
- •Contents at a Glance
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Preface
- •What This Book Is
- •What You Need
- •Developer Options
- •What You Need to Know
- •What’s Different About Coding for iOS?
- •Only One Active Application
- •Only One Window
- •Limited Access
- •Limited Response Time
- •Limited Screen Size
- •Limited System Resources
- •No Garbage Collection, but…
- •Some New Stuff
- •A Different Approach
- •What’s in This Book
- •What’s New in This Update?
- •Are You Ready?
- •Setting Up Your Project in Xcode
- •The Xcode Workspace Window
- •The Toolbar
- •The Navigator View
- •The Jump Bar
- •The Utility Pane
- •Interface Builder
- •New Compiler and Debugger
- •A Closer Look at Our Project
- •Introducing Xcode’s Interface Builder
- •What’s in the Nib File?
- •The Library
- •Adding a Label to the View
- •Changing Attributes
- •Some iPhone Polish—Finishing Touches
- •Bring It on Home
- •The Model-View-Controller Paradigm
- •Creating Our Project
- •Looking at the View Controller
- •Understanding Outlets and Actions
- •Outlets
- •Actions
- •Cleaning Up the View Controller
- •Designing the User Interface
- •Adding the Buttons and Action Method
- •Adding the Label and Outlet
- •Writing the Action Method
- •Trying It Out
- •Looking at the Application Delegate
- •Bring It on Home
- •A Screen Full of Controls
- •Active, Static, and Passive Controls
- •Creating the Application
- •Implementing the Image View and Text Fields
- •Adding the Image View
- •Resizing the Image View
- •Setting View Attributes
- •The Mode Attribute
- •Interaction Checkboxes
- •The Alpha Value
- •Background
- •Drawing Checkboxes
- •Stretching
- •Adding the Text Fields
- •Text Field Inspector Settings
- •Setting the Attributes for the Second Text Field
- •Creating and Connecting Outlets
- •Closing the Keyboard
- •Closing the Keyboard When Done Is Tapped
- •Touching the Background to Close the Keyboard
- •Adding the Slider and Label
- •Creating and Connecting the Actions and Outlets
- •Implementing the Action Method
- •Adding Two Labeled Switches
- •Connecting and Creating Outlets and Actions
- •Implementing the Switch Actions
- •Adding the Button
- •Connecting and Creating the Button Outlets and Actions
- •Implementing the Segmented Control Action
- •Implementing the Action Sheet and Alert
- •Conforming to the Action Sheet Delegate Method
- •Showing the Action Sheet
- •Spiffing Up the Button
- •Using the viewDidLoad Method
- •Control States
- •Stretchable Images
- •Crossing the Finish Line
- •The Mechanics of Autorotation
- •Points, Pixels, and the Retina Display
- •Autorotation Approaches
- •Handling Rotation Using Autosize Attributes
- •Configuring Supported Orientations
- •Specifying Rotation Support
- •Designing an Interface with Autosize Attributes
- •Using the Size Inspector’s Autosize Attributes
- •Setting the Buttons’ Autosize Attributes
- •Restructuring a View When Rotated
- •Creating and Connecting Outlets
- •Moving the Buttons on Rotation
- •Swapping Views
- •Designing the Two Views
- •Implementing the Swap
- •Changing Outlet Collections
- •Rotating Out of Here
- •Common Types of Multiview Apps
- •The Architecture of a Multiview Application
- •The Root Controller
- •Anatomy of a Content View
- •Building View Switcher
- •Creating Our View Controller and Nib Files
- •Modifying the App Delegate
- •Modifying BIDSwitchViewController.h
- •Adding a View Controller
- •Building a View with a Toolbar
- •Writing the Root View Controller
- •Implementing the Content Views
- •Animating the Transition
- •Switching Off
- •The Pickers Application
- •Delegates and Data Sources
- •Setting Up the Tab Bar Framework
- •Creating the Files
- •Adding the Root View Controller
- •Creating TabBarController.xib
- •The Initial Test Run
- •Implementing the Date Picker
- •Implementing the Single-Component Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller As a Data Source and Delegate
- •Implementing a Multicomponent Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller
- •Implementing Dependent Components
- •Creating a Simple Game with a Custom Picker
- •Writing the Controller Header File
- •Building the View
- •Adding Image Resources
- •Implementing the Controller
- •The spin Method
- •The viewDidLoad Method
- •Final Details
- •Linking in the Audio Toolbox Framework
- •Final Spin
- •Table View Basics
- •Table Views and Table View Cells
- •Grouped and Plain Tables
- •Implementing a Simple Table
- •Designing the View
- •Writing the Controller
- •Adding an Image
- •Using Table View Cell Styles
- •Setting the Indent Level
- •Handling Row Selection
- •Changing the Font Size and Row Height
- •Customizing Table View Cells
- •Adding Subviews to the Table View Cell
- •Creating a UITableViewCell Subclass
- •Adding New Cells
- •Implementing the Controller’s Code
- •Loading a UITableViewCell from a Nib
- •Designing the Table View Cell in Interface Builder
- •Using the New Table View Cell
- •Grouped and Indexed Sections
- •Building the View
- •Importing the Data
- •Implementing the Controller
- •Adding an Index
- •Implementing a Search Bar
- •Rethinking the Design
- •A Deep Mutable Copy
- •Updating the Controller Header File
- •Modifying the View
- •Modifying the Controller Implementation
- •Copying Data from allNames
- •Implementing the Search
- •Changes to viewDidLoad
- •Changes to Data Source Methods
- •Adding a Table View Delegate Method
- •Adding Search Bar Delegate Methods
- •Adding a Magnifying Glass to the Index
- •Adding the Special Value to the Keys Array
- •Suppressing the Section Header
- •Telling the Table View What to Do
- •Putting It All on the Table
- •Navigation Controller Basics
- •Stacky Goodness
- •A Stack of Controllers
- •Nav, a Hierarchical Application in Six Parts
- •Meet the Subcontrollers
- •The Disclosure Button View
- •The Checklist View
- •The Rows Control View
- •The Movable Rows View
- •The Deletable Rows View
- •The Editable Detail View
- •The Nav Application’s Skeleton
- •Creating the Top-Level View Controller
- •Setting Up the Navigation Controller
- •Adding the Images to the Project
- •First Subcontroller: The Disclosure Button View
- •Creating the Detail View
- •Modifying the Disclosure Button Controller
- •Adding a Disclosure Button Controller Instance
- •Second Subcontroller: The Checklist
- •Creating the Checklist View
- •Adding a Checklist Controller Instance
- •Third Subcontroller: Controls on Table Rows
- •Creating the Row Controls View
- •Adding a Rows Control Controller Instance
- •Fourth Subcontroller: Movable Rows
- •Creating the Movable Row View
- •Adding a Move Me Controller Instance
- •Fifth Subcontroller: Deletable Rows
- •Creating the Deletable Rows View
- •Adding a Delete Me Controller Instance
- •Sixth Subcontroller: An Editable Detail Pane
- •Creating the Data Model Object
- •Creating the Detail View List Controller
- •Creating the Detail View Controller
- •Adding an Editable Detail View Controller Instance
- •But There’s One More Thing. . .
- •Breaking the Tape
- •Creating a Simple Storyboard
- •Dynamic Prototype Cells
- •Dynamic Table Content, Storyboard-Style
- •Editing Prototype Cells
- •Good Old Table View Data Source
- •Will It Load?
- •Static Cells
- •Going Static
- •So Long, Good Old Table View Data Source
- •You Say Segue, I Say Segue
- •Creating Segue Navigator
- •Filling the Blank Slate
- •First Transition
- •A Slightly More Useful Task List
- •Viewing Task Details
- •Make More Segues, Please
- •Passing a Task from the List
- •Handling Task Details
- •Passing Back Details
- •Making the List Receive the Details
- •If Only We Could End with a Smooth Transition
- •Split Views and Popovers
- •Creating a SplitView Project
- •The Storyboard Defines the Structure
- •The Code Defines the Functionality
- •The App Delegate
- •The Master View Controller
- •The Detail View Controller
- •Here Come the Presidents
- •Creating Your Own Popover
- •iPad Wrap-Up
- •Getting to Know Your Settings Bundle
- •The AppSettings Application
- •Creating the Project
- •Working with the Settings Bundle
- •Adding a Settings Bundle to Our Project
- •Setting Up the Property List
- •Adding a Text Field Setting
- •Adding an Application Icon
- •Adding a Secure Text Field Setting
- •Adding a Multivalue Field
- •Adding a Toggle Switch Setting
- •Adding the Slider Setting
- •Adding Icons to the Settings Bundle
- •Adding a Child Settings View
- •Reading Settings in Our Application
- •Retrieving User Settings
- •Creating the Main View
- •Updating the Main View Controller
- •Registering Default Values
- •Changing Defaults from Our Application
- •Keeping It Real
- •Beam Me Up, Scotty
- •Your Application’s Sandbox
- •Getting the Documents Directory
- •Getting the tmp Directory
- •File-Saving Strategies
- •Single-File Persistence
- •Multiple-File Persistence
- •Using Property Lists
- •Property List Serialization
- •The First Version of the Persistence Application
- •Creating the Persistence Project
- •Designing the Persistence Application View
- •Editing the Persistence Classes
- •Archiving Model Objects
- •Conforming to NSCoding
- •Implementing NSCopying
- •Archiving and Unarchiving Data Objects
- •The Archiving Application
- •Implementing the BIDFourLines Class
- •Implementing the BIDViewController Class
- •Using iOS’s Embedded SQLite3
- •Creating or Opening the Database
- •Using Bind Variables
- •The SQLite3 Application
- •Linking to the SQLite3 Library
- •Modifying the Persistence View Controller
- •Using Core Data
- •Entities and Managed Objects
- •Key-Value Coding
- •Putting It All in Context
- •Creating New Managed Objects
- •Retrieving Managed Objects
- •The Core Data Application
- •Designing the Data Model
- •Creating the Persistence View and Controller
- •Persistence Rewarded
- •Managing Document Storage with UIDocument
- •Building TinyPix
- •Creating BIDTinyPixDocument
- •Code Master
- •Initial Storyboarding
- •Creating BIDTinyPixView
- •Storyboard Detailing
- •Adding iCloud Support
- •Creating a Provisioning Profile
- •Enabling iCloud Entitlements
- •How to Query
- •Save Where?
- •Storing Preferences on iCloud
- •What We Didn’t Cover
- •Grand Central Dispatch
- •Introducing SlowWorker
- •Threading Basics
- •Units of Work
- •GCD: Low-Level Queueing
- •Becoming a Blockhead
- •Improving SlowWorker
- •Don’t Forget That Main Thread
- •Giving Some Feedback
- •Concurrent Blocks
- •Background Processing
- •Application Life Cycle
- •State-Change Notifications
- •Creating State Lab
- •Exploring Execution States
- •Making Use of Execution State Changes
- •Handling the Inactive State
- •Handling the Background State
- •Removing Resources When Entering the Background
- •Saving State When Entering the Background
- •A Brief Journey to Yesteryear
- •Back to the Background
- •Requesting More Backgrounding Time
- •Grand Central Dispatch, Over and Out
- •Two Views of a Graphical World
- •The Quartz 2D Approach to Drawing
- •Quartz 2D’s Graphics Contexts
- •The Coordinate System
- •Specifying Colors
- •A Bit of Color Theory for Your iOS Device’s Display
- •Other Color Models
- •Color Convenience Methods
- •Drawing Images in Context
- •Drawing Shapes: Polygons, Lines, and Curves
- •The QuartzFun Application
- •Setting Up the QuartzFun Application
- •Creating a Random Color
- •Defining Application Constants
- •Implementing the QuartzFunView Skeleton
- •Creating and Connecting Outlets and Actions
- •Implementing the Action Methods
- •Adding Quartz 2D Drawing Code
- •Drawing the Line
- •Drawing the Rectangle and Ellipse
- •Drawing the Image
- •Optimizing the QuartzFun Application
- •The GLFun Application
- •Setting Up the GLFun Application
- •Creating BIDGLFunView
- •Updating BIDViewController
- •Updating the Nib
- •Finishing GLFun
- •Drawing to a Close
- •Multitouch Terminology
- •The Responder Chain
- •Responding to Events
- •Forwarding an Event: Keeping the Responder Chain Alive
- •The Multitouch Architecture
- •The Four Touch Notification Methods
- •The TouchExplorer Application
- •The Swipes Application
- •Automatic Gesture Recognition
- •Implementing Multiple Swipes
- •Detecting Multiple Taps
- •Detecting Pinches
- •Defining Custom Gestures
- •The CheckPlease Application
- •The CheckPlease Touch Methods
- •Garçon? Check, Please!
- •The Location Manager
- •Setting the Desired Accuracy
- •Setting the Distance Filter
- •Starting the Location Manager
- •Using the Location Manager Wisely
- •The Location Manager Delegate
- •Getting Location Updates
- •Getting Latitude and Longitude Using CLLocation
- •Error Notifications
- •Trying Out Core Location
- •Updating Location Manager
- •Determining Distance Traveled
- •Wherever You Go, There You Are
- •Accelerometer Physics
- •Don’t Forget Rotation
- •Core Motion and the Motion Manager
- •Event-Based Motion
- •Proactive Motion Access
- •Accelerometer Results
- •Detecting Shakes
- •Baked-In Shaking
- •Shake and Break
- •Accelerometer As Directional Controller
- •Rolling Marbles
- •Writing the Ball View
- •Calculating Ball Movement
- •Rolling On
- •Using the Image Picker and UIImagePickerController
- •Implementing the Image Picker Controller Delegate
- •Road Testing the Camera and Library
- •Designing the Interface
- •Implementing the Camera View Controller
- •It’s a Snap!
- •Localization Architecture
- •Strings Files
- •What’s in a Strings File?
- •The Localized String Macro
- •Real-World iOS: Localizing Your Application
- •Setting Up LocalizeMe
- •Trying Out LocalizeMe
- •Localizing the Nib
- •Localizing an Image
- •Generating and Localizing a Strings File
- •Localizing the App Display Name
- •Auf Wiedersehen
- •Apple’s Documentation
- •Mailing Lists
- •Discussion Forums
- •Web Sites
- •Blogs
- •Conferences
- •Follow the Authors
- •Farewell
- •Index

CHAPTER 5: Autorotation and Autosizing |
115 |
Fortunately, you don’t need to do a thing in most situations to account for this. When we work with on-screen elements, we specify dimensions and distances in points, not in pixels. For older iPhones and all iPads, points and pixels are equivalent. One point is one pixel. On more recent model iPhones and iPod touches, however, a point equates to 4 pixels and the screen is still 320 × 480 points, even though there are actually 640
× 960 pixels. Think of it as a “virtual resolution,” with iOS automatically mapping points to the physical pixels of your screen. We’ll talk more about this in Chapter 16.
In typical applications, most of the work in actually moving the pixels around the screen is managed by iOS. Your application’s main job in all this is making sure everything fits nicely and looks proper in the resized window.
Autorotation Approaches
Your application can take three general approaches when managing rotation. Which one you use depends on the complexity of your interface. We’ll look at all three approaches in this chapter.
With simpler interfaces, you can specify the correct autosize attributes for all of the objects that make up your interface. Autosize attributes tell the iOS device how your controls should behave when their enclosing view is resized. If you’ve worked with Cocoa on Mac OS X, you’re already familiar with the basic process, because it is the same one used to specify how Cocoa controls behave when the user resizes the window in which they are contained.
Autosize attributes are quick and easy to use, but they aren’t appropriate for all applications. More complex interfaces must handle autorotation in a different manner. For more complex views, you have two additional approaches:
Manually reposition the objects in your view in code when notified that your view is rotating.
Actually design two different versions of your view in Xcode’s Interface Builder: one view for portrait mode and a separate view for landscape mode.
In both cases, you will need to override methods from UIViewController in your view’s controller class.
Let’s get started, shall we? We’ll look at autosizing first.
Handling Rotation Using Autosize Attributes
We’ll create a simple app to demonstrate using autosize attributes. Start a new Single View Application project in Xcode, and call it Autosize. Choose iPhone as the Device Family and make sure to use ARC. Before we lay out our GUI in a nib file, we need to tell iOS that our view supports autorotation. We do that by modifying the view controller class.
www.it-ebooks.info
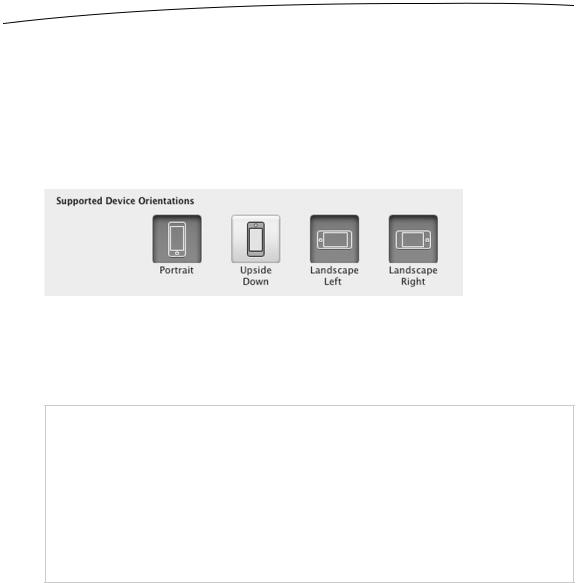
116 |
CHAPTER 5: Autorotation and Autosizing |
Configuring Supported Orientations
First, we need to specify which orientations our application supports. When your window appeared, it should have opened to your project settings. If not, click the top line in the project navigator (the one named after your project), and then make sure you’re on the Summary tab. Among the options available in the summary, you should see a section called iPhone / iPod Deployment Info and, within that, a section called
Supported Device Orientations (see Figure 5–2).
Figure 5–2. The Summary tab for our project shows, among other things, the supported device orientations.
This is how you identify which orientations your application supports. It doesn’t necessarily mean that every view in your application will use all of the selected orientations, but if you’re going to support an orientation in any of your application’s views, that orientation must be selected here.
NOTE: The four buttons shown in Figure 5–2 are actually just a shortcut to adding and deleting entries in your application’s Info.plist file. If you single-click Autosize-Info.plist in the Supporting
Files folder in the project navigator, you should see an entry called either
UISupportedInterfaceOrientations or Supported interface orientations, with three subentries for the three orientations currently selected. Selecting and deselecting those buttons in the project
summary simply adds and removes items from this array. Using the buttons is easier and less prone to error, so we definitely recommend using the buttons, but we thought you should know
what they do.
Have you noticed that the Upside Down orientation is off by default? That’s because if the phone rings while it is being held upside down, the phone is likely to remain upside down when you answer it. iPad app projects default to all four orientations being supported because the iPad is meant to be used in any orientation. Since our project is an iPhone project, we can leave the buttons as they are set.
We’ve identified the orientations our app will support, but that’s not all we need to do. We also must specify for each view controller which orientations are supported, and that must be a subset of the orientations selected here.
www.it-ebooks.info
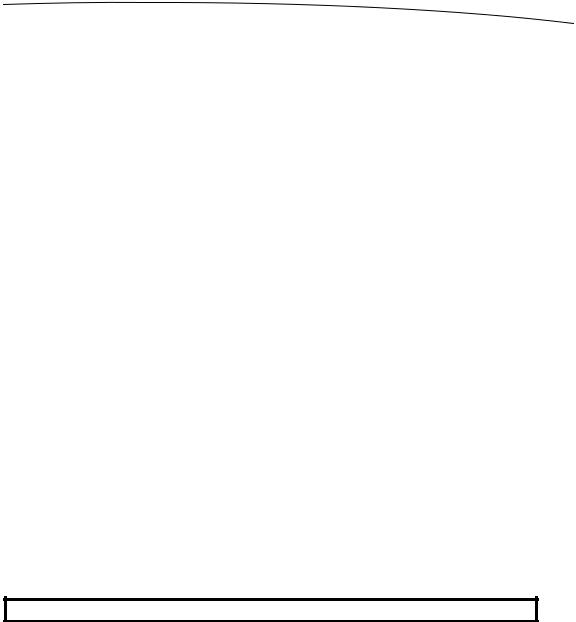
CHAPTER 5: Autorotation and Autosizing |
117 |
Specifying Rotation Support
Single-click BIDViewController.m. In the code that’s already there, you’ll see a method called shouldAutorotateToInterfaceOrientation: provided for you, courtesy of the template:
- (BOOL)shouldAutorotateToInterfaceOrientation: (UIInterfaceOrientation)interfaceOrientation {
// Return YES for supported orientations
return (interfaceOrientation != UIInterfaceOrientationPortraitUpsideDown);
}
This method is iOS’s way of asking a view controller if it’s OK to rotate to a specific orientation. Four defined orientations correspond to the four general ways that an iOS device can be held:
UIInterfaceOrientationPortrait
UIInterfaceOrientationPortraitUpsideDown
UIInterfaceOrientationLandscapeLeft
UIInterfaceOrientationLandscapeRight
In the case of the iPhone, the template defaults to supporting all orientations except upside down, just as you saw in the supported orientations. If we had instead created an iPad project, the default version of the shouldAutorotateToInterfaceOrientation: method created by the template would just return YES.
When the iOS device is changed to a new orientation, the shouldAutorotateToInterfaceOrientation: method is called on the active view controller. The parameter interfaceOrientation will contain one of the four values in the preceding list, and this method needs to return either YES or NO to signify whether the application’s window should be rotated to match the new orientation. Because every view controller subclass can implement this differently, it is possible for one application to support autorotation with some of its views but not with others, or for one view controller to support certain orientations under certain conditions.
Code Sense in Action
Have you noticed that the defined system constants on the iPhone are always designed so that values that work together start with the same letters? One reason why UIInterfaceOrientationPortrait,
UIInterfaceOrientationPortraitUpsideDown, UIInterfaceOrientationLandscapeLeft, and UIInterfaceOrientationLandscapeRight all begin with UIInterfaceOrientation is to let you take advantage of Xcode’s Code Sense feature.
You’ve probably noticed that as you type, Xcode frequently tries to complete the word you are typing. That’s Code Sense in action.
www.it-ebooks.info
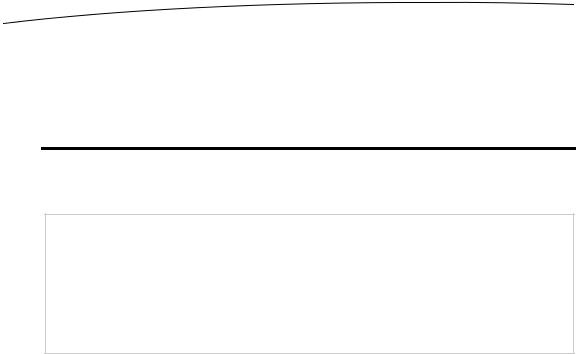
118 |
CHAPTER 5: Autorotation and Autosizing |
Developers cannot possibly remember all the various defined constants in the system, but you can remember the common beginning for the groups you use frequently. When you need to specify an orientation, simply type UIInterfaceOrientation (or even UIInterf), and then press the escape key to bring up a list of all matches. (In Xcode’s preferences, you can change that matching key from escape to something else.) You can use the arrow keys to navigate the list that appears and make a selection by pressing the tab or return key. This is much faster than needing to look up the values in the documentation or header files.
Once again, the template has predicted what we would need, so we can leave this code untouched for now. However, feel free to play around with this method by returning YES or NO for different orientations.
NOTE: iOS actually has two different types of orientations. The one we’re discussing here is the interface orientation. There’s also a separate but related concept of device orientation. Device orientation specifies how the device is currently being held. Interface orientation is which way the stuff on the screen is rotated. If you turn a standard iPhone app upside down, the device
orientation will be upside down, but the interface orientation will be one of the other three, since
iPhone apps typically don’t support portrait upside down.
Designing an Interface with Autosize Attributes
In Xcode, select BIDViewController.xib to edit the file in Interface Builder. One nice thing about using autosize attributes is that they require very little code. We do need to specify which orientations we support in code, but the rest of the autoresize implementation can be done right here in Interface Builder.
To see how this works, drag six Round Rect Buttons from the library over to your view, and place them as shown in Figure 5–3. Double-click each button, and assign a title to each one so you can tell them apart later. We’ve used UL for the upper-left button, UR for the upper-right button, L for the middle-left button, R for the middle-right button, LL for the lower-left button, and LR for the lower-right button.
www.it-ebooks.info
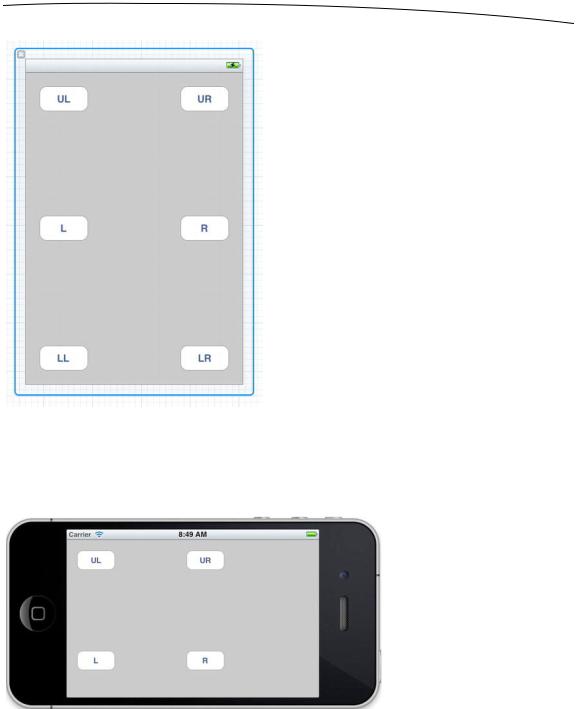
CHAPTER 5: Autorotation and Autosizing |
119 |
Figure 5–3. Adding six labeled buttons to the interface
Let’s see what happens now that we’ve specified that we support autorotation but haven’t set any autosize attributes. Build and run the app. Once the iPhone simulator comes up, select Hardware Rotate Left, which will simulate turning the iPhone to landscape mode. Take a look at Figure 5–4. Oh, dear.
Figure 5–4. Well, that’s not very useful, is it? Where are buttons LL and LR?
Most controls default to a setting that has them stay where they are in relation to the left side and top of the screen. There are some exceptions to that rule, but it’s usually true.
www.it-ebooks.info