
- •Contents at a Glance
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Preface
- •What This Book Is
- •What You Need
- •Developer Options
- •What You Need to Know
- •What’s Different About Coding for iOS?
- •Only One Active Application
- •Only One Window
- •Limited Access
- •Limited Response Time
- •Limited Screen Size
- •Limited System Resources
- •No Garbage Collection, but…
- •Some New Stuff
- •A Different Approach
- •What’s in This Book
- •What’s New in This Update?
- •Are You Ready?
- •Setting Up Your Project in Xcode
- •The Xcode Workspace Window
- •The Toolbar
- •The Navigator View
- •The Jump Bar
- •The Utility Pane
- •Interface Builder
- •New Compiler and Debugger
- •A Closer Look at Our Project
- •Introducing Xcode’s Interface Builder
- •What’s in the Nib File?
- •The Library
- •Adding a Label to the View
- •Changing Attributes
- •Some iPhone Polish—Finishing Touches
- •Bring It on Home
- •The Model-View-Controller Paradigm
- •Creating Our Project
- •Looking at the View Controller
- •Understanding Outlets and Actions
- •Outlets
- •Actions
- •Cleaning Up the View Controller
- •Designing the User Interface
- •Adding the Buttons and Action Method
- •Adding the Label and Outlet
- •Writing the Action Method
- •Trying It Out
- •Looking at the Application Delegate
- •Bring It on Home
- •A Screen Full of Controls
- •Active, Static, and Passive Controls
- •Creating the Application
- •Implementing the Image View and Text Fields
- •Adding the Image View
- •Resizing the Image View
- •Setting View Attributes
- •The Mode Attribute
- •Interaction Checkboxes
- •The Alpha Value
- •Background
- •Drawing Checkboxes
- •Stretching
- •Adding the Text Fields
- •Text Field Inspector Settings
- •Setting the Attributes for the Second Text Field
- •Creating and Connecting Outlets
- •Closing the Keyboard
- •Closing the Keyboard When Done Is Tapped
- •Touching the Background to Close the Keyboard
- •Adding the Slider and Label
- •Creating and Connecting the Actions and Outlets
- •Implementing the Action Method
- •Adding Two Labeled Switches
- •Connecting and Creating Outlets and Actions
- •Implementing the Switch Actions
- •Adding the Button
- •Connecting and Creating the Button Outlets and Actions
- •Implementing the Segmented Control Action
- •Implementing the Action Sheet and Alert
- •Conforming to the Action Sheet Delegate Method
- •Showing the Action Sheet
- •Spiffing Up the Button
- •Using the viewDidLoad Method
- •Control States
- •Stretchable Images
- •Crossing the Finish Line
- •The Mechanics of Autorotation
- •Points, Pixels, and the Retina Display
- •Autorotation Approaches
- •Handling Rotation Using Autosize Attributes
- •Configuring Supported Orientations
- •Specifying Rotation Support
- •Designing an Interface with Autosize Attributes
- •Using the Size Inspector’s Autosize Attributes
- •Setting the Buttons’ Autosize Attributes
- •Restructuring a View When Rotated
- •Creating and Connecting Outlets
- •Moving the Buttons on Rotation
- •Swapping Views
- •Designing the Two Views
- •Implementing the Swap
- •Changing Outlet Collections
- •Rotating Out of Here
- •Common Types of Multiview Apps
- •The Architecture of a Multiview Application
- •The Root Controller
- •Anatomy of a Content View
- •Building View Switcher
- •Creating Our View Controller and Nib Files
- •Modifying the App Delegate
- •Modifying BIDSwitchViewController.h
- •Adding a View Controller
- •Building a View with a Toolbar
- •Writing the Root View Controller
- •Implementing the Content Views
- •Animating the Transition
- •Switching Off
- •The Pickers Application
- •Delegates and Data Sources
- •Setting Up the Tab Bar Framework
- •Creating the Files
- •Adding the Root View Controller
- •Creating TabBarController.xib
- •The Initial Test Run
- •Implementing the Date Picker
- •Implementing the Single-Component Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller As a Data Source and Delegate
- •Implementing a Multicomponent Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller
- •Implementing Dependent Components
- •Creating a Simple Game with a Custom Picker
- •Writing the Controller Header File
- •Building the View
- •Adding Image Resources
- •Implementing the Controller
- •The spin Method
- •The viewDidLoad Method
- •Final Details
- •Linking in the Audio Toolbox Framework
- •Final Spin
- •Table View Basics
- •Table Views and Table View Cells
- •Grouped and Plain Tables
- •Implementing a Simple Table
- •Designing the View
- •Writing the Controller
- •Adding an Image
- •Using Table View Cell Styles
- •Setting the Indent Level
- •Handling Row Selection
- •Changing the Font Size and Row Height
- •Customizing Table View Cells
- •Adding Subviews to the Table View Cell
- •Creating a UITableViewCell Subclass
- •Adding New Cells
- •Implementing the Controller’s Code
- •Loading a UITableViewCell from a Nib
- •Designing the Table View Cell in Interface Builder
- •Using the New Table View Cell
- •Grouped and Indexed Sections
- •Building the View
- •Importing the Data
- •Implementing the Controller
- •Adding an Index
- •Implementing a Search Bar
- •Rethinking the Design
- •A Deep Mutable Copy
- •Updating the Controller Header File
- •Modifying the View
- •Modifying the Controller Implementation
- •Copying Data from allNames
- •Implementing the Search
- •Changes to viewDidLoad
- •Changes to Data Source Methods
- •Adding a Table View Delegate Method
- •Adding Search Bar Delegate Methods
- •Adding a Magnifying Glass to the Index
- •Adding the Special Value to the Keys Array
- •Suppressing the Section Header
- •Telling the Table View What to Do
- •Putting It All on the Table
- •Navigation Controller Basics
- •Stacky Goodness
- •A Stack of Controllers
- •Nav, a Hierarchical Application in Six Parts
- •Meet the Subcontrollers
- •The Disclosure Button View
- •The Checklist View
- •The Rows Control View
- •The Movable Rows View
- •The Deletable Rows View
- •The Editable Detail View
- •The Nav Application’s Skeleton
- •Creating the Top-Level View Controller
- •Setting Up the Navigation Controller
- •Adding the Images to the Project
- •First Subcontroller: The Disclosure Button View
- •Creating the Detail View
- •Modifying the Disclosure Button Controller
- •Adding a Disclosure Button Controller Instance
- •Second Subcontroller: The Checklist
- •Creating the Checklist View
- •Adding a Checklist Controller Instance
- •Third Subcontroller: Controls on Table Rows
- •Creating the Row Controls View
- •Adding a Rows Control Controller Instance
- •Fourth Subcontroller: Movable Rows
- •Creating the Movable Row View
- •Adding a Move Me Controller Instance
- •Fifth Subcontroller: Deletable Rows
- •Creating the Deletable Rows View
- •Adding a Delete Me Controller Instance
- •Sixth Subcontroller: An Editable Detail Pane
- •Creating the Data Model Object
- •Creating the Detail View List Controller
- •Creating the Detail View Controller
- •Adding an Editable Detail View Controller Instance
- •But There’s One More Thing. . .
- •Breaking the Tape
- •Creating a Simple Storyboard
- •Dynamic Prototype Cells
- •Dynamic Table Content, Storyboard-Style
- •Editing Prototype Cells
- •Good Old Table View Data Source
- •Will It Load?
- •Static Cells
- •Going Static
- •So Long, Good Old Table View Data Source
- •You Say Segue, I Say Segue
- •Creating Segue Navigator
- •Filling the Blank Slate
- •First Transition
- •A Slightly More Useful Task List
- •Viewing Task Details
- •Make More Segues, Please
- •Passing a Task from the List
- •Handling Task Details
- •Passing Back Details
- •Making the List Receive the Details
- •If Only We Could End with a Smooth Transition
- •Split Views and Popovers
- •Creating a SplitView Project
- •The Storyboard Defines the Structure
- •The Code Defines the Functionality
- •The App Delegate
- •The Master View Controller
- •The Detail View Controller
- •Here Come the Presidents
- •Creating Your Own Popover
- •iPad Wrap-Up
- •Getting to Know Your Settings Bundle
- •The AppSettings Application
- •Creating the Project
- •Working with the Settings Bundle
- •Adding a Settings Bundle to Our Project
- •Setting Up the Property List
- •Adding a Text Field Setting
- •Adding an Application Icon
- •Adding a Secure Text Field Setting
- •Adding a Multivalue Field
- •Adding a Toggle Switch Setting
- •Adding the Slider Setting
- •Adding Icons to the Settings Bundle
- •Adding a Child Settings View
- •Reading Settings in Our Application
- •Retrieving User Settings
- •Creating the Main View
- •Updating the Main View Controller
- •Registering Default Values
- •Changing Defaults from Our Application
- •Keeping It Real
- •Beam Me Up, Scotty
- •Your Application’s Sandbox
- •Getting the Documents Directory
- •Getting the tmp Directory
- •File-Saving Strategies
- •Single-File Persistence
- •Multiple-File Persistence
- •Using Property Lists
- •Property List Serialization
- •The First Version of the Persistence Application
- •Creating the Persistence Project
- •Designing the Persistence Application View
- •Editing the Persistence Classes
- •Archiving Model Objects
- •Conforming to NSCoding
- •Implementing NSCopying
- •Archiving and Unarchiving Data Objects
- •The Archiving Application
- •Implementing the BIDFourLines Class
- •Implementing the BIDViewController Class
- •Using iOS’s Embedded SQLite3
- •Creating or Opening the Database
- •Using Bind Variables
- •The SQLite3 Application
- •Linking to the SQLite3 Library
- •Modifying the Persistence View Controller
- •Using Core Data
- •Entities and Managed Objects
- •Key-Value Coding
- •Putting It All in Context
- •Creating New Managed Objects
- •Retrieving Managed Objects
- •The Core Data Application
- •Designing the Data Model
- •Creating the Persistence View and Controller
- •Persistence Rewarded
- •Managing Document Storage with UIDocument
- •Building TinyPix
- •Creating BIDTinyPixDocument
- •Code Master
- •Initial Storyboarding
- •Creating BIDTinyPixView
- •Storyboard Detailing
- •Adding iCloud Support
- •Creating a Provisioning Profile
- •Enabling iCloud Entitlements
- •How to Query
- •Save Where?
- •Storing Preferences on iCloud
- •What We Didn’t Cover
- •Grand Central Dispatch
- •Introducing SlowWorker
- •Threading Basics
- •Units of Work
- •GCD: Low-Level Queueing
- •Becoming a Blockhead
- •Improving SlowWorker
- •Don’t Forget That Main Thread
- •Giving Some Feedback
- •Concurrent Blocks
- •Background Processing
- •Application Life Cycle
- •State-Change Notifications
- •Creating State Lab
- •Exploring Execution States
- •Making Use of Execution State Changes
- •Handling the Inactive State
- •Handling the Background State
- •Removing Resources When Entering the Background
- •Saving State When Entering the Background
- •A Brief Journey to Yesteryear
- •Back to the Background
- •Requesting More Backgrounding Time
- •Grand Central Dispatch, Over and Out
- •Two Views of a Graphical World
- •The Quartz 2D Approach to Drawing
- •Quartz 2D’s Graphics Contexts
- •The Coordinate System
- •Specifying Colors
- •A Bit of Color Theory for Your iOS Device’s Display
- •Other Color Models
- •Color Convenience Methods
- •Drawing Images in Context
- •Drawing Shapes: Polygons, Lines, and Curves
- •The QuartzFun Application
- •Setting Up the QuartzFun Application
- •Creating a Random Color
- •Defining Application Constants
- •Implementing the QuartzFunView Skeleton
- •Creating and Connecting Outlets and Actions
- •Implementing the Action Methods
- •Adding Quartz 2D Drawing Code
- •Drawing the Line
- •Drawing the Rectangle and Ellipse
- •Drawing the Image
- •Optimizing the QuartzFun Application
- •The GLFun Application
- •Setting Up the GLFun Application
- •Creating BIDGLFunView
- •Updating BIDViewController
- •Updating the Nib
- •Finishing GLFun
- •Drawing to a Close
- •Multitouch Terminology
- •The Responder Chain
- •Responding to Events
- •Forwarding an Event: Keeping the Responder Chain Alive
- •The Multitouch Architecture
- •The Four Touch Notification Methods
- •The TouchExplorer Application
- •The Swipes Application
- •Automatic Gesture Recognition
- •Implementing Multiple Swipes
- •Detecting Multiple Taps
- •Detecting Pinches
- •Defining Custom Gestures
- •The CheckPlease Application
- •The CheckPlease Touch Methods
- •Garçon? Check, Please!
- •The Location Manager
- •Setting the Desired Accuracy
- •Setting the Distance Filter
- •Starting the Location Manager
- •Using the Location Manager Wisely
- •The Location Manager Delegate
- •Getting Location Updates
- •Getting Latitude and Longitude Using CLLocation
- •Error Notifications
- •Trying Out Core Location
- •Updating Location Manager
- •Determining Distance Traveled
- •Wherever You Go, There You Are
- •Accelerometer Physics
- •Don’t Forget Rotation
- •Core Motion and the Motion Manager
- •Event-Based Motion
- •Proactive Motion Access
- •Accelerometer Results
- •Detecting Shakes
- •Baked-In Shaking
- •Shake and Break
- •Accelerometer As Directional Controller
- •Rolling Marbles
- •Writing the Ball View
- •Calculating Ball Movement
- •Rolling On
- •Using the Image Picker and UIImagePickerController
- •Implementing the Image Picker Controller Delegate
- •Road Testing the Camera and Library
- •Designing the Interface
- •Implementing the Camera View Controller
- •It’s a Snap!
- •Localization Architecture
- •Strings Files
- •What’s in a Strings File?
- •The Localized String Macro
- •Real-World iOS: Localizing Your Application
- •Setting Up LocalizeMe
- •Trying Out LocalizeMe
- •Localizing the Nib
- •Localizing an Image
- •Generating and Localizing a Strings File
- •Localizing the App Display Name
- •Auf Wiedersehen
- •Apple’s Documentation
- •Mailing Lists
- •Discussion Forums
- •Web Sites
- •Blogs
- •Conferences
- •Follow the Authors
- •Farewell
- •Index

CHAPTER 11: iPad Considerations |
401 |
Creating Your Own Popover
There’s still one piece of iPad GUI technology that we haven’t dealt with in quite enough detail yet: the creation and display of your own popover. So far, we’ve had a UIPopoverController handed to us from a UISplitView delegate method, which let us keep track of it in an instance variable so we could force it to go away, but popovers really come in handy when you want to present your own view controllers.
To see how this works, we’re going to add a popover to be activated by a permanent toolbar item (unlike the one that the UISplitView delegate method gives us, which is meant to come and go). This popover will display a table view containing a list of languages. If the user picks a language from the list, the web view will load whatever Wikipedia entry was already showing in the new language. This will be simple enough to do, since switching from one language to another in Wikipedia is just a matter of changing a small piece of the URL that contains an embedded country code.
NOTE: Both uses of popovers in this example are in the service of showing a UITableView, but don’t let that mislead you—UIPopoverController can be used to handle the display of any view controller content you like! We’re sticking with table views for this example because it’s a common use case, it’s easy to show in a relatively small amount of code, and it’s something with which you should already be quite familiar.
Start off by right-clicking the Presidents folder in Xcode and selecting New File… from the contextual menu. When the assistant appears, select Cocoa Touch, then select UIViewController subclass, and then click Next. On the next screen, name the new class
BIDLanguageListController and select UITableViewController from the Subclass of field. Turn on the checkbox next to Targeted for iPad, and turn off the checkbox next to With XIB for user interface. Click Next, double-check the location where you’re saving the file, and click Create.
The BIDLanguageListController is going to be a pretty standard table view controller class. It will display a list of items and let the detail view controller know when a choice is made by using a pointer back to the detail view controller. Edit BIDLanguageListController.h, adding the bold lines shown here:
#import <UIKit/UIKit.h>
@class BIDDetailViewController;
@interface BIDLanguageListController : UITableViewController
@property (weak, nonatomic) BIDDetailViewController *detailViewController; @property (strong, nonatomic) NSArray *languageNames;
@property (strong, nonatomic) NSArray *languageCodes;
@end
www.it-ebooks.info

402 |
CHAPTER 11: iPad Considerations |
These additions define a pointer back to the detail view controller (which we’ll set from code in the detail view controller itself when we’re about to display the language list), as well as a pair of arrays for containing the values that will be displayed (English, French, and so on) and the underlying values that will be used to build an URL from the chosen language (en, fr, and so on).
If you copied and pasted this code from the book’s source archive (or e-book) into your own project or typed it yourself a little sloppily, you may not have noticed an important difference in how the detailViewController property was declared earlier. Unlike most properties that reference an object pointer, we declared this one using weak instead of strong. This is something that we must do in order to avoid a retain cycle.
What’s a retain cycle? It’s a situation where a set of two or more objects have retained one another in a circular fashion. Each object has a retain counter of one or higher and will therefore never release the pointers it contains, so they will never be deallocated either. Most potential retain cycles can be avoided by carefully considering the creation of your objects, often by trying to figure out who “owns” whom. In this sense, an instance of BIDDetailViewController owns an instance of BIDLanguageListController, because it’s the BIDDetailViewController that actually creates the BIDLanguageListController in order to get a piece of work done. Whenever you have a pair of objects that each needs to refer to one another, you’ll usually want the owner object to retain the other object, while the other object should specifically not retain its owner. Since we’re using the ARC feature that Apple introduced in Xcode 4.2, the compiler does most of the work for us. Instead of paying attention to the details about releasing and retaining objects, all we need to do is declare a property with the weak keyword instead of strong. ARC will do the rest!
Now, switch over to BIDLanguageListController.m to implement the following changes. At the top of the file, start by importing the header for BIDDetailViewController, and then synthesize getters and setters for the properties you declared:
#import "BIDLanguageListController.h"
#import "BIDDetailViewController.h"
@implementation BIDLanguageListController
@synthesize languageNames; @synthesize languageCodes; @synthesize detailViewController;
.
.
.
Then scroll down a bit to the viewDidLoad method, and add a bit of setup code:
- (void)viewDidLoad { [super viewDidLoad];
self.languageNames = [NSArray arrayWithObjects:@"English", @"French", @"German", @"Spanish", nil];
self.languageCodes = [NSArray arrayWithObjects:@"en", @"fr", @"de", @"es", nil]; self.clearsSelectionOnViewWillAppear = NO;
www.it-ebooks.info

CHAPTER 11: iPad Considerations |
403 |
self.contentSizeForViewInPopover = CGSizeMake(320.0, [self.languageCodes count] * 44.0);
}
This sets up the language arrays and also defines the size that this view will use if shown in a popover (which, as we know, it will be). Without defining the size, we would end up with a popover stretching vertically to fill nearly the whole screen, even with only four entries in it.
Farther down, we have a few methods generated by Xcode’s template that don’t contain particularly useful code—just a warning and some placeholder text. Let’s replace those with something real:
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView { #warning Potentially incomplete method implementation.
// Return the number of sections. return 0;
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
#warning Incomplete method implementation.
// Return the number of rows in the section. return 0;
return [self.languageCodes count];
}
Then add a line near the end of tableView:cellForRowAtIndexPath: to put a language name into a cell:
// Configure the cell.
cell.textLabel.text = [languageNames objectAtIndex:[indexPath row]]; return cell;
Next, fix tableView:didSelectRowAtIndexPath: by eliminating the comment block it contains and adding this new code instead:
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
detailViewController.languageString = [self.languageCodes objectAtIndex: [indexPath row]];
}
Note that BIDDetailViewController doesn’t actually have a languageString property. We’ll take care of that in just a bit. But first, finish up BIDLanguageListController by making the following bookkeeping changes:
- (void)viewDidUnload { [super viewDidUnload];
self.detailViewController = nil; self.languageNames = nil; self.languageCodes = nil;
}
www.it-ebooks.info

404 |
CHAPTER 11: iPad Considerations |
Now, it’s time to make the changes required for BIDDetailViewController to handle the popover, as well as generate the correct URL whenever the user either changes the display language or picks a different president. Start by making the following changes in
BIDDetailViewController.h:
#import <UIKit/UIKit.h>
@interface BIDDetailViewController : UIViewController <UISplitViewControllerDelegate>
@property (strong, nonatomic) id detailItem;
@property (strong, nonatomic) IBOutlet UILabel *detailDescriptionLabel; @property (weak, nonatomic) IBOutlet UIWebView *webView;
@property (strong, nonatomic) UIBarButtonItem *languageButton;
@property (strong, nonatomic) UIPopoverController *languagePopoverController; @property (copy, nonatomic) NSString *languageString;
- (IBAction)touchLanguageButton;
@end
All we need to do now is fix BIDDetailViewController.m so that it can handle the language popover and the URL construction. Start by adding this import somewhere at the top:
#import "BIDLanguageListController.h"
Then synthesize the new properties just below the @implementation line:
@synthesize languageButton;
@synthesize languagePopoverController;
@synthesize languageString;
The next thing we’re going to add is a function that takes as arguments a URL pointing to a Wikipedia page and a two-letter language code, and returns a URL that combines the two. We’ll use this at appropriate spots in our controller code later. You can place this function just about anywhere, including within the class’s implementation. The compiler is smart enough to always treat a function as just a function. Why don’t you place it just after the last synthesize statement toward the top of the file?
static NSString * modifyUrlForLanguage(NSString *url, NSString *lang) { if (!lang) {
return url;
}
//We're relying on a particular Wikipedia URL format here. This
//is a bit fragile!
NSRange languageCodeRange = NSMakeRange(7, 2);
if ([[url substringWithRange:languageCodeRange] isEqualToString:lang]) { return url;
} else {
NSString *newUrl = [url stringByReplacingCharactersInRange:languageCodeRange withString:lang];
return newUrl;
}
}
www.it-ebooks.info
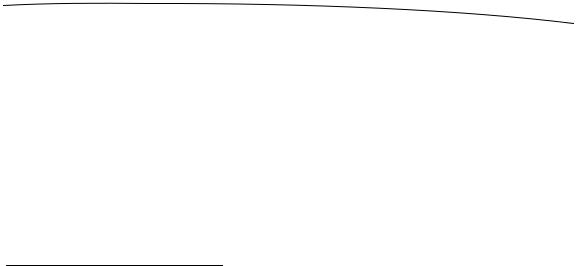
CHAPTER 11: iPad Considerations |
405 |
Why make this a function instead of a method? There are a couple of reasons. First, instance methods in a class are typically meant to do something involving one or more instance variables. This function does not make use of any instance variables. It simply performs an operation on two strings and returns another. We could have made it a class method, but even that feels a bit wrong, since what the method does isn’t really related specifically to our controller class. Sometimes, a function is just what you need.
Our next move is to update the setDetailItem: method. This method will use the function we just defined to combine the URL that’s passed in with the chosen languageString to generate the correct URL. It also makes sure that our second popover, if present, disappears just like the first popover (the one that was defined for us) does.
- (void)setDetailItem:(id)newDetailItem { if (detailItem != newDetailItem) {
_detailItem = newDetailItem;
_detailItem = modifyUrlForLanguage(newDetailItem, languageString);
// Update the view. [self configureView];
}
if (self.masterPopoverController != nil) { [self.masterPopoverController dismissPopoverAnimated:YES];
}
}
Now, let’s update the viewDidLoad method. Here, we’re going to create a UIBarButtonItem and put it into the UINavigationItem at the top of the screen.
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib. self.languageButton = [[UIBarButtonItem alloc] init]; languageButton.title = @"Choose Language";
languageButton.target = self;
languageButton.action = @selector(touchLanguageButton); self.navigationItem.rightBarButtonItem = self.languageButton;
[self configureView];
}
Next, we implement setLanguageString:. This also calls our modifyUrlForLanguage() function so that the URL can be regenerated (and the new page loaded) immediately. Add this method to the bottom of the file, just above the @end:
- (void)setLanguageString:(NSString *)newString {
if (![newString isEqualToString:languageString]) { languageString = [newString copy];
self.detailItem = modifyUrlForLanguage(_detailItem, languageString);
}
if (languagePopoverController != nil) { [languagePopoverController dismissPopoverAnimated:YES];
www.it-ebooks.info