to do so as long as its inter face is the same. Without being clearly delineated and developed sepa rately, it becomes impossible to cleanly remove an object type and replace it with another.
Not reusable. Ovens are used to make lots of different dishes. I don’t want to create a new oven every time I encounter a new recipe. Having solved a problem once, it would be nice to be able to reuse the solution in future programs.
The remaining chapters in this Part demonstrate how object-oriented lan guage features address these problems.
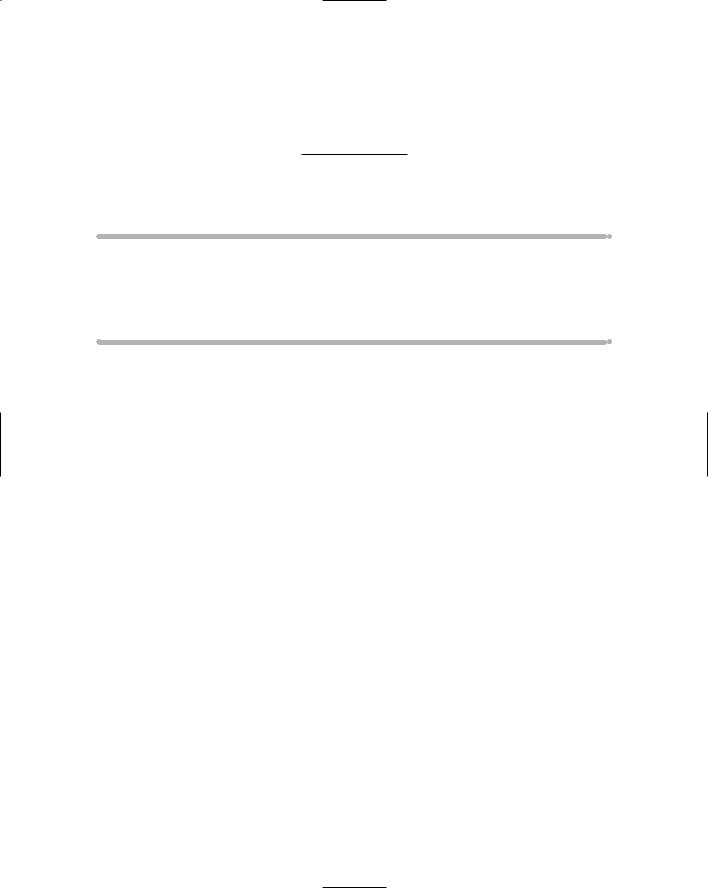
Chapter 12
Adding Class to C++
In This Chapter
Grouping data into classes
Declaring and defining class members
Accessing class members
Programs often deal with groups of data: a person’s name, rank, and serial number, stuff like that. Any one of these values is not sufficient to describe
a person — only in the aggregate do the values make any sense. A simple structure such as an array is great for holding stand-alone values; however, it doesn’t work very well for data groups. This makes good ol’ arrays inadequate for storing complex data (such as personal credit records that the Web compa nies maintain so they can lose them to hackers).
For reasons that will become clear shortly, I’ll call such a grouping of data an object. A microwave oven is an object (see Chapter 11 if that doesn’t make sense). You are an object. Your name, rank, and credit card number in a data base are objects.
Introducing the Class
What you need is a structure that can hold all the different types of data nec essary to describe a single object. In my simple example, a single object can hold both a first name and a last name along with a credit card number.
C++ calls the structure that combines multiples pieces of data into a single object a class.

162 Part III: Introduction to Classes
The Format of a Class
A class used to describe a name and credit card grouping might appear as follows:
//the dataset class class NameDataSet
{
public:
char firstName[128]; char lastName [128]; int creditCard;
};
//a single instance of a dataset NameDataSet nds;
A class definition starts with the keyword class followed by the name of the class and an open-close brace pair.
The statement after the open brace is the keyword public. (Hold off asking about the meaning of the public keyword. I’ll make its meaning public a little later. Later chapters describe options to public, such as private. Thus, the public must stay private until I can make the private public.)
The alternative keyword struct can be used. The keywords struct and class are identical except that the public declaration is assumed in the struct and can be omitted. You should stick with class for most programs for rea sons that will become clear later in this chapter.
Following the public keyword are the entries it takes to describe the object. The NameDataSet class contains the first and last name entries along with the credit card number. As you would expect, the first and last names are both character arrays — the credit card number is shown here as a simple integer (“the better to steal you with, my dear”).
A class declaration includes the data necessary to describe a single object.
The last line of the snippet declares the variable nds to be a single entry of class NameDataSet. Thus, nds might be an entry that describes a single person.
We say that nds is an instance of the class NameDataSet. You instantiate the class NameDataSet to create nds. Finally, we say that firstName and the others are members or properties of the class. We say a whole lot of silly things.