
- •contents
- •preface
- •acknowledgments
- •about this book
- •Special features
- •Best practices
- •Design patterns in action
- •Software directory
- •Roadmap
- •Part 1: JUnit distilled
- •Part 2: Testing strategies
- •Part 3: Testing components
- •Code
- •References
- •Author online
- •about the authors
- •about the title
- •about the cover illustration
- •JUnit jumpstart
- •1.1 Proving it works
- •1.2 Starting from scratch
- •1.3 Understanding unit testing frameworks
- •1.4 Setting up JUnit
- •1.5 Testing with JUnit
- •1.6 Summary
- •2.1 Exploring core JUnit
- •2.2 Launching tests with test runners
- •2.2.1 Selecting a test runner
- •2.2.2 Defining your own test runner
- •2.3 Composing tests with TestSuite
- •2.3.1 Running the automatic suite
- •2.3.2 Rolling your own test suite
- •2.4 Collecting parameters with TestResult
- •2.5 Observing results with TestListener
- •2.6 Working with TestCase
- •2.6.1 Managing resources with a fixture
- •2.6.2 Creating unit test methods
- •2.7 Stepping through TestCalculator
- •2.7.1 Creating a TestSuite
- •2.7.2 Creating a TestResult
- •2.7.3 Executing the test methods
- •2.7.4 Reviewing the full JUnit life cycle
- •2.8 Summary
- •3.1 Introducing the controller component
- •3.1.1 Designing the interfaces
- •3.1.2 Implementing the base classes
- •3.2 Let’s test it!
- •3.2.1 Testing the DefaultController
- •3.2.2 Adding a handler
- •3.2.3 Processing a request
- •3.2.4 Improving testProcessRequest
- •3.3 Testing exception-handling
- •3.3.1 Simulating exceptional conditions
- •3.3.2 Testing for exceptions
- •3.4 Setting up a project for testing
- •3.5 Summary
- •4.1 The need for unit tests
- •4.1.1 Allowing greater test coverage
- •4.1.2 Enabling teamwork
- •4.1.3 Preventing regression and limiting debugging
- •4.1.4 Enabling refactoring
- •4.1.5 Improving implementation design
- •4.1.6 Serving as developer documentation
- •4.1.7 Having fun
- •4.2 Different kinds of tests
- •4.2.1 The four flavors of software tests
- •4.2.2 The three flavors of unit tests
- •4.3 Determining how good tests are
- •4.3.1 Measuring test coverage
- •4.3.2 Generating test coverage reports
- •4.3.3 Testing interactions
- •4.4 Test-Driven Development
- •4.4.1 Tweaking the cycle
- •4.5 Testing in the development cycle
- •4.6 Summary
- •5.1 A day in the life
- •5.2 Running tests from Ant
- •5.2.1 Ant, indispensable Ant
- •5.2.2 Ant targets, projects, properties, and tasks
- •5.2.3 The javac task
- •5.2.4 The JUnit task
- •5.2.5 Putting Ant to the task
- •5.2.6 Pretty printing with JUnitReport
- •5.2.7 Automatically finding the tests to run
- •5.3 Running tests from Maven
- •5.3.2 Configuring Maven for a project
- •5.3.3 Executing JUnit tests with Maven
- •5.3.4 Handling dependent jars with Maven
- •5.4 Running tests from Eclipse
- •5.4.1 Creating an Eclipse project
- •5.4.2 Running JUnit tests in Eclipse
- •5.5 Summary
- •6.1 Introducing stubs
- •6.2 Practicing on an HTTP connection sample
- •6.2.1 Choosing a stubbing solution
- •6.2.2 Using Jetty as an embedded server
- •6.3 Stubbing the web server’s resources
- •6.3.1 Setting up the first stub test
- •6.3.2 Testing for failure conditions
- •6.3.3 Reviewing the first stub test
- •6.4 Stubbing the connection
- •6.4.1 Producing a custom URL protocol handler
- •6.4.2 Creating a JDK HttpURLConnection stub
- •6.4.3 Running the test
- •6.5 Summary
- •7.1 Introducing mock objects
- •7.2 Mock tasting: a simple example
- •7.3 Using mock objects as a refactoring technique
- •7.3.1 Easy refactoring
- •7.3.2 Allowing more flexible code
- •7.4 Practicing on an HTTP connection sample
- •7.4.1 Defining the mock object
- •7.4.2 Testing a sample method
- •7.4.3 Try #1: easy method refactoring technique
- •7.4.4 Try #2: refactoring by using a class factory
- •7.5 Using mocks as Trojan horses
- •7.6 Deciding when to use mock objects
- •7.7 Summary
- •8.1 The problem with unit-testing components
- •8.2 Testing components using mock objects
- •8.2.1 Testing the servlet sample using EasyMock
- •8.2.2 Pros and cons of using mock objects to test components
- •8.3 What are integration unit tests?
- •8.4 Introducing Cactus
- •8.5 Testing components using Cactus
- •8.5.1 Running Cactus tests
- •8.5.2 Executing the tests using Cactus/Jetty integration
- •8.6 How Cactus works
- •8.6.2 Stepping through a test
- •8.7 Summary
- •9.1 Presenting the Administration application
- •9.2 Writing servlet tests with Cactus
- •9.2.1 Designing the first test
- •9.2.2 Using Maven to run Cactus tests
- •9.2.3 Finishing the Cactus servlet tests
- •9.3 Testing servlets with mock objects
- •9.3.1 Writing a test using DynaMocks and DynaBeans
- •9.3.2 Finishing the DynaMock tests
- •9.4 Writing filter tests with Cactus
- •9.4.1 Testing the filter with a SELECT query
- •9.4.2 Testing the filter for other query types
- •9.4.3 Running the Cactus filter tests with Maven
- •9.5 When to use Cactus, and when to use mock objects
- •9.6 Summary
- •10.1 Revisiting the Administration application
- •10.2 What is JSP unit testing?
- •10.3 Unit-testing a JSP in isolation with Cactus
- •10.3.1 Executing a JSP with SQL results data
- •10.3.2 Writing the Cactus test
- •10.3.3 Executing Cactus JSP tests with Maven
- •10.4 Unit-testing taglibs with Cactus
- •10.4.1 Defining a custom tag
- •10.4.2 Testing the custom tag
- •10.5 Unit-testing taglibs with mock objects
- •10.5.1 Introducing MockMaker and installing its Eclipse plugin
- •10.5.2 Using MockMaker to generate mocks from classes
- •10.6 When to use mock objects and when to use Cactus
- •10.7 Summary
- •Unit-testing database applications
- •11.1 Introduction to unit-testing databases
- •11.2 Testing business logic in isolation from the database
- •11.2.1 Implementing a database access layer interface
- •11.2.2 Setting up a mock database interface layer
- •11.2.3 Mocking the database interface layer
- •11.3 Testing persistence code in isolation from the database
- •11.3.1 Testing the execute method
- •11.3.2 Using expectations to verify state
- •11.4 Writing database integration unit tests
- •11.4.1 Filling the requirements for database integration tests
- •11.4.2 Presetting database data
- •11.5 Running the Cactus test using Ant
- •11.5.1 Reviewing the project structure
- •11.5.2 Introducing the Cactus/Ant integration module
- •11.5.3 Creating the Ant build file step by step
- •11.5.4 Executing the Cactus tests
- •11.6 Tuning for build performance
- •11.6.2 Grouping tests in functional test suites
- •11.7.1 Choosing an approach
- •11.7.2 Applying continuous integration
- •11.8 Summary
- •Unit-testing EJBs
- •12.1 Defining a sample EJB application
- •12.2 Using a façade strategy
- •12.3 Unit-testing JNDI code using mock objects
- •12.4 Unit-testing session beans
- •12.4.1 Using the factory method strategy
- •12.4.2 Using the factory class strategy
- •12.4.3 Using the mock JNDI implementation strategy
- •12.5 Using mock objects to test message-driven beans
- •12.6 Using mock objects to test entity beans
- •12.7 Choosing the right mock-objects strategy
- •12.8 Using integration unit tests
- •12.9 Using JUnit and remote calls
- •12.9.1 Requirements for using JUnit directly
- •12.9.2 Packaging the Petstore application in an ear file
- •12.9.3 Performing automatic deployment and execution of tests
- •12.9.4 Writing a remote JUnit test for PetstoreEJB
- •12.9.5 Fixing JNDI names
- •12.9.6 Running the tests
- •12.10 Using Cactus
- •12.10.1 Writing an EJB unit test with Cactus
- •12.10.2 Project directory structure
- •12.10.3 Packaging the Cactus tests
- •12.10.4 Executing the Cactus tests
- •12.11 Summary
- •A.1 Getting the source code
- •A.2 Source code overview
- •A.3 External libraries
- •A.4 Jar versions
- •A.5 Directory structure conventions
- •B.1 Installing Eclipse
- •B.2 Setting up Eclipse projects from the sources
- •B.3 Running JUnit tests from Eclipse
- •B.4 Running Ant scripts from Eclipse
- •B.5 Running Cactus tests from Eclipse
- •references
- •index

Working with TestCase |
29 |
|
|
2.6.1Managing resources with a fixture
Some tests require resources that can be a bother to set up. A prime example is something like a database connection. Several tests in a TestCase may need a connection to a test database and access to a number of test tables. Another series of tests may require complex data structures or a long series of random inputs.
Putting common setup code in your tests doesn’t make sense. You are not testing your ability to create a resource—you just need a stable background environment in which to run tests. The set of background resources that you need to run a test is commonly called a test fixture.
DEFINITION fixture—The set of common resources or data that you need to run one or more tests.
A fixture is automatically created and destroyed by a TestCase through its setUp and tearDown methods. The TestCase calls setUp before running each of its tests and then calls tearDown when each test is complete. A key reason why you put more than one test method into the same TestCase is to share the fixture code. The TestCase life cycle is depicted in figure 2.6.
In practice, many developers now use mock objects or stubs to simulate database connections and other complex resources. For more about mock objects and stubs, see chapters 6 and 7, respectively.
A database connection is a good example of why you might need a fixture. If a TestCase includes several database tests, they each need a fresh connection to the database. A fixture makes it easy for you to open a new connection for each test without replicating code. For more about testing databases, see chapter 11. You can also use a fixture to generate input files; doing this means you do not have to carry your test files with your tests, and you always have a known state before the test is executed.
JUnit also reuses code through the utility methods provided by the Assert interface, as we’ll explain in the next section.
setUp() |
testXXX() |
tearDown() |
Figure 2.6 The TestCase life cycle re-creates your fixture, or scaffolding, for each test method.
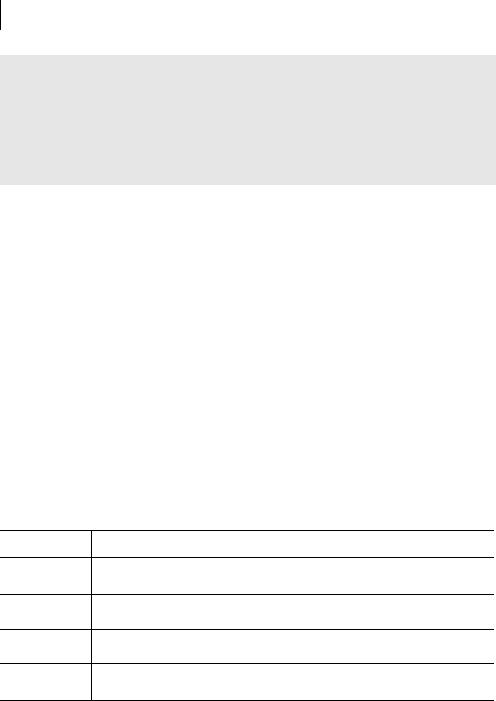
30CHAPTER 2
Exploring JUnit
JUnit design goals
When it is easy to reuse a fixture between tests, you can write tests more quickly. Each time you reuse the fixture, you decrease the initial investment made when the fixture was created. The TestCase fixtures speak to JUnit’s third design goal:
The framework must lower the cost of writing tests by reusing code.
2.6.2Creating unit test methods
Fixtures are a great way to reuse setup code. But there are many other common tasks that many tests perform over and over again. The JUnit framework encapsulates the most common testing tasks with an array of assert methods. The assert methods can make writing unit tests much easier.
The Assert supertype
In listing 2.2, we introduced the Test interface that both TestSuite and TestCase implement. The Test interface specifies only two methods, countTestCases and run. But in writing the TestCalculator case, you also used an assert method inherited from the base TestCase class.
The assert methods are defined in a utility class named (you guessed it) Assert. This class contains many of the nuts and bolts you use to construct tests.
If you look at the Javadoc or the source, you’ll see that, with 38 signatures, the Assert interface is longer than most. But if you look a little closer, you’ll notice that Assert has only eight public methods, as shown in table 2.3.
Table 2.3 The eight core methods provided by the Assert superclass
Method |
Description |
|
|
assertTrue Asserts that a condition is true. If it isn’t, the method throws an AssertionFailedError with the given message (if any).
assertFalse Asserts that a condition is true. If it isn’t, the method throws an AssertionFailedError with the given message (if any).
assertEquals Asserts that two objects are equal. If they are not, the method throws an AssertionFailedError with the given message (if any).
assertNotNull Asserts that an object isn’t null. If it is, the method throws an AssertionFailedError with the message (if any).
continued on next page

Working with TestCase |
31 |
|
|
Table 2.3 The eight core methods provided by the Assert superclass (continued)
Method |
Description |
|
|
assertNull Asserts that an object is null. If it isn’t, the method throws an AssertionFailedError with the given message (if any).
assertSame Asserts that two objects refer to the same object. If they do not, the method throws an AssertionFailedError with the given message (if any).
assertNotSame Asserts that two objects do not refer to the same object. If they do, the method throws an AssertionFailedError with the given message (if any).
fail |
Fails a test with the given message. |
The Javadoc is bulked up by convenience forms of these eight methods. The convenience forms make it easy to pass whatever type you need in your test. The assertEquals method, for example, has 20 forms! Most of the assertEquals forms are conveniences that end up calling the core assertEquals(String message, Object expected, Object actual) form.
The TestCase members
Along with the methods provided by Assert, TestCase implements 10 methods of its own. Table 2.4 overviews the 10 TestCase methods not provided by the Assert interface.
Table 2.4 The 10 additional methods provided by TestCase
Method |
Description |
|
|
countTestCases |
Counts the number of TestCases executed by run(TestResult result). (Spec- |
|
ified by the Test interface.) |
|
|
createResult |
Creates a default TestResult object. |
|
|
getName |
Gets the name of a TestCase. |
|
|
run |
Runs the TestCase and collects the results in TestResult. (Specified by the |
|
Test interface.) |
|
|
runBare |
Runs the test sequence without any special features, like automatic discovery of |
|
test methods. |
|
|
runTest |
Override to run the test and assert its state. |
|
|
setName |
Sets the name of a TestCase. |
|
|
setUp |
Initializes the fixture, for example, to open a network connection. This method is |
|
called before a test is executed. (Specified by the Test interface.) |
|
|
continued on next page
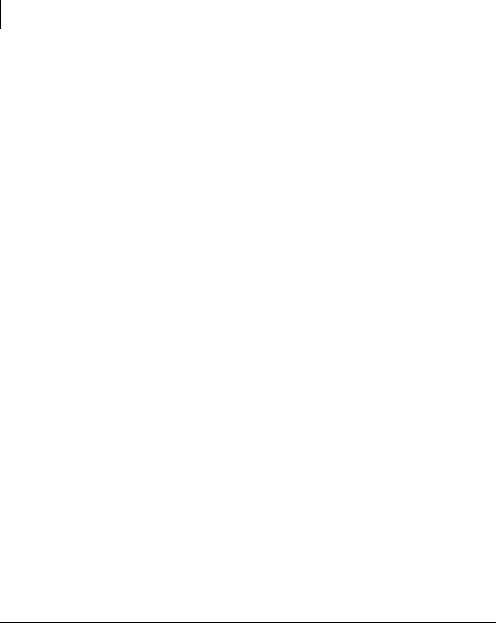
32CHAPTER 2
Exploring JUnit
Table 2.4 The 10 additional methods provided by TestCase (continued)
Method |
Description |
|
|
tearDown |
De-initializes the fixture, for example, to close a network connection. This method |
|
is called after a test is executed. (Specified by the Test interface.) |
|
|
toString |
Returns a string representation of the TestCase. |
|
|
In practice, many TestCases use the setUp and tearDown methods. The other methods in table 2.4 are mainly of interest to developers creating JUnit extensions. Used together, the 18 methods in tables 2.3 and 2.4 provide you with all the functionality you need to write tests with JUnit.
Keeping tests independent
As you begin to write your own tests, remember the first rule: Each unit test must run independently of all other unit tests. Unit tests must be able to be run in any order. One test must not depend on some side effect caused by a previous test (for example, a member variable being left in a certain state). If tests begin to depend on one another, you are inviting trouble. Here are some of the problems with codependent tests:
■Not portable—By default, JUnit finds test methods by reflection. The reflection API does not guarantee an order in which it returns the method names. If your tests depend on an ordering, then your suite may work in one Java Virtual Machine (JVM) but fail in another.
■Hard to maintain—When you modify one test, you may find that a number of other tests are affected. If you need to change the other tests, you spend time maintaining tests—time that could have been spent developing code.
■Not legible—To understand how co-dependent tests work, you must understand how each one works in turn. Tests become more difficult to read and harder to maintain. A good test must be easy to read and simple to maintain.
2.7Stepping through TestCalculator
Let’s demonstrate how all the core JUnit classes work together, using the TestCalculator test from listing 2.1 as an example. This is a very simple test class with a single test method:
import junit.framework.TestCase;
public class TestCalculator extends TestCase