
- •contents
- •preface
- •acknowledgments
- •about this book
- •Special features
- •Best practices
- •Design patterns in action
- •Software directory
- •Roadmap
- •Part 1: JUnit distilled
- •Part 2: Testing strategies
- •Part 3: Testing components
- •Code
- •References
- •Author online
- •about the authors
- •about the title
- •about the cover illustration
- •JUnit jumpstart
- •1.1 Proving it works
- •1.2 Starting from scratch
- •1.3 Understanding unit testing frameworks
- •1.4 Setting up JUnit
- •1.5 Testing with JUnit
- •1.6 Summary
- •2.1 Exploring core JUnit
- •2.2 Launching tests with test runners
- •2.2.1 Selecting a test runner
- •2.2.2 Defining your own test runner
- •2.3 Composing tests with TestSuite
- •2.3.1 Running the automatic suite
- •2.3.2 Rolling your own test suite
- •2.4 Collecting parameters with TestResult
- •2.5 Observing results with TestListener
- •2.6 Working with TestCase
- •2.6.1 Managing resources with a fixture
- •2.6.2 Creating unit test methods
- •2.7 Stepping through TestCalculator
- •2.7.1 Creating a TestSuite
- •2.7.2 Creating a TestResult
- •2.7.3 Executing the test methods
- •2.7.4 Reviewing the full JUnit life cycle
- •2.8 Summary
- •3.1 Introducing the controller component
- •3.1.1 Designing the interfaces
- •3.1.2 Implementing the base classes
- •3.2 Let’s test it!
- •3.2.1 Testing the DefaultController
- •3.2.2 Adding a handler
- •3.2.3 Processing a request
- •3.2.4 Improving testProcessRequest
- •3.3 Testing exception-handling
- •3.3.1 Simulating exceptional conditions
- •3.3.2 Testing for exceptions
- •3.4 Setting up a project for testing
- •3.5 Summary
- •4.1 The need for unit tests
- •4.1.1 Allowing greater test coverage
- •4.1.2 Enabling teamwork
- •4.1.3 Preventing regression and limiting debugging
- •4.1.4 Enabling refactoring
- •4.1.5 Improving implementation design
- •4.1.6 Serving as developer documentation
- •4.1.7 Having fun
- •4.2 Different kinds of tests
- •4.2.1 The four flavors of software tests
- •4.2.2 The three flavors of unit tests
- •4.3 Determining how good tests are
- •4.3.1 Measuring test coverage
- •4.3.2 Generating test coverage reports
- •4.3.3 Testing interactions
- •4.4 Test-Driven Development
- •4.4.1 Tweaking the cycle
- •4.5 Testing in the development cycle
- •4.6 Summary
- •5.1 A day in the life
- •5.2 Running tests from Ant
- •5.2.1 Ant, indispensable Ant
- •5.2.2 Ant targets, projects, properties, and tasks
- •5.2.3 The javac task
- •5.2.4 The JUnit task
- •5.2.5 Putting Ant to the task
- •5.2.6 Pretty printing with JUnitReport
- •5.2.7 Automatically finding the tests to run
- •5.3 Running tests from Maven
- •5.3.2 Configuring Maven for a project
- •5.3.3 Executing JUnit tests with Maven
- •5.3.4 Handling dependent jars with Maven
- •5.4 Running tests from Eclipse
- •5.4.1 Creating an Eclipse project
- •5.4.2 Running JUnit tests in Eclipse
- •5.5 Summary
- •6.1 Introducing stubs
- •6.2 Practicing on an HTTP connection sample
- •6.2.1 Choosing a stubbing solution
- •6.2.2 Using Jetty as an embedded server
- •6.3 Stubbing the web server’s resources
- •6.3.1 Setting up the first stub test
- •6.3.2 Testing for failure conditions
- •6.3.3 Reviewing the first stub test
- •6.4 Stubbing the connection
- •6.4.1 Producing a custom URL protocol handler
- •6.4.2 Creating a JDK HttpURLConnection stub
- •6.4.3 Running the test
- •6.5 Summary
- •7.1 Introducing mock objects
- •7.2 Mock tasting: a simple example
- •7.3 Using mock objects as a refactoring technique
- •7.3.1 Easy refactoring
- •7.3.2 Allowing more flexible code
- •7.4 Practicing on an HTTP connection sample
- •7.4.1 Defining the mock object
- •7.4.2 Testing a sample method
- •7.4.3 Try #1: easy method refactoring technique
- •7.4.4 Try #2: refactoring by using a class factory
- •7.5 Using mocks as Trojan horses
- •7.6 Deciding when to use mock objects
- •7.7 Summary
- •8.1 The problem with unit-testing components
- •8.2 Testing components using mock objects
- •8.2.1 Testing the servlet sample using EasyMock
- •8.2.2 Pros and cons of using mock objects to test components
- •8.3 What are integration unit tests?
- •8.4 Introducing Cactus
- •8.5 Testing components using Cactus
- •8.5.1 Running Cactus tests
- •8.5.2 Executing the tests using Cactus/Jetty integration
- •8.6 How Cactus works
- •8.6.2 Stepping through a test
- •8.7 Summary
- •9.1 Presenting the Administration application
- •9.2 Writing servlet tests with Cactus
- •9.2.1 Designing the first test
- •9.2.2 Using Maven to run Cactus tests
- •9.2.3 Finishing the Cactus servlet tests
- •9.3 Testing servlets with mock objects
- •9.3.1 Writing a test using DynaMocks and DynaBeans
- •9.3.2 Finishing the DynaMock tests
- •9.4 Writing filter tests with Cactus
- •9.4.1 Testing the filter with a SELECT query
- •9.4.2 Testing the filter for other query types
- •9.4.3 Running the Cactus filter tests with Maven
- •9.5 When to use Cactus, and when to use mock objects
- •9.6 Summary
- •10.1 Revisiting the Administration application
- •10.2 What is JSP unit testing?
- •10.3 Unit-testing a JSP in isolation with Cactus
- •10.3.1 Executing a JSP with SQL results data
- •10.3.2 Writing the Cactus test
- •10.3.3 Executing Cactus JSP tests with Maven
- •10.4 Unit-testing taglibs with Cactus
- •10.4.1 Defining a custom tag
- •10.4.2 Testing the custom tag
- •10.5 Unit-testing taglibs with mock objects
- •10.5.1 Introducing MockMaker and installing its Eclipse plugin
- •10.5.2 Using MockMaker to generate mocks from classes
- •10.6 When to use mock objects and when to use Cactus
- •10.7 Summary
- •Unit-testing database applications
- •11.1 Introduction to unit-testing databases
- •11.2 Testing business logic in isolation from the database
- •11.2.1 Implementing a database access layer interface
- •11.2.2 Setting up a mock database interface layer
- •11.2.3 Mocking the database interface layer
- •11.3 Testing persistence code in isolation from the database
- •11.3.1 Testing the execute method
- •11.3.2 Using expectations to verify state
- •11.4 Writing database integration unit tests
- •11.4.1 Filling the requirements for database integration tests
- •11.4.2 Presetting database data
- •11.5 Running the Cactus test using Ant
- •11.5.1 Reviewing the project structure
- •11.5.2 Introducing the Cactus/Ant integration module
- •11.5.3 Creating the Ant build file step by step
- •11.5.4 Executing the Cactus tests
- •11.6 Tuning for build performance
- •11.6.2 Grouping tests in functional test suites
- •11.7.1 Choosing an approach
- •11.7.2 Applying continuous integration
- •11.8 Summary
- •Unit-testing EJBs
- •12.1 Defining a sample EJB application
- •12.2 Using a façade strategy
- •12.3 Unit-testing JNDI code using mock objects
- •12.4 Unit-testing session beans
- •12.4.1 Using the factory method strategy
- •12.4.2 Using the factory class strategy
- •12.4.3 Using the mock JNDI implementation strategy
- •12.5 Using mock objects to test message-driven beans
- •12.6 Using mock objects to test entity beans
- •12.7 Choosing the right mock-objects strategy
- •12.8 Using integration unit tests
- •12.9 Using JUnit and remote calls
- •12.9.1 Requirements for using JUnit directly
- •12.9.2 Packaging the Petstore application in an ear file
- •12.9.3 Performing automatic deployment and execution of tests
- •12.9.4 Writing a remote JUnit test for PetstoreEJB
- •12.9.5 Fixing JNDI names
- •12.9.6 Running the tests
- •12.10 Using Cactus
- •12.10.1 Writing an EJB unit test with Cactus
- •12.10.2 Project directory structure
- •12.10.3 Packaging the Cactus tests
- •12.10.4 Executing the Cactus tests
- •12.11 Summary
- •A.1 Getting the source code
- •A.2 Source code overview
- •A.3 External libraries
- •A.4 Jar versions
- •A.5 Directory structure conventions
- •B.1 Installing Eclipse
- •B.2 Setting up Eclipse projects from the sources
- •B.3 Running JUnit tests from Eclipse
- •B.4 Running Ant scripts from Eclipse
- •B.5 Running Cactus tests from Eclipse
- •references
- •index
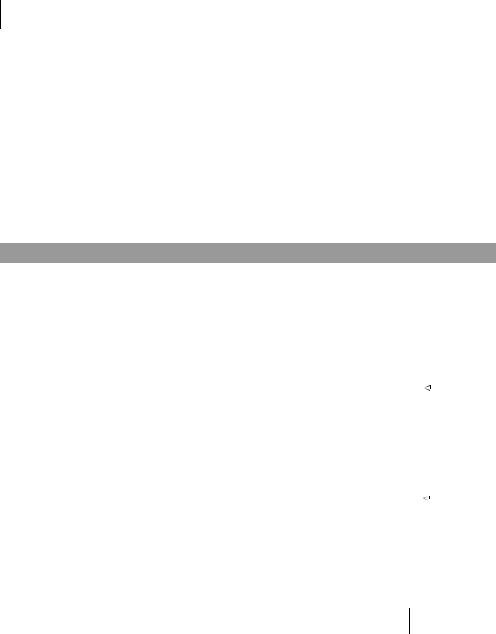
136CHAPTER 6
Coarse-grained testing with stubs
You have to use several inner classes (b and c) to be able to pass the StubHttpURLConnection class. You could also use anonymous inner classes for conciseness, but that approach would make the code more complex to read. Note that you haven’t written the StubHttpURLConnection class yet, which is the topic of the next section.
6.4.2Creating a JDK HttpURLConnection stub
The last step is to create a stub implementation of the HttpURLConnection class so you can return any value you want for the test. Listing 6.8 shows a simple implementation that returns the string It works as a stream to the caller.
Listing 6.8 Stubbed HttpURLConnection class
package junitbook.coarse.try2; |
|
|
|
|
|
|
|
import java.net.HttpURLConnection; |
|
|
|
|
|
|
|
import java.net.ProtocolException; |
HttpURLConnection |
|
|
|
|||
|
|||||||
import java.net.URL; |
|
has no interface; |
|
|
|
||
import java.io.InputStream; |
extend it and |
|
|
|
|||
import java.io.IOException; |
override its methods |
|
|
|
|||
import java.io.ByteArrayInputStream; |
|
|
|
|
|
|
|
public class StubHttpURLConnection extends HttpURLConnection |
|
|
|
|
|||
|
|
|
|
||||
{ |
|
|
|
|
|
|
|
private boolean isInput = true; |
|
|
|
|
|
|
|
protected StubHttpURLConnection(URL url) |
Override getInputStream |
|
|||||
|
|||||||
{ |
|
method to return a |
|
||||
super(url); |
|
|
|
string |
|
||
} |
|
|
|
|
|
|
|
public InputStream getInputStream() throws IOException |
|
|
|||||
|
|
||||||
{ |
|
|
|
|
|
|
|
if (!isInput) |
b Stub logic |
|
|
|
|
|
|
{ |
|
|
|
|
|
|
|
throw new ProtocolException( "Cannot read from URLConnection"
+ " if doInput=false (call setDoInput(true))");
}
ByteArrayInputStream bais = new ByteArrayInputStream( new String("It works").getBytes());
return bais;
}
Return expected string as a Stream
public void disconnect()
{
}

Stubbing the connection |
137 |
|
|
public void connect() throws IOException
{
}
public boolean usingProxy()
{
return false;
}
}
HttpURLConnection does not provide an interface, so you have to extend it and override the methods you want to stub. In this stub, you provide an implementation for the getInputStream method because it is the only method used by your code under test. Should the code to test use more APIs from HttpURLConnection, you would also need to stub these additional methods. This is where the code would become more complex—you would need to completely reproduce the same behavior as the real HttpURLConnection. For example, at b, you test that if setDoInput(false) has been called in the code under test, then a call to the getInputStream method returns a ProtocolException. (This is the behavior of HttpURLConnection.) Fortunately, in most cases, you only need to stub a few methods and not the whole API.
6.4.3Running the test
Let’s run the TestWebClient test, which uses the StubHttpURLConnection. Figure 6.5 shows the result.
As you can see, it is much easier to stub the connection than to stub the web resource. This approach does not bring the same level of testing (you are not
Figure 6.5
Result of executing
TestWebClient (which uses the
StubHttpURLConnection)
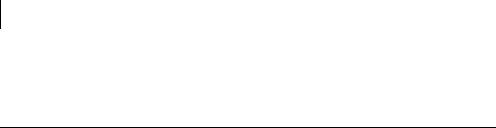
138CHAPTER 6
Coarse-grained testing with stubs
performing integration tests), but it enables you to more easily write a focused unit test for the WebClient code logic.
6.5 Summary
In this chapter, we have demonstrated how using a stub has helped us unit-test code accessing a remote web server using the JDK’s HttpURLConnection API. In particular, we have shown how to stub the remote web server by using Jetty. Jetty’s embeddable nature lets you concentrate on stubbing only the Jetty HTTP request handler, instead of having to stub the whole container. We also demonstrated a more lightweight stub by writing it for the JDK’s HttpURLConnection implementation.
The next chapter will demonstrate a technique called mock objects that allows fine-grained unit testing, is completely generic, and (best of all) forces you to write good code. Although stubs are very useful in some cases, they’re more a vestige of the past, when the general consensus was that tests should be a separate activity and should not modify the code. The new mock-objects strategy not only allows modification of code, it favors it. Using mock objects is a unit-testing strategy, but it is more than that: It is a completely new way of writing code.

Testing 7in isolation with mock objects
This chapter covers
■Introducing and demonstrating mock objects
■Performing different refactorings
■Using mock objects to verify API contracts on collaborating classes
■Practicing on an HTTP connection sample application
139