
- •contents
- •preface
- •acknowledgments
- •about this book
- •Special features
- •Best practices
- •Design patterns in action
- •Software directory
- •Roadmap
- •Part 1: JUnit distilled
- •Part 2: Testing strategies
- •Part 3: Testing components
- •Code
- •References
- •Author online
- •about the authors
- •about the title
- •about the cover illustration
- •JUnit jumpstart
- •1.1 Proving it works
- •1.2 Starting from scratch
- •1.3 Understanding unit testing frameworks
- •1.4 Setting up JUnit
- •1.5 Testing with JUnit
- •1.6 Summary
- •2.1 Exploring core JUnit
- •2.2 Launching tests with test runners
- •2.2.1 Selecting a test runner
- •2.2.2 Defining your own test runner
- •2.3 Composing tests with TestSuite
- •2.3.1 Running the automatic suite
- •2.3.2 Rolling your own test suite
- •2.4 Collecting parameters with TestResult
- •2.5 Observing results with TestListener
- •2.6 Working with TestCase
- •2.6.1 Managing resources with a fixture
- •2.6.2 Creating unit test methods
- •2.7 Stepping through TestCalculator
- •2.7.1 Creating a TestSuite
- •2.7.2 Creating a TestResult
- •2.7.3 Executing the test methods
- •2.7.4 Reviewing the full JUnit life cycle
- •2.8 Summary
- •3.1 Introducing the controller component
- •3.1.1 Designing the interfaces
- •3.1.2 Implementing the base classes
- •3.2 Let’s test it!
- •3.2.1 Testing the DefaultController
- •3.2.2 Adding a handler
- •3.2.3 Processing a request
- •3.2.4 Improving testProcessRequest
- •3.3 Testing exception-handling
- •3.3.1 Simulating exceptional conditions
- •3.3.2 Testing for exceptions
- •3.4 Setting up a project for testing
- •3.5 Summary
- •4.1 The need for unit tests
- •4.1.1 Allowing greater test coverage
- •4.1.2 Enabling teamwork
- •4.1.3 Preventing regression and limiting debugging
- •4.1.4 Enabling refactoring
- •4.1.5 Improving implementation design
- •4.1.6 Serving as developer documentation
- •4.1.7 Having fun
- •4.2 Different kinds of tests
- •4.2.1 The four flavors of software tests
- •4.2.2 The three flavors of unit tests
- •4.3 Determining how good tests are
- •4.3.1 Measuring test coverage
- •4.3.2 Generating test coverage reports
- •4.3.3 Testing interactions
- •4.4 Test-Driven Development
- •4.4.1 Tweaking the cycle
- •4.5 Testing in the development cycle
- •4.6 Summary
- •5.1 A day in the life
- •5.2 Running tests from Ant
- •5.2.1 Ant, indispensable Ant
- •5.2.2 Ant targets, projects, properties, and tasks
- •5.2.3 The javac task
- •5.2.4 The JUnit task
- •5.2.5 Putting Ant to the task
- •5.2.6 Pretty printing with JUnitReport
- •5.2.7 Automatically finding the tests to run
- •5.3 Running tests from Maven
- •5.3.2 Configuring Maven for a project
- •5.3.3 Executing JUnit tests with Maven
- •5.3.4 Handling dependent jars with Maven
- •5.4 Running tests from Eclipse
- •5.4.1 Creating an Eclipse project
- •5.4.2 Running JUnit tests in Eclipse
- •5.5 Summary
- •6.1 Introducing stubs
- •6.2 Practicing on an HTTP connection sample
- •6.2.1 Choosing a stubbing solution
- •6.2.2 Using Jetty as an embedded server
- •6.3 Stubbing the web server’s resources
- •6.3.1 Setting up the first stub test
- •6.3.2 Testing for failure conditions
- •6.3.3 Reviewing the first stub test
- •6.4 Stubbing the connection
- •6.4.1 Producing a custom URL protocol handler
- •6.4.2 Creating a JDK HttpURLConnection stub
- •6.4.3 Running the test
- •6.5 Summary
- •7.1 Introducing mock objects
- •7.2 Mock tasting: a simple example
- •7.3 Using mock objects as a refactoring technique
- •7.3.1 Easy refactoring
- •7.3.2 Allowing more flexible code
- •7.4 Practicing on an HTTP connection sample
- •7.4.1 Defining the mock object
- •7.4.2 Testing a sample method
- •7.4.3 Try #1: easy method refactoring technique
- •7.4.4 Try #2: refactoring by using a class factory
- •7.5 Using mocks as Trojan horses
- •7.6 Deciding when to use mock objects
- •7.7 Summary
- •8.1 The problem with unit-testing components
- •8.2 Testing components using mock objects
- •8.2.1 Testing the servlet sample using EasyMock
- •8.2.2 Pros and cons of using mock objects to test components
- •8.3 What are integration unit tests?
- •8.4 Introducing Cactus
- •8.5 Testing components using Cactus
- •8.5.1 Running Cactus tests
- •8.5.2 Executing the tests using Cactus/Jetty integration
- •8.6 How Cactus works
- •8.6.2 Stepping through a test
- •8.7 Summary
- •9.1 Presenting the Administration application
- •9.2 Writing servlet tests with Cactus
- •9.2.1 Designing the first test
- •9.2.2 Using Maven to run Cactus tests
- •9.2.3 Finishing the Cactus servlet tests
- •9.3 Testing servlets with mock objects
- •9.3.1 Writing a test using DynaMocks and DynaBeans
- •9.3.2 Finishing the DynaMock tests
- •9.4 Writing filter tests with Cactus
- •9.4.1 Testing the filter with a SELECT query
- •9.4.2 Testing the filter for other query types
- •9.4.3 Running the Cactus filter tests with Maven
- •9.5 When to use Cactus, and when to use mock objects
- •9.6 Summary
- •10.1 Revisiting the Administration application
- •10.2 What is JSP unit testing?
- •10.3 Unit-testing a JSP in isolation with Cactus
- •10.3.1 Executing a JSP with SQL results data
- •10.3.2 Writing the Cactus test
- •10.3.3 Executing Cactus JSP tests with Maven
- •10.4 Unit-testing taglibs with Cactus
- •10.4.1 Defining a custom tag
- •10.4.2 Testing the custom tag
- •10.5 Unit-testing taglibs with mock objects
- •10.5.1 Introducing MockMaker and installing its Eclipse plugin
- •10.5.2 Using MockMaker to generate mocks from classes
- •10.6 When to use mock objects and when to use Cactus
- •10.7 Summary
- •Unit-testing database applications
- •11.1 Introduction to unit-testing databases
- •11.2 Testing business logic in isolation from the database
- •11.2.1 Implementing a database access layer interface
- •11.2.2 Setting up a mock database interface layer
- •11.2.3 Mocking the database interface layer
- •11.3 Testing persistence code in isolation from the database
- •11.3.1 Testing the execute method
- •11.3.2 Using expectations to verify state
- •11.4 Writing database integration unit tests
- •11.4.1 Filling the requirements for database integration tests
- •11.4.2 Presetting database data
- •11.5 Running the Cactus test using Ant
- •11.5.1 Reviewing the project structure
- •11.5.2 Introducing the Cactus/Ant integration module
- •11.5.3 Creating the Ant build file step by step
- •11.5.4 Executing the Cactus tests
- •11.6 Tuning for build performance
- •11.6.2 Grouping tests in functional test suites
- •11.7.1 Choosing an approach
- •11.7.2 Applying continuous integration
- •11.8 Summary
- •Unit-testing EJBs
- •12.1 Defining a sample EJB application
- •12.2 Using a façade strategy
- •12.3 Unit-testing JNDI code using mock objects
- •12.4 Unit-testing session beans
- •12.4.1 Using the factory method strategy
- •12.4.2 Using the factory class strategy
- •12.4.3 Using the mock JNDI implementation strategy
- •12.5 Using mock objects to test message-driven beans
- •12.6 Using mock objects to test entity beans
- •12.7 Choosing the right mock-objects strategy
- •12.8 Using integration unit tests
- •12.9 Using JUnit and remote calls
- •12.9.1 Requirements for using JUnit directly
- •12.9.2 Packaging the Petstore application in an ear file
- •12.9.3 Performing automatic deployment and execution of tests
- •12.9.4 Writing a remote JUnit test for PetstoreEJB
- •12.9.5 Fixing JNDI names
- •12.9.6 Running the tests
- •12.10 Using Cactus
- •12.10.1 Writing an EJB unit test with Cactus
- •12.10.2 Project directory structure
- •12.10.3 Packaging the Cactus tests
- •12.10.4 Executing the Cactus tests
- •12.11 Summary
- •A.1 Getting the source code
- •A.2 Source code overview
- •A.3 External libraries
- •A.4 Jar versions
- •A.5 Directory structure conventions
- •B.1 Installing Eclipse
- •B.2 Setting up Eclipse projects from the sources
- •B.3 Running JUnit tests from Eclipse
- •B.4 Running Ant scripts from Eclipse
- •B.5 Running Cactus tests from Eclipse
- •references
- •index
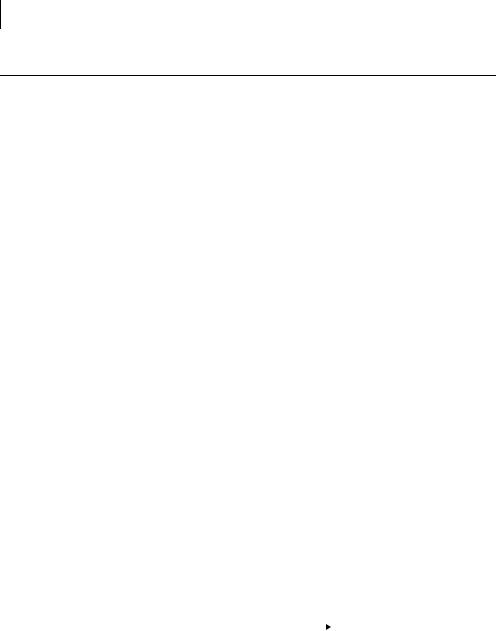
150CHAPTER 7
Testing in isolation with mock objects
7.4Practicing on an HTTP connection sample
To see how mock objects work in a practical example, let’s use a simple application that opens an HTTP connection to a remote server and reads the content of a page. In chapter 6 we tested that application using stubs. Let’s now unit-test it using a mock-object approach to simulate the HTTP connection.
In addition, you’ll learn how to write mocks for classes that do not have a Java interface (namely, the HttpURLConnection class). We will show a full scenario in which you start with an initial testing implementation, improve the implementation as you go, and modify the original code to make it more flexible. We will also show how to test for error conditions using mocks.
As you dive in, you will keep improving both the test code and the sample application, exactly as you might if you were writing the unit tests for the same application. In the process, you will learn how to reach a simple and elegant testing solution while making your application code more flexible and capable of handling change.
Figure 7.2 introduces the sample HTTP application, which consists of a simple WebClient.getContent method performing an HTTP connection to a web resource executing on a web server. You want to be able to unit-test the getContent method in isolation from the web resource.
7.4.1Defining the mock object
Figure 7.3 illustrates the definition of a mock object. The MockURL class stands in for the real URL class, and all calls to the URL class in getContent are directed to the MockURL class. As you can see, the test is the controller: It creates and configures the behavior the mock must have for this test, it (somehow) replaces the real URL class with the MockURL class, and it runs the test.
WebClient |
|
|
Web Server |
|
||
|
|
|
|
|
|
|
public String getContent(URL url) |
|
|
|
Web Resource |
|
|
{ |
|
|
|
|
|
|
[...] |
|
HTTP |
|
|
public void doGet(...) |
|
url.openConnection(); |
|
|
|
|
{ |
|
|
|
|
|
|
||
[...] |
|
connection |
|
|
//Generate HTML |
|
} |
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Figure 7.2 The sample HTTP application before introducing the test

|
|
|
|
|
Practicing on an HTTP connection sample |
151 |
|||||||
WebClient (being tested) |
|
|
|
Mock |
|
|
|
|
|||||
|
|
|
|
|
|
|
|||||||
|
|
|
|
|
|
|
|
||||||
public String getContent(URL url) |
|
|
|
public MockURL |
|
|
|||||||
{ |
|
|
|
|
|
|
{ |
public void openConnection() |
|
|
|||
[...] |
|
|
|
method |
|
{ |
|
|
|||||
} |
|
|
|
|
|
|
{ |
// logic controlled by |
|
|
|||
mockUrl.openConnection(); |
|
|
|
|
|
|
|
||||||
[...] |
|
|
|
call |
|
|
|
} |
// test case |
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
c swap in mock |
d |
|
run test |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
public TestWebClient extends TestCase |
|
|
|
|
|
|
|
|
|
||||
{ |
|
|
|
|
|
|
|
|
|
|
|
|
|
[...] |
|
|
|
|
|
create and configure the |
|
|
|
||||
public void testGetConnect() |
|
|
|
|
|||||||||
|
|
Mock behavior |
|
|
|
|
|||||||
{ |
|
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
// b, c and d |
|
|
|
|
|
b |
|
|
|
|
|||
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Test Case |
|
|
|
|
|
|
|
|
|
|
|
|
|
Figure 7.3 The steps involved in a test using mock objects
Figure 7.3 shows an interesting aspect of the mock-objects strategy: the need to be able to swap-in the mock in the production code. The perceptive reader will have noticed that because the URL class is final, it is actually not possible to create a MockURL class that extends it. In the coming sections, we will demonstrate how this feat can be performed in a different way (by mocking at another level). In any case, when using the mock-objects strategy, swapping-in the mock instead of the real class is the hard part. This may be viewed as a negative point for mock objects, because you usually need to modify your code to provide a trapdoor. Ironically, modifying code to encourage flexibility is one of the strongest advantages of using mocks, as explained in section 7.3.1.
7.4.2Testing a sample method
The example in listing 7.6 demonstrates a code snippet that opens an HTTP connection to a given URL and reads the content found at that URL. Let’s imagine that it’s one method of a bigger application that you want to unit-test, and let’s unit-test that method.
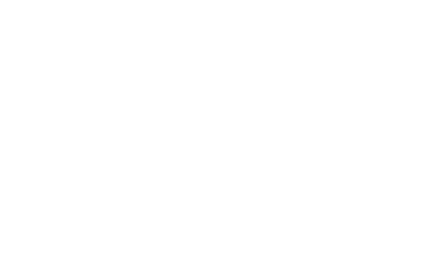
152 |
CHAPTER 7 |
|
|
|
|
|
Testing in isolation with mock objects |
|
|
|
|
|
|
|
|
|
|
|
Listing 7.6 Sample method that opens an HTTP connection |
|
|
|
|
|
package junitbook.fine.try1; |
|
|
|
|
|
import java.net.URL; |
|
|
|
|
|
import java.net.HttpURLConnection; |
|
|
|
|
|
import java.io.InputStream; |
|
|
|
|
|
import java.io.IOException; |
|
|
|
|
|
public class WebClient |
|
|
|
|
|
{ |
|
|
|
|
|
public String getContent(URL url) |
|
|
|
|
|
{ |
|
|
|
|
|
StringBuffer content = new StringBuffer(); |
|
|
|
|
|
try |
|
|
|
|
|
{ |
|
|
|
|
|
HttpURLConnection connection = |
|
b |
||
|
|
||||
|
(HttpURLConnection) url.openConnection(); |
|
|
|
|
|
connection.setDoInput(true); |
c |
|||
|
InputStream is = connection.getInputStream(); |
||||
|
byte[] buffer = new byte[2048]; |
|
|
d |
|
|
|
|
|||
|
int count; |
|
|
||
|
|
|
|
|
|
|
while (-1 != (count = is.read(buffer))) |
|
|
|
|
|
{ |
|
|
|
|
|
content.append(new String(buffer, 0, count)); |
|
|||
|
} |
|
|
|
|
|
} |
|
|
|
|
|
catch (IOException e) |
|
|
|
|
|
{ |
|
|
|
|
|
return null; |
|
|
|
|
|
} |
|
|
|
|
|
return content.toString(); |
|
|
|
|
|
} |
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
b c Open an HTTP connection using the HttpURLConnection class. |
|
|
|
|
dRead the content until there is nothing more to read.
If an error occurs, you return null. Admittedly, this is not the best possible errorhandling solution, but it is good enough for the moment. (And your tests will give you the courage to refactor later.)
7.4.3Try #1: easy method refactoring technique
The idea is to be able to test the getContent method independently of a real HTTP connection to a web server. If you map the knowledge you acquired in section 7.2, it means writing a mock URL in which the url.openConnection method returns a mock HttpURLConnection. The MockHttpURLConnection class would provide an
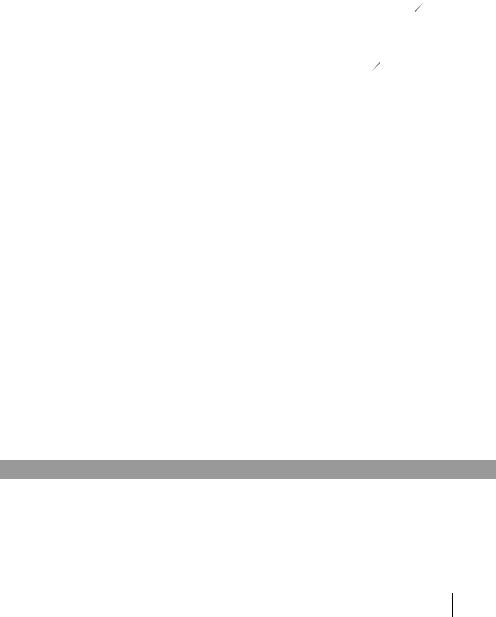
Practicing on an HTTP connection sample |
153 |
|
|
implementation that lets the test decides what the getInputStream method returns. Ideally, you would be able to write the following test:
public void testGetContentOk() throws Exception
{
MockHttpURLConnection mockConnection = |
|
|
b |
|
new MockHttpURLConnection(); |
|
|
|
|
|
|
|
|
|
mockConnection.setupGetInputStream( |
|
|
|
|
new ByteArrayInputStream("It works".getBytes())); |
|
|||
MockURL mockURL = new MockURL(); |
|
|
c |
|
|
|
|||
mockURL.setupOpenConnection(mockConnection); |
|
|
|
|
WebClient client = new WebClient(); |
|
|
|
|
String result = client.getContent(mockURL); |
d |
|||
assertEquals("It works", result); |
e |
|
|
|
}
bCreate a mock HttpURLConnection that you set up to return a stream containing It works when the getInputStream method is called on it.
cDo the same for creating a mock URL class and set it up to return the MockURLConnection when url.openConnection is called.
d Call the getContent method to test, passing to it your MockURL instance.
eAssert the result.
Unfortunately, this approach does not work! The JDK URL class is a final class, and no URL interface is available. So much for extensibility….
You need to find another solution and, potentially, another object to mock. One solution is to stub the URLStreamHandlerFactory class. We explored this solution in chapter 6, so let’s find a technique that uses mock objects: refactoring the getContent method. If you think about it, this method does two things: It gets an HttpURLConnection object and then reads the content from it. Refactoring leads to the class shown in listing 7.7 (changes from listing 7.6 are in bold). We have extracted the part that retrieved the HttpURLConnection object.
Listing 7.7 Extracting retrieval of the connection object from getContent
public class WebClient
{
public String getContent(URL url)
{
StringBuffer content = new StringBuffer();
try
{
HttpURLConnection connection =
createHttpURLConnection(url);
b
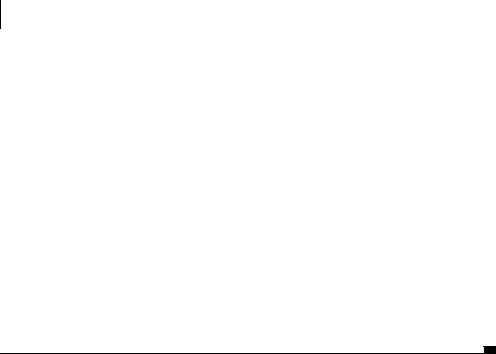
154CHAPTER 7
Testing in isolation with mock objects
InputStream is = connection.getInputStream();
byte[] buffer = new byte[2048]; int count;
while (-1 != (count = is.read(buffer)))
{
content.append(new String(buffer, 0, count));
}
}
catch (IOException e)
{
return null;
}
return content.toString();
}
protected HttpURLConnection createHttpURLConnection(URL url) b throws IOException
{
return (HttpURLConnection) url.openConnection();
}
}
bRefactoring. You now call the createHttpURLConnection method to create the HTTP connection.
How does this solution let you test getContent more effectively? You can now apply a useful trick, which consists of writing a test helper class that extends the WebClient class and overrides its createHttpURLConnection method, as follows:
private class TestableWebClient extends WebClient
{
private HttpURLConnection connection;
public void setHttpURLConnection(HttpURLConnection connection)
{
this.connection = connection;
}
public HttpURLConnection createHttpURLConnection(URL url) throws IOException
{
return this.connection;
}
}
In the test, you can call the setHttpURLConnection method, passing it the mock HttpURLConnection object. The test now becomes the following (differences are shown in bold):