
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
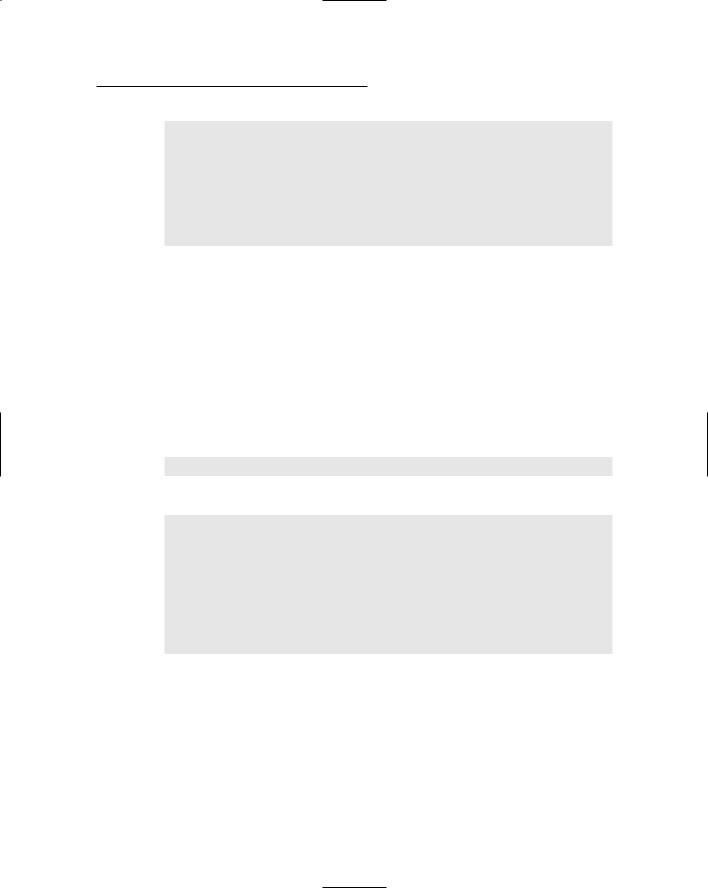
Chapter 14: Point and Stare at Objects 187
Student s;
Student* pS = &s; // create a pointer to s
//access the gpa member of the object pointed at by pS
//(this works as expected)
(*pS).gpa = 3.5;
return 0;
}
The *pS evaluates to the pointer’s Student object pointed at by pS. The
.gpa refers to the gpa member of that object.
Pointing toward arrow pointers
Using the splat operator together with parentheses works just fine for deref erencing pointers to objects; however, even the most hardened techies would admit that this mixing of asterisks and parentheses is a bit tortured.
C++ offers a more convenient operator for accessing members of an object to avoid clumsy object pointer expressions. The -> operator is defined as follows:
ps->gpa is equivalent to(*pS).gpa
This leads to the following:
int main(int argc, char* pArgs[])
{
Student s;
Student* pS = &s; // create a pointer to s
// access the gpa member of the object pointed at by pS pS->gpa = 3.5;
return 0;
}
The arrow operator is used almost exclusively because it is easier to read; however, the two forms are completely equivalent.
Passing Objects to Functions
Passing pointers to functions is just one of the ways to entertain yourself with pointer variables.

188 Part III: Introduction to Classes
Calling a function with an object value
As you know, C++ passes arguments to functions by reference when the argu ment type is flagged with the squiggly ‘&’ property (see Chapter 8). However, by default, C++ passes arguments to functions by value (you can check Chapter 6, on this one, if you insist).
Complex, user-defined class objects are passed the same as simple int values as shown in the following PassObjVal program:
//PassObjVal - attempts to change the value of an object
//in a function fail when the object is
//passed by value
#include <cstdio> #include <cstdlib> #include <iostream> using namespace std;
class Student
{
public:
int semesterHours; float gpa;
};
void someFn(Student copyS)
{
copyS.semesterHours = 10; copyS.gpa = 3.0;
cout << “The value of copyS.gpa = “ << copyS.gpa << “\n”;
}
int main(int argc, char* pArgs[])
{
Student s; s.gpa = 0.0;
//display the value of s.gpa before calling someFn() cout << “The value of s.gpa = “ << s.gpa << “\n”;
//pass the address of the existing object
cout << “Calling someFn(Student)\n”; someFn(s);
cout << “Returned from someFn(Student)\n”;
// the value of s.gpa remains 0
cout << “The value of s.gpa = “ << s.gpa << “\n”;
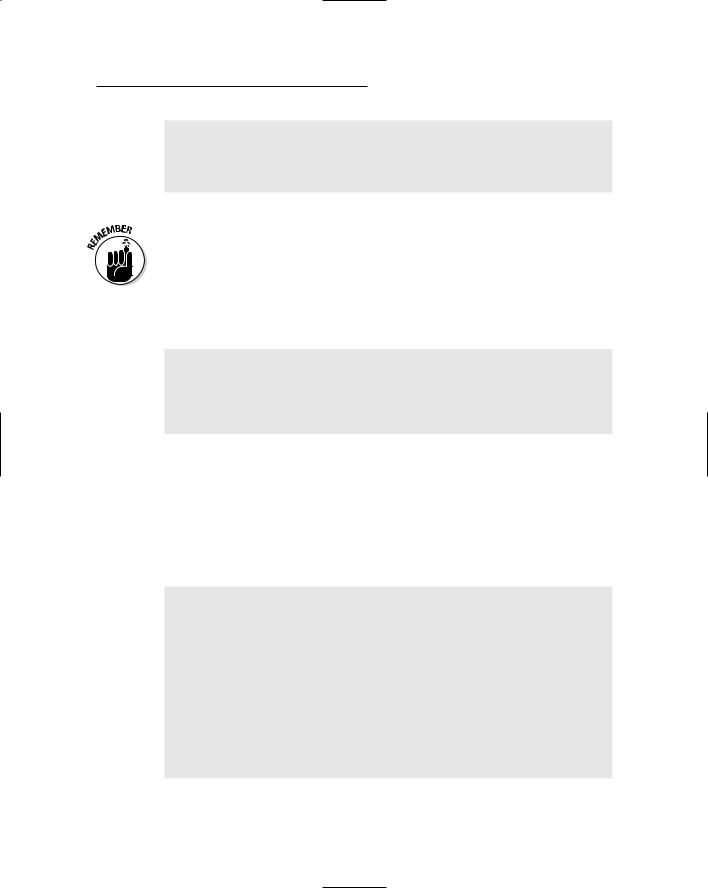
Chapter 14: Point and Stare at Objects 189
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
The function main() creates an object s and then passes s to the function someFn().
It is not the object s itself that is passed, but a copy of s.
The object copyS in someFn() begins life as an exact copy of the variable s in main(). Any change to copyS made within someFn() has no effect on s back in main(). Executing this program generates the following understandable but disappointing response:
The value of s.gpa = 0
Calling someFn(Student)
The value of copyS.gpa = 3
Returned from someFn(Student)
The value of s.gpa = 0
Press any key to continue . . .
Calling a function with an object pointer
Most of the time, the programmer wants any changes made in the function to be reflected in the calling function as well. For this, the C++ programmer must pass either the address of an object or a reference to the object rather than the object itself. The following PassObjPtr program uses the address approach.
//PassObjPtr - change the contents of an object in
//a function by passing a pointer to the #include <cstdio>
#include <cstdlib> #include <iostream> using namespace std;
class Student
{
public:
int semesterHours; float gpa;
};
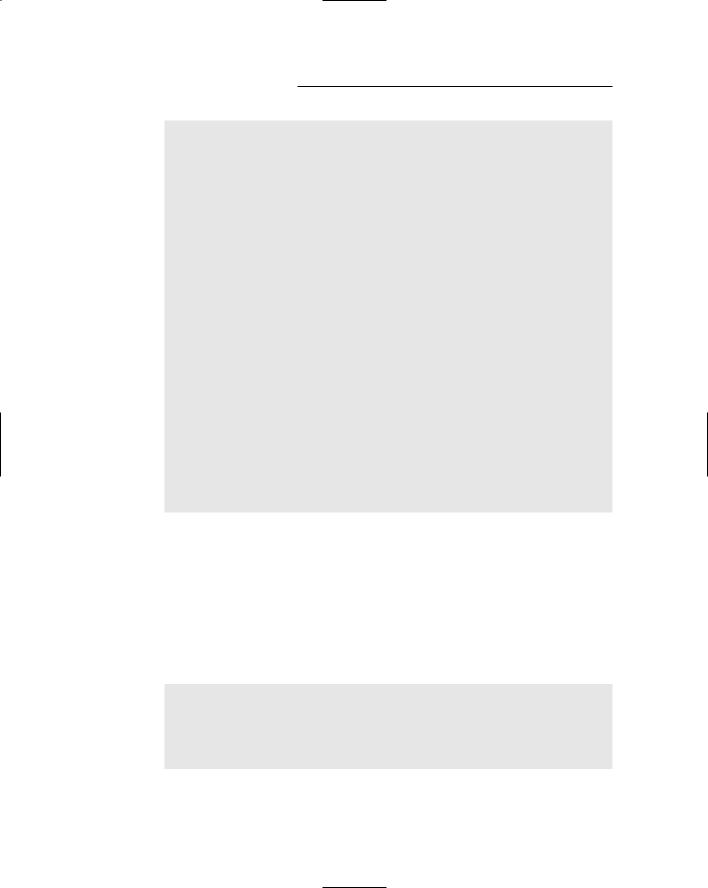
190 Part III: Introduction to Classes
void someFn(Student* pS)
{
pS->semesterHours = 10; pS->gpa = 3.0;
cout << “The value of pS->gpa = “ << pS->gpa << “\n”;
}
int main(int nNumberofArgs, char* pszArgs[])
{
Student s; s.gpa = 0.0;
//display the value of s.gpa before calling someFn() cout << “The value of s.gpa = “ << s.gpa << “\n”;
//pass the address of the existing object
cout << “Calling someFn(Student*)\n”; someFn(&s);
cout << “Returned from someFn(Student*)\n”;
// the value of s.gpa is now 3.0
cout << “The value of s.gpa = “ << s.gpa << “\n”;
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
The type of the argument to someFn() is a pointer to a Student object (oth erwise known as Student*). This is reflected in the way that the program calls someFn(), passing the address of s rather than the value of s. Giving someFn() the address of s allows him to modify whatever value that is stored there. Conceptually, this is akin to writing down the address of the house s on the piece of paper pS and then passing that paper to someFn(). The function someFn() uses the arrow syntax for dereferencing the pS pointer.
The output from PassObjPtr is much more satisfying (to me anyway):
The value of s.gpa = 0
Calling someFn(Student*)
The value of pS->gpa = 3
Returned from someFn(Student*)
The value of s.gpa = 3
Press any key to continue . . .