
- •Contents at a Glance
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Preface
- •What This Book Is
- •What You Need
- •Developer Options
- •What You Need to Know
- •What’s Different About Coding for iOS?
- •Only One Active Application
- •Only One Window
- •Limited Access
- •Limited Response Time
- •Limited Screen Size
- •Limited System Resources
- •No Garbage Collection, but…
- •Some New Stuff
- •A Different Approach
- •What’s in This Book
- •What’s New in This Update?
- •Are You Ready?
- •Setting Up Your Project in Xcode
- •The Xcode Workspace Window
- •The Toolbar
- •The Navigator View
- •The Jump Bar
- •The Utility Pane
- •Interface Builder
- •New Compiler and Debugger
- •A Closer Look at Our Project
- •Introducing Xcode’s Interface Builder
- •What’s in the Nib File?
- •The Library
- •Adding a Label to the View
- •Changing Attributes
- •Some iPhone Polish—Finishing Touches
- •Bring It on Home
- •The Model-View-Controller Paradigm
- •Creating Our Project
- •Looking at the View Controller
- •Understanding Outlets and Actions
- •Outlets
- •Actions
- •Cleaning Up the View Controller
- •Designing the User Interface
- •Adding the Buttons and Action Method
- •Adding the Label and Outlet
- •Writing the Action Method
- •Trying It Out
- •Looking at the Application Delegate
- •Bring It on Home
- •A Screen Full of Controls
- •Active, Static, and Passive Controls
- •Creating the Application
- •Implementing the Image View and Text Fields
- •Adding the Image View
- •Resizing the Image View
- •Setting View Attributes
- •The Mode Attribute
- •Interaction Checkboxes
- •The Alpha Value
- •Background
- •Drawing Checkboxes
- •Stretching
- •Adding the Text Fields
- •Text Field Inspector Settings
- •Setting the Attributes for the Second Text Field
- •Creating and Connecting Outlets
- •Closing the Keyboard
- •Closing the Keyboard When Done Is Tapped
- •Touching the Background to Close the Keyboard
- •Adding the Slider and Label
- •Creating and Connecting the Actions and Outlets
- •Implementing the Action Method
- •Adding Two Labeled Switches
- •Connecting and Creating Outlets and Actions
- •Implementing the Switch Actions
- •Adding the Button
- •Connecting and Creating the Button Outlets and Actions
- •Implementing the Segmented Control Action
- •Implementing the Action Sheet and Alert
- •Conforming to the Action Sheet Delegate Method
- •Showing the Action Sheet
- •Spiffing Up the Button
- •Using the viewDidLoad Method
- •Control States
- •Stretchable Images
- •Crossing the Finish Line
- •The Mechanics of Autorotation
- •Points, Pixels, and the Retina Display
- •Autorotation Approaches
- •Handling Rotation Using Autosize Attributes
- •Configuring Supported Orientations
- •Specifying Rotation Support
- •Designing an Interface with Autosize Attributes
- •Using the Size Inspector’s Autosize Attributes
- •Setting the Buttons’ Autosize Attributes
- •Restructuring a View When Rotated
- •Creating and Connecting Outlets
- •Moving the Buttons on Rotation
- •Swapping Views
- •Designing the Two Views
- •Implementing the Swap
- •Changing Outlet Collections
- •Rotating Out of Here
- •Common Types of Multiview Apps
- •The Architecture of a Multiview Application
- •The Root Controller
- •Anatomy of a Content View
- •Building View Switcher
- •Creating Our View Controller and Nib Files
- •Modifying the App Delegate
- •Modifying BIDSwitchViewController.h
- •Adding a View Controller
- •Building a View with a Toolbar
- •Writing the Root View Controller
- •Implementing the Content Views
- •Animating the Transition
- •Switching Off
- •The Pickers Application
- •Delegates and Data Sources
- •Setting Up the Tab Bar Framework
- •Creating the Files
- •Adding the Root View Controller
- •Creating TabBarController.xib
- •The Initial Test Run
- •Implementing the Date Picker
- •Implementing the Single-Component Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller As a Data Source and Delegate
- •Implementing a Multicomponent Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller
- •Implementing Dependent Components
- •Creating a Simple Game with a Custom Picker
- •Writing the Controller Header File
- •Building the View
- •Adding Image Resources
- •Implementing the Controller
- •The spin Method
- •The viewDidLoad Method
- •Final Details
- •Linking in the Audio Toolbox Framework
- •Final Spin
- •Table View Basics
- •Table Views and Table View Cells
- •Grouped and Plain Tables
- •Implementing a Simple Table
- •Designing the View
- •Writing the Controller
- •Adding an Image
- •Using Table View Cell Styles
- •Setting the Indent Level
- •Handling Row Selection
- •Changing the Font Size and Row Height
- •Customizing Table View Cells
- •Adding Subviews to the Table View Cell
- •Creating a UITableViewCell Subclass
- •Adding New Cells
- •Implementing the Controller’s Code
- •Loading a UITableViewCell from a Nib
- •Designing the Table View Cell in Interface Builder
- •Using the New Table View Cell
- •Grouped and Indexed Sections
- •Building the View
- •Importing the Data
- •Implementing the Controller
- •Adding an Index
- •Implementing a Search Bar
- •Rethinking the Design
- •A Deep Mutable Copy
- •Updating the Controller Header File
- •Modifying the View
- •Modifying the Controller Implementation
- •Copying Data from allNames
- •Implementing the Search
- •Changes to viewDidLoad
- •Changes to Data Source Methods
- •Adding a Table View Delegate Method
- •Adding Search Bar Delegate Methods
- •Adding a Magnifying Glass to the Index
- •Adding the Special Value to the Keys Array
- •Suppressing the Section Header
- •Telling the Table View What to Do
- •Putting It All on the Table
- •Navigation Controller Basics
- •Stacky Goodness
- •A Stack of Controllers
- •Nav, a Hierarchical Application in Six Parts
- •Meet the Subcontrollers
- •The Disclosure Button View
- •The Checklist View
- •The Rows Control View
- •The Movable Rows View
- •The Deletable Rows View
- •The Editable Detail View
- •The Nav Application’s Skeleton
- •Creating the Top-Level View Controller
- •Setting Up the Navigation Controller
- •Adding the Images to the Project
- •First Subcontroller: The Disclosure Button View
- •Creating the Detail View
- •Modifying the Disclosure Button Controller
- •Adding a Disclosure Button Controller Instance
- •Second Subcontroller: The Checklist
- •Creating the Checklist View
- •Adding a Checklist Controller Instance
- •Third Subcontroller: Controls on Table Rows
- •Creating the Row Controls View
- •Adding a Rows Control Controller Instance
- •Fourth Subcontroller: Movable Rows
- •Creating the Movable Row View
- •Adding a Move Me Controller Instance
- •Fifth Subcontroller: Deletable Rows
- •Creating the Deletable Rows View
- •Adding a Delete Me Controller Instance
- •Sixth Subcontroller: An Editable Detail Pane
- •Creating the Data Model Object
- •Creating the Detail View List Controller
- •Creating the Detail View Controller
- •Adding an Editable Detail View Controller Instance
- •But There’s One More Thing. . .
- •Breaking the Tape
- •Creating a Simple Storyboard
- •Dynamic Prototype Cells
- •Dynamic Table Content, Storyboard-Style
- •Editing Prototype Cells
- •Good Old Table View Data Source
- •Will It Load?
- •Static Cells
- •Going Static
- •So Long, Good Old Table View Data Source
- •You Say Segue, I Say Segue
- •Creating Segue Navigator
- •Filling the Blank Slate
- •First Transition
- •A Slightly More Useful Task List
- •Viewing Task Details
- •Make More Segues, Please
- •Passing a Task from the List
- •Handling Task Details
- •Passing Back Details
- •Making the List Receive the Details
- •If Only We Could End with a Smooth Transition
- •Split Views and Popovers
- •Creating a SplitView Project
- •The Storyboard Defines the Structure
- •The Code Defines the Functionality
- •The App Delegate
- •The Master View Controller
- •The Detail View Controller
- •Here Come the Presidents
- •Creating Your Own Popover
- •iPad Wrap-Up
- •Getting to Know Your Settings Bundle
- •The AppSettings Application
- •Creating the Project
- •Working with the Settings Bundle
- •Adding a Settings Bundle to Our Project
- •Setting Up the Property List
- •Adding a Text Field Setting
- •Adding an Application Icon
- •Adding a Secure Text Field Setting
- •Adding a Multivalue Field
- •Adding a Toggle Switch Setting
- •Adding the Slider Setting
- •Adding Icons to the Settings Bundle
- •Adding a Child Settings View
- •Reading Settings in Our Application
- •Retrieving User Settings
- •Creating the Main View
- •Updating the Main View Controller
- •Registering Default Values
- •Changing Defaults from Our Application
- •Keeping It Real
- •Beam Me Up, Scotty
- •Your Application’s Sandbox
- •Getting the Documents Directory
- •Getting the tmp Directory
- •File-Saving Strategies
- •Single-File Persistence
- •Multiple-File Persistence
- •Using Property Lists
- •Property List Serialization
- •The First Version of the Persistence Application
- •Creating the Persistence Project
- •Designing the Persistence Application View
- •Editing the Persistence Classes
- •Archiving Model Objects
- •Conforming to NSCoding
- •Implementing NSCopying
- •Archiving and Unarchiving Data Objects
- •The Archiving Application
- •Implementing the BIDFourLines Class
- •Implementing the BIDViewController Class
- •Using iOS’s Embedded SQLite3
- •Creating or Opening the Database
- •Using Bind Variables
- •The SQLite3 Application
- •Linking to the SQLite3 Library
- •Modifying the Persistence View Controller
- •Using Core Data
- •Entities and Managed Objects
- •Key-Value Coding
- •Putting It All in Context
- •Creating New Managed Objects
- •Retrieving Managed Objects
- •The Core Data Application
- •Designing the Data Model
- •Creating the Persistence View and Controller
- •Persistence Rewarded
- •Managing Document Storage with UIDocument
- •Building TinyPix
- •Creating BIDTinyPixDocument
- •Code Master
- •Initial Storyboarding
- •Creating BIDTinyPixView
- •Storyboard Detailing
- •Adding iCloud Support
- •Creating a Provisioning Profile
- •Enabling iCloud Entitlements
- •How to Query
- •Save Where?
- •Storing Preferences on iCloud
- •What We Didn’t Cover
- •Grand Central Dispatch
- •Introducing SlowWorker
- •Threading Basics
- •Units of Work
- •GCD: Low-Level Queueing
- •Becoming a Blockhead
- •Improving SlowWorker
- •Don’t Forget That Main Thread
- •Giving Some Feedback
- •Concurrent Blocks
- •Background Processing
- •Application Life Cycle
- •State-Change Notifications
- •Creating State Lab
- •Exploring Execution States
- •Making Use of Execution State Changes
- •Handling the Inactive State
- •Handling the Background State
- •Removing Resources When Entering the Background
- •Saving State When Entering the Background
- •A Brief Journey to Yesteryear
- •Back to the Background
- •Requesting More Backgrounding Time
- •Grand Central Dispatch, Over and Out
- •Two Views of a Graphical World
- •The Quartz 2D Approach to Drawing
- •Quartz 2D’s Graphics Contexts
- •The Coordinate System
- •Specifying Colors
- •A Bit of Color Theory for Your iOS Device’s Display
- •Other Color Models
- •Color Convenience Methods
- •Drawing Images in Context
- •Drawing Shapes: Polygons, Lines, and Curves
- •The QuartzFun Application
- •Setting Up the QuartzFun Application
- •Creating a Random Color
- •Defining Application Constants
- •Implementing the QuartzFunView Skeleton
- •Creating and Connecting Outlets and Actions
- •Implementing the Action Methods
- •Adding Quartz 2D Drawing Code
- •Drawing the Line
- •Drawing the Rectangle and Ellipse
- •Drawing the Image
- •Optimizing the QuartzFun Application
- •The GLFun Application
- •Setting Up the GLFun Application
- •Creating BIDGLFunView
- •Updating BIDViewController
- •Updating the Nib
- •Finishing GLFun
- •Drawing to a Close
- •Multitouch Terminology
- •The Responder Chain
- •Responding to Events
- •Forwarding an Event: Keeping the Responder Chain Alive
- •The Multitouch Architecture
- •The Four Touch Notification Methods
- •The TouchExplorer Application
- •The Swipes Application
- •Automatic Gesture Recognition
- •Implementing Multiple Swipes
- •Detecting Multiple Taps
- •Detecting Pinches
- •Defining Custom Gestures
- •The CheckPlease Application
- •The CheckPlease Touch Methods
- •Garçon? Check, Please!
- •The Location Manager
- •Setting the Desired Accuracy
- •Setting the Distance Filter
- •Starting the Location Manager
- •Using the Location Manager Wisely
- •The Location Manager Delegate
- •Getting Location Updates
- •Getting Latitude and Longitude Using CLLocation
- •Error Notifications
- •Trying Out Core Location
- •Updating Location Manager
- •Determining Distance Traveled
- •Wherever You Go, There You Are
- •Accelerometer Physics
- •Don’t Forget Rotation
- •Core Motion and the Motion Manager
- •Event-Based Motion
- •Proactive Motion Access
- •Accelerometer Results
- •Detecting Shakes
- •Baked-In Shaking
- •Shake and Break
- •Accelerometer As Directional Controller
- •Rolling Marbles
- •Writing the Ball View
- •Calculating Ball Movement
- •Rolling On
- •Using the Image Picker and UIImagePickerController
- •Implementing the Image Picker Controller Delegate
- •Road Testing the Camera and Library
- •Designing the Interface
- •Implementing the Camera View Controller
- •It’s a Snap!
- •Localization Architecture
- •Strings Files
- •What’s in a Strings File?
- •The Localized String Macro
- •Real-World iOS: Localizing Your Application
- •Setting Up LocalizeMe
- •Trying Out LocalizeMe
- •Localizing the Nib
- •Localizing an Image
- •Generating and Localizing a Strings File
- •Localizing the App Display Name
- •Auf Wiedersehen
- •Apple’s Documentation
- •Mailing Lists
- •Discussion Forums
- •Web Sites
- •Blogs
- •Conferences
- •Follow the Authors
- •Farewell
- •Index

656 |
CHAPTER 19: Whee! Gyro and Accelerometer! |
phone is being held upright in portrait orientation. A positive value for y indicates some force is being exerted in the opposite direction, which could mean the phone is being held upside down or that the phone is being moved in a downward direction.
Keeping the diagram in Figure 19–1 in mind, let’s look at some accelerometer results (see Figure 19–3). Note that, in real life, you will almost never get values this precise, as the accelerometer is sensitive enough to sense even tiny amounts of motion, and you will usually pick up at least some tiny amount of force on all three axes. This is realworld physics, not high-school physics.
Figure 19–3. Idealized acceleration values for different device orientations
Probably the most common usage of the accelerometer in third-party applications is as a controller for games. We’ll create a program that uses the accelerometer for input a little later in the chapter, but first, we’ll look at another common accelerometer use: detecting shakes.
Detecting Shakes
Like a gesture, a shake can be used as a form of input to your application. For example, the drawing program GLPaint, which is one of the iOS sample code projects, lets users erase drawings by shaking their iOS device, sort of like an Etch A Sketch.
Detecting shakes is relatively trivial. All it requires is checking for an absolute value on one of the axes that is greater than a set threshold. During normal usage, it’s not uncommon for one of the three axes to register values up to around 1.3 g, but getting values much higher than that generally requires intentional force. The accelerometer seems to be unable to register values higher than around 2.3 g (at least in our experience), so you don’t want to set your threshold any higher than that.
To detect a shake, you could check for an absolute value greater than 1.5 for a slight shake and 2.0 for a strong shake, like this:
- (void)accelerometer:(UIAccelerometer *)accelerometer didAccelerate:(UIAcceleration *)acceleration {
if (fabsf(acceleration.x) > 2.0
www.it-ebooks.info

CHAPTER 19: Whee! Gyro and Accelerometer! |
657 |
||fabsf(acceleration.y) > 2.0
||fabsf(acceleration.z) > 2.0) { // Do something here...
}
}
This method would detect any movement on any axis that exceeded two g-forces.
You could implement more sophisticated shake detection by requiring the user to shake back and forth a certain number of times to register as a shake, like so:
- (void)accelerometer:(UIAccelerometer *)accelerometer didAccelerate:(UIAcceleration *)acceleration {
static NSInteger shakeCount = 0; static NSDate *shakeStart;
NSDate *now = [[NSDate alloc] init];
NSDate *checkDate = [[NSDate alloc] initWithTimeInterval:1.5f sinceDate:shakeStart];
if ([now compare:checkDate] == NSOrderedDescending || shakeStart == nil) {
shakeCount = 0;
shakeStart = [[NSDate alloc] init];
}
if (fabsf(acceleration.x) > 2.0
||fabsf(acceleration.y) > 2.0
||fabsf(acceleration.z) > 2.0) { shakeCount++;
if (shakeCount > 4) {
// Do something shakeCount = 0;
shakeStart = [[NSDate alloc] init];
}
}
}
This method keeps track of the number of times the accelerometer reports a value above 2.0, and if it happens four times within a 1.5-second span of time, it registers as a shake.
Baked-In Shaking
There’s actually another way to check for shakes—one that’s baked right into the responder chain. Remember back in Chapter 17 how we implemented methods like touchesBegan:withEvent: to detect touches? Well, iOS also provides three similar responder methods for detecting motion:
When motion begins, the motionBegan:withEvent: method is sent to the first responder and then on through the responder chain, as discussed in Chapter 17.
When the motion ends, the motionEnded:withEvent: method is sent to the first responder.
www.it-ebooks.info
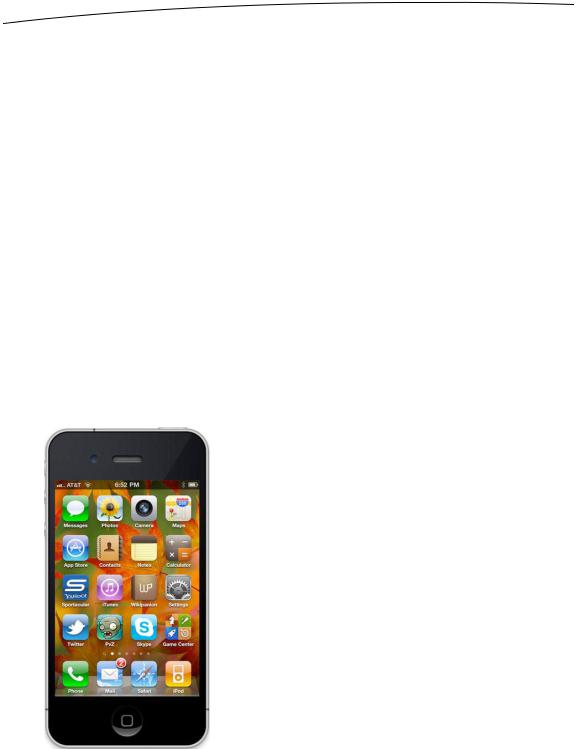
658CHAPTER 19: Whee! Gyro and Accelerometer!
If the phone rings, or some other interrupting action happens during the shake, the motionCancelled:withEvent: message is sent to the first responder.
This means that you can actually detect a shake without using CMMotionManager directly. All you need to do is override the appropriate motion-sensing methods in your view or view controller, and they will be called automatically when the user shakes the phone. Unless you specifically need more control over the shake gesture, you should use the baked-in motion detection rather than the manual method described previously, but we thought we would show you the manual method in case you ever do need more control.
Now that you have the basic idea of how to detect shakes, we’re going to break your phone.
Shake and Break
OK, we’re not really going to break your phone, but we’ll write an application that detects shakes, and then makes your phone look and sound like it broke as a result of the shake.
When you launch the application, the program will display a picture that looks like the iPhone home screen (see Figure 19–4). Shake the phone hard enough, though, and your poor phone will make a sound that you never want to hear coming out of a consumer electronics device. What’s more, your screen will look like the one shown in Figure 19–5. Why do we do these evil things? Not to worry. You can reset the iPhone to its previously pristine state by touching the screen.
Figure 19–4. The ShakeAndBreak application looks innocuous enough…
www.it-ebooks.info
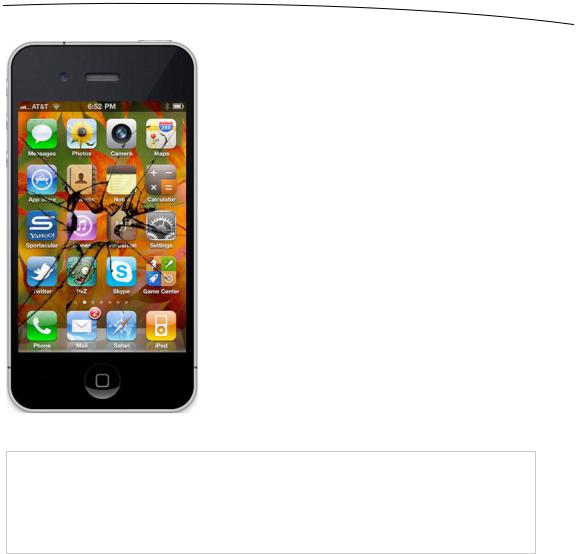
CHAPTER 19: Whee! Gyro and Accelerometer! |
659 |
Figure 19–5. . . . but handle it too roughly and—oh no!
NOTE: Just for completeness, we’ve included a modified version of ShakeAndBreak in the project archives based on the built-in shake-detection method. You’ll find it in the project archive in a folder named 19 - ShakeAndBreak - Motion Methods. The magic is in the
BIDViewController‘s motionEnded:withEvent: method.
Create a new project in Xcode using the Single View Application template. Call the new project ShakeAndBreak. In the 19 - ShakeAndBreak folder of the project archive, we’ve provided the two images and the sound file you need for this application. Drag home.png, homebroken.png, and glass.wav to your project. There’s also an icon.png file in that folder. Add that to the project as well.
Next, expand the Supporting Files folder, and select ShakeAndBreak-Info.plist to bring up the property list editor. We need to add an entry to the property list to tell our application not to use a status bar. Start off by right-clicking (or control-clicking) anywhere in the property list editor, and selecting the Show Raw Keys/Values option from the context menu so you can see the real names of the configurations we’re setting. Single-click any row in the property list, and press enter to add a new row. Change the new row’s Key to UIStatusBarHidden. The Value of this row will default to NO. Change it to YES. Finally,
www.it-ebooks.info
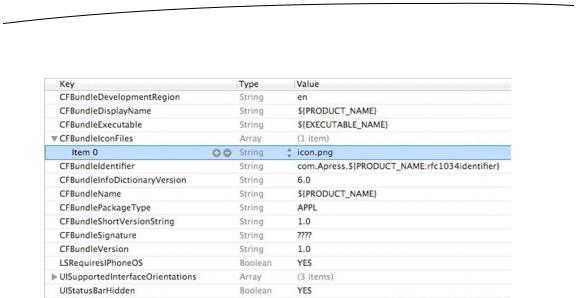
660 |
CHAPTER 19: Whee! Gyro and Accelerometer! |
expand the array entry named CFBundleIconFiles, and press enter to add a new String item. Type icon.png in the Value column (see Figure 19–6).
Figure 19–6. Changing the value for CFBundleIconFiles (shown highlighted) and for UIStatusBarHidden (last line in the property list)
Now, let’s start creating our view controller. We’re going to need to create an outlet to point to an image view so that we can change the displayed image. We’ll also need a couple of UIImage instances to hold the two pictures, a sound ID to refer to the sound, and a Boolean to keep track of whether the screen needs to be reset. Single-click BIDViewController.h, and add the following code:
#import <UIKit/UIKit.h>
#import <CoreMotion/CoreMotion.h> #import <AudioToolbox/AudioToolbox.h>
#define |
kAccelerationThreshold |
1.7 |
#define |
kUpdateInterval |
(1.0f / 10.0f) |
@interface BIDViewController : UIViewController
<UIAccelerometerDelegate>
@property (weak, nonatomic) IBOutlet UIImageView *imageView; @property (strong, nonatomic) CMMotionManager *motionManager; @property (assign, nonatomic) BOOL brokenScreenShowing; @property (assign, nonatomic) SystemSoundID soundID; @property (strong, nonatomic) UIImage *fixed;
@property (strong, nonatomic) UIImage *broken;
@end
Save the header file. Now, select BIDViewController.xib to edit the file in Interface Builder. Click the View icon to select the view, and then bring up the attributes inspector and change the Status Bar popup under Simulated Metrics from Gray to None. Next, drag an Image View over from the library to the view in the layout area. The image view should automatically resize to take up the full window, so just place it so that it sits perfectly within the window.
www.it-ebooks.info

CHAPTER 19: Whee! Gyro and Accelerometer! |
661 |
Control-drag from the File’s Owner icon to the image view, and select the imageView outlet. Then save the nib file.
Next, select the BIDViewController.m file, and add the following code near the top of the file:
#import "BIDViewController.h"
@implementation BIDViewController
@synthesize imageView; @synthesize motionManager; @synthesize brokenScreenShowing; @synthesize soundID;
@synthesize fixed; @synthesize broken;
.
.
.
Give the viewDidLoad method the following implementation:
- (void) viewDidLoad { [super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
NSString *path = [[NSBundle mainBundle] pathForResource:@"glass" ofType:@"wav"];
NSURL *url = [NSURL fileURLWithPath:path]; AudioServicesCreateSystemSoundID((__bridge CFURLRef)url,
&soundID);
self.fixed = [UIImage imageNamed:@"home.png"]; self.broken = [UIImage imageNamed:@"homebroken.png"];
imageView.image = fixed;
self.motionManager = [[CMMotionManager alloc] init]; motionManager.accelerometerUpdateInterval = kUpdateInterval; NSOperationQueue *queue = [[NSOperationQueue alloc] init]; [motionManager startAccelerometerUpdatesToQueue:queue
withHandler: ^(CMAccelerometerData *accelerometerData, NSError *error){
if (error) {
[motionManager stopAccelerometerUpdates]; } else {
if (!brokenScreenShowing) {
CMAcceleration acceleration = accelerometerData.acceleration; if (acceleration.x > kAccelerationThreshold
||acceleration.y > kAccelerationThreshold
||acceleration.z > kAccelerationThreshold) {
[imageView performSelectorOnMainThread:@selector(setImage:) withObject:broken
waitUntilDone:NO];
AudioServicesPlaySystemSound(soundID); brokenScreenShowing = YES;
}
www.it-ebooks.info
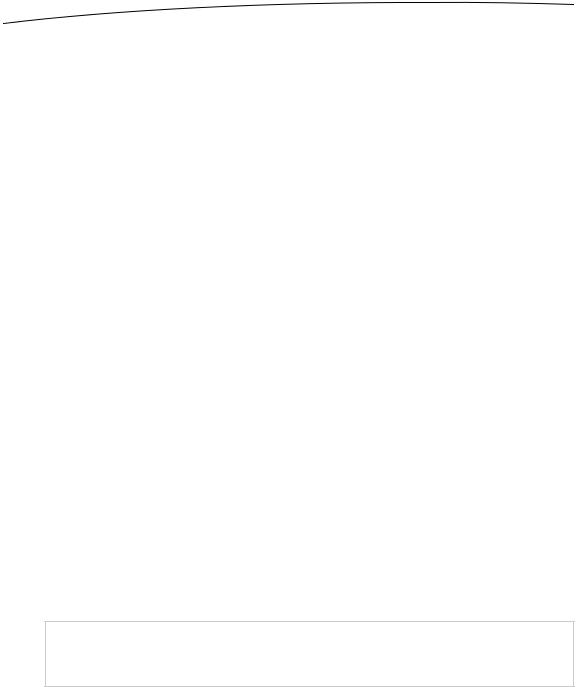
662 |
CHAPTER 19: Whee! Gyro and Accelerometer! |
}
}
}];
}
Insert the following lines of code into the existing viewDidUnload method:
- (void)viewDidUnload
{
[super viewDidUnload];
//Release any retained subviews of the main view.
//e.g. self.myOutlet = nil;
self.imageView = nil; self.motionManager = nil; self.fixed = nil; self.broken = nil;
}
And finally, add the following new method at the bottom of the file:
.
.
.
#pragma mark -
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event { imageView.image = fixed;
brokenScreenShowing = NO;
}
@end
The first method we implement, and the one where most of the interesting things happen, is viewDidLoad. First, we create an NSURL object pointing to our sound file, load it into memory, and save the assigned identifier in the soundID instance variable. In order to satisfy the requirements for ARC, we need to tell the compiler how to manage the memory for the NSURL object before passing it off to
AudioServicesCreateSystemSoundID(), which we do by casting it using the __bridge qualifier.
NSString *path = [[NSBundle mainBundle] pathForResource:@"glass" ofType:@"wav"];
NSURL *url = [NSURL fileURLWithPath:path]; AudioServicesCreateSystemSoundID((__bridge CFURLRef)url,
&soundID);
NOTE: New to the __bridge qualifier? It’s discussed in Chapter 7. In a nutshell, it is used to
safely bridge to ARC.
We then load the two images into memory.
self.fixed = [UIImage imageNamed:@"home.png"]; self.broken = [UIImage imageNamed:@"homebroken.png"];
www.it-ebooks.info

CHAPTER 19: Whee! Gyro and Accelerometer! |
663 |
Finally, we set imageView to show the unbroken screenshot and set brokenScreenShowing to NO to indicate that the screen does not currently need to be reset.
imageView.image = fixed; brokenScreenShowing = NO;
Then we create a CMMotionManager and an NSOperationQueue (just as we’ve done before), and start up the accelerometer, sending it a block to be run each time the accelerometer value changes.
self.motionManager = [[CMMotionManager alloc] init]; motionManager.accelerometerUpdateInterval = kUpdateInterval; NSOperationQueue *queue = [[NSOperationQueue alloc] init]; [motionManager startAccelerometerUpdatesToQueue:queue
withHandler:
If the block finds accelerometer values high enough to trigger the break, it makes imageView switch over to the broken image and starts playing the breaking sound. Note that imageView is a member of the UIImageView class, which, like most parts of UIKit, is meant to run only in the main thread. Since the block may be run in another thread, we force the imageView update to happen on the main thread.
^(CMAccelerometerData *accelerometerData, NSError *error){ if (error) {
[motionManager stopAccelerometerUpdates]; } else {
if (!brokenScreenShowing) {
CMAcceleration acceleration = accelerometerData.acceleration; if (acceleration.x > kAccelerationThreshold
||acceleration.y > kAccelerationThreshold
||acceleration.z > kAccelerationThreshold) {
[imageView performSelectorOnMainThread:@selector(setImage:) withObject:broken
waitUntilDone:NO];
AudioServicesPlaySystemSound(soundID); brokenScreenShowing = YES;
}
}
}
}];
The last method is one you should be quite familiar with by now. It’s called when the screen is touched. All we do in that method is to set the image back to the unbroken screen and set brokenScreenShowing back to NO.
imageView.image = fixed; brokenScreenShowing = NO;
Finally, add the CoreMotion.framework as well as the AudioToolbox.framework so that we can play the sound file. You can link the frameworks into your application by following the instructions from earlier in the chapter.
Compile and run the application, and take it for a test drive. For those of you who don’t have the ability to run this application on your iOS device, you might want to give the shake-event-based version a try. The simulator does not simulate the accelerometer
www.it-ebooks.info