
- •Contents at a Glance
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Preface
- •What This Book Is
- •What You Need
- •Developer Options
- •What You Need to Know
- •What’s Different About Coding for iOS?
- •Only One Active Application
- •Only One Window
- •Limited Access
- •Limited Response Time
- •Limited Screen Size
- •Limited System Resources
- •No Garbage Collection, but…
- •Some New Stuff
- •A Different Approach
- •What’s in This Book
- •What’s New in This Update?
- •Are You Ready?
- •Setting Up Your Project in Xcode
- •The Xcode Workspace Window
- •The Toolbar
- •The Navigator View
- •The Jump Bar
- •The Utility Pane
- •Interface Builder
- •New Compiler and Debugger
- •A Closer Look at Our Project
- •Introducing Xcode’s Interface Builder
- •What’s in the Nib File?
- •The Library
- •Adding a Label to the View
- •Changing Attributes
- •Some iPhone Polish—Finishing Touches
- •Bring It on Home
- •The Model-View-Controller Paradigm
- •Creating Our Project
- •Looking at the View Controller
- •Understanding Outlets and Actions
- •Outlets
- •Actions
- •Cleaning Up the View Controller
- •Designing the User Interface
- •Adding the Buttons and Action Method
- •Adding the Label and Outlet
- •Writing the Action Method
- •Trying It Out
- •Looking at the Application Delegate
- •Bring It on Home
- •A Screen Full of Controls
- •Active, Static, and Passive Controls
- •Creating the Application
- •Implementing the Image View and Text Fields
- •Adding the Image View
- •Resizing the Image View
- •Setting View Attributes
- •The Mode Attribute
- •Interaction Checkboxes
- •The Alpha Value
- •Background
- •Drawing Checkboxes
- •Stretching
- •Adding the Text Fields
- •Text Field Inspector Settings
- •Setting the Attributes for the Second Text Field
- •Creating and Connecting Outlets
- •Closing the Keyboard
- •Closing the Keyboard When Done Is Tapped
- •Touching the Background to Close the Keyboard
- •Adding the Slider and Label
- •Creating and Connecting the Actions and Outlets
- •Implementing the Action Method
- •Adding Two Labeled Switches
- •Connecting and Creating Outlets and Actions
- •Implementing the Switch Actions
- •Adding the Button
- •Connecting and Creating the Button Outlets and Actions
- •Implementing the Segmented Control Action
- •Implementing the Action Sheet and Alert
- •Conforming to the Action Sheet Delegate Method
- •Showing the Action Sheet
- •Spiffing Up the Button
- •Using the viewDidLoad Method
- •Control States
- •Stretchable Images
- •Crossing the Finish Line
- •The Mechanics of Autorotation
- •Points, Pixels, and the Retina Display
- •Autorotation Approaches
- •Handling Rotation Using Autosize Attributes
- •Configuring Supported Orientations
- •Specifying Rotation Support
- •Designing an Interface with Autosize Attributes
- •Using the Size Inspector’s Autosize Attributes
- •Setting the Buttons’ Autosize Attributes
- •Restructuring a View When Rotated
- •Creating and Connecting Outlets
- •Moving the Buttons on Rotation
- •Swapping Views
- •Designing the Two Views
- •Implementing the Swap
- •Changing Outlet Collections
- •Rotating Out of Here
- •Common Types of Multiview Apps
- •The Architecture of a Multiview Application
- •The Root Controller
- •Anatomy of a Content View
- •Building View Switcher
- •Creating Our View Controller and Nib Files
- •Modifying the App Delegate
- •Modifying BIDSwitchViewController.h
- •Adding a View Controller
- •Building a View with a Toolbar
- •Writing the Root View Controller
- •Implementing the Content Views
- •Animating the Transition
- •Switching Off
- •The Pickers Application
- •Delegates and Data Sources
- •Setting Up the Tab Bar Framework
- •Creating the Files
- •Adding the Root View Controller
- •Creating TabBarController.xib
- •The Initial Test Run
- •Implementing the Date Picker
- •Implementing the Single-Component Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller As a Data Source and Delegate
- •Implementing a Multicomponent Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller
- •Implementing Dependent Components
- •Creating a Simple Game with a Custom Picker
- •Writing the Controller Header File
- •Building the View
- •Adding Image Resources
- •Implementing the Controller
- •The spin Method
- •The viewDidLoad Method
- •Final Details
- •Linking in the Audio Toolbox Framework
- •Final Spin
- •Table View Basics
- •Table Views and Table View Cells
- •Grouped and Plain Tables
- •Implementing a Simple Table
- •Designing the View
- •Writing the Controller
- •Adding an Image
- •Using Table View Cell Styles
- •Setting the Indent Level
- •Handling Row Selection
- •Changing the Font Size and Row Height
- •Customizing Table View Cells
- •Adding Subviews to the Table View Cell
- •Creating a UITableViewCell Subclass
- •Adding New Cells
- •Implementing the Controller’s Code
- •Loading a UITableViewCell from a Nib
- •Designing the Table View Cell in Interface Builder
- •Using the New Table View Cell
- •Grouped and Indexed Sections
- •Building the View
- •Importing the Data
- •Implementing the Controller
- •Adding an Index
- •Implementing a Search Bar
- •Rethinking the Design
- •A Deep Mutable Copy
- •Updating the Controller Header File
- •Modifying the View
- •Modifying the Controller Implementation
- •Copying Data from allNames
- •Implementing the Search
- •Changes to viewDidLoad
- •Changes to Data Source Methods
- •Adding a Table View Delegate Method
- •Adding Search Bar Delegate Methods
- •Adding a Magnifying Glass to the Index
- •Adding the Special Value to the Keys Array
- •Suppressing the Section Header
- •Telling the Table View What to Do
- •Putting It All on the Table
- •Navigation Controller Basics
- •Stacky Goodness
- •A Stack of Controllers
- •Nav, a Hierarchical Application in Six Parts
- •Meet the Subcontrollers
- •The Disclosure Button View
- •The Checklist View
- •The Rows Control View
- •The Movable Rows View
- •The Deletable Rows View
- •The Editable Detail View
- •The Nav Application’s Skeleton
- •Creating the Top-Level View Controller
- •Setting Up the Navigation Controller
- •Adding the Images to the Project
- •First Subcontroller: The Disclosure Button View
- •Creating the Detail View
- •Modifying the Disclosure Button Controller
- •Adding a Disclosure Button Controller Instance
- •Second Subcontroller: The Checklist
- •Creating the Checklist View
- •Adding a Checklist Controller Instance
- •Third Subcontroller: Controls on Table Rows
- •Creating the Row Controls View
- •Adding a Rows Control Controller Instance
- •Fourth Subcontroller: Movable Rows
- •Creating the Movable Row View
- •Adding a Move Me Controller Instance
- •Fifth Subcontroller: Deletable Rows
- •Creating the Deletable Rows View
- •Adding a Delete Me Controller Instance
- •Sixth Subcontroller: An Editable Detail Pane
- •Creating the Data Model Object
- •Creating the Detail View List Controller
- •Creating the Detail View Controller
- •Adding an Editable Detail View Controller Instance
- •But There’s One More Thing. . .
- •Breaking the Tape
- •Creating a Simple Storyboard
- •Dynamic Prototype Cells
- •Dynamic Table Content, Storyboard-Style
- •Editing Prototype Cells
- •Good Old Table View Data Source
- •Will It Load?
- •Static Cells
- •Going Static
- •So Long, Good Old Table View Data Source
- •You Say Segue, I Say Segue
- •Creating Segue Navigator
- •Filling the Blank Slate
- •First Transition
- •A Slightly More Useful Task List
- •Viewing Task Details
- •Make More Segues, Please
- •Passing a Task from the List
- •Handling Task Details
- •Passing Back Details
- •Making the List Receive the Details
- •If Only We Could End with a Smooth Transition
- •Split Views and Popovers
- •Creating a SplitView Project
- •The Storyboard Defines the Structure
- •The Code Defines the Functionality
- •The App Delegate
- •The Master View Controller
- •The Detail View Controller
- •Here Come the Presidents
- •Creating Your Own Popover
- •iPad Wrap-Up
- •Getting to Know Your Settings Bundle
- •The AppSettings Application
- •Creating the Project
- •Working with the Settings Bundle
- •Adding a Settings Bundle to Our Project
- •Setting Up the Property List
- •Adding a Text Field Setting
- •Adding an Application Icon
- •Adding a Secure Text Field Setting
- •Adding a Multivalue Field
- •Adding a Toggle Switch Setting
- •Adding the Slider Setting
- •Adding Icons to the Settings Bundle
- •Adding a Child Settings View
- •Reading Settings in Our Application
- •Retrieving User Settings
- •Creating the Main View
- •Updating the Main View Controller
- •Registering Default Values
- •Changing Defaults from Our Application
- •Keeping It Real
- •Beam Me Up, Scotty
- •Your Application’s Sandbox
- •Getting the Documents Directory
- •Getting the tmp Directory
- •File-Saving Strategies
- •Single-File Persistence
- •Multiple-File Persistence
- •Using Property Lists
- •Property List Serialization
- •The First Version of the Persistence Application
- •Creating the Persistence Project
- •Designing the Persistence Application View
- •Editing the Persistence Classes
- •Archiving Model Objects
- •Conforming to NSCoding
- •Implementing NSCopying
- •Archiving and Unarchiving Data Objects
- •The Archiving Application
- •Implementing the BIDFourLines Class
- •Implementing the BIDViewController Class
- •Using iOS’s Embedded SQLite3
- •Creating or Opening the Database
- •Using Bind Variables
- •The SQLite3 Application
- •Linking to the SQLite3 Library
- •Modifying the Persistence View Controller
- •Using Core Data
- •Entities and Managed Objects
- •Key-Value Coding
- •Putting It All in Context
- •Creating New Managed Objects
- •Retrieving Managed Objects
- •The Core Data Application
- •Designing the Data Model
- •Creating the Persistence View and Controller
- •Persistence Rewarded
- •Managing Document Storage with UIDocument
- •Building TinyPix
- •Creating BIDTinyPixDocument
- •Code Master
- •Initial Storyboarding
- •Creating BIDTinyPixView
- •Storyboard Detailing
- •Adding iCloud Support
- •Creating a Provisioning Profile
- •Enabling iCloud Entitlements
- •How to Query
- •Save Where?
- •Storing Preferences on iCloud
- •What We Didn’t Cover
- •Grand Central Dispatch
- •Introducing SlowWorker
- •Threading Basics
- •Units of Work
- •GCD: Low-Level Queueing
- •Becoming a Blockhead
- •Improving SlowWorker
- •Don’t Forget That Main Thread
- •Giving Some Feedback
- •Concurrent Blocks
- •Background Processing
- •Application Life Cycle
- •State-Change Notifications
- •Creating State Lab
- •Exploring Execution States
- •Making Use of Execution State Changes
- •Handling the Inactive State
- •Handling the Background State
- •Removing Resources When Entering the Background
- •Saving State When Entering the Background
- •A Brief Journey to Yesteryear
- •Back to the Background
- •Requesting More Backgrounding Time
- •Grand Central Dispatch, Over and Out
- •Two Views of a Graphical World
- •The Quartz 2D Approach to Drawing
- •Quartz 2D’s Graphics Contexts
- •The Coordinate System
- •Specifying Colors
- •A Bit of Color Theory for Your iOS Device’s Display
- •Other Color Models
- •Color Convenience Methods
- •Drawing Images in Context
- •Drawing Shapes: Polygons, Lines, and Curves
- •The QuartzFun Application
- •Setting Up the QuartzFun Application
- •Creating a Random Color
- •Defining Application Constants
- •Implementing the QuartzFunView Skeleton
- •Creating and Connecting Outlets and Actions
- •Implementing the Action Methods
- •Adding Quartz 2D Drawing Code
- •Drawing the Line
- •Drawing the Rectangle and Ellipse
- •Drawing the Image
- •Optimizing the QuartzFun Application
- •The GLFun Application
- •Setting Up the GLFun Application
- •Creating BIDGLFunView
- •Updating BIDViewController
- •Updating the Nib
- •Finishing GLFun
- •Drawing to a Close
- •Multitouch Terminology
- •The Responder Chain
- •Responding to Events
- •Forwarding an Event: Keeping the Responder Chain Alive
- •The Multitouch Architecture
- •The Four Touch Notification Methods
- •The TouchExplorer Application
- •The Swipes Application
- •Automatic Gesture Recognition
- •Implementing Multiple Swipes
- •Detecting Multiple Taps
- •Detecting Pinches
- •Defining Custom Gestures
- •The CheckPlease Application
- •The CheckPlease Touch Methods
- •Garçon? Check, Please!
- •The Location Manager
- •Setting the Desired Accuracy
- •Setting the Distance Filter
- •Starting the Location Manager
- •Using the Location Manager Wisely
- •The Location Manager Delegate
- •Getting Location Updates
- •Getting Latitude and Longitude Using CLLocation
- •Error Notifications
- •Trying Out Core Location
- •Updating Location Manager
- •Determining Distance Traveled
- •Wherever You Go, There You Are
- •Accelerometer Physics
- •Don’t Forget Rotation
- •Core Motion and the Motion Manager
- •Event-Based Motion
- •Proactive Motion Access
- •Accelerometer Results
- •Detecting Shakes
- •Baked-In Shaking
- •Shake and Break
- •Accelerometer As Directional Controller
- •Rolling Marbles
- •Writing the Ball View
- •Calculating Ball Movement
- •Rolling On
- •Using the Image Picker and UIImagePickerController
- •Implementing the Image Picker Controller Delegate
- •Road Testing the Camera and Library
- •Designing the Interface
- •Implementing the Camera View Controller
- •It’s a Snap!
- •Localization Architecture
- •Strings Files
- •What’s in a Strings File?
- •The Localized String Macro
- •Real-World iOS: Localizing Your Application
- •Setting Up LocalizeMe
- •Trying Out LocalizeMe
- •Localizing the Nib
- •Localizing an Image
- •Generating and Localizing a Strings File
- •Localizing the App Display Name
- •Auf Wiedersehen
- •Apple’s Documentation
- •Mailing Lists
- •Discussion Forums
- •Web Sites
- •Blogs
- •Conferences
- •Follow the Authors
- •Farewell
- •Index

CHAPTER 8: Introduction to Table Views |
237 |
Creating a UITableViewCell Subclass
Up until this point, the standard table view cells we’ve been using have taken care of all the details of cell layout for us. Our controller code has been kept clear of the messy details about where to place labels and images, and has been able to just pass off the display values to the cell. This keeps presentation logic out of the controller, and that’s a really good design to stick to. For this project, we’re going to make a new cell subclass of our own that takes care of the details for the new layout, which will keep our controller as simple as possible.
Adding New Cells
Select the Cells folder in the project navigator, and press N to create a new file. In the assistant that pops up, choose Objective-C class from the Cocoa Touch section, and click Next. On the following screen, enter BIDNameAndColorCell as the name of the new class, select UITableViewCell in the Subclass of popup list, and click Next again. On the final screen, select the Cells folder that already contains your other source code, make sure Cells is chosen both in the Group and Target controls at the bottom, and click Create.
Select BIDNameAndColorCell.h, and add the following code:
#import <UIKit/UIKit.h>
@interface BIDNameAndColorCell : UITableViewCell
@property (copy, nonatomic) NSString *name; @property (copy, nonatomic) NSString *color;
@end
Here, we’ve defined two properties that our controller will use to pass values to each cell. Note that instead of declaring the NSString properties with strong semantics, we’re using copy. Doing so with NSString values is always a good idea, because there’s a risk that the string value passed in to a property setter may actually be an NSMutableString, which the sender can modify later on, leading to problems. Copying each string that’s passed in to a property gives us a stable, unchangeable snapshot of what the string contains at the moment the setter is called.
That’s all we need to expose in the header, so let’s move on to
BIDNameAndColorCell.m. Add the following code at the top of the file:
#import "BIDNameAndColorCell.h"
#define |
kNameValueTag |
1 |
#define |
kColorValueTag |
2 |
@implementation BIDNameAndColorCell
@synthesize name; @synthesize color;
.
.
.
www.it-ebooks.info

238 |
CHAPTER 8: Introduction to Table Views |
Notice that we’ve defined two constants. We’re going to use these to assign tags to some of the subviews that we’ll be adding to the table view cell. We’ll add four subviews to the cell, and two of those need to be changed for every row. In order to do that, we need some mechanism that will allow us to retrieve the two fields from the cell when we go to update that cell with a particular row’s data. If we set unique tag values for each label that we’ll use again, we’ll be able to retrieve them from the table view cell and set their values. We also declared the name and color properties, which the controller will use to set the values that should appear in the cell.
Now, edit the existing initWithStyle:reuseIdentifier: method to create the views that we’ll need to display.
- (id)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier
{
self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]; if (self) {
// Initialization code
CGRect nameLabelRect = CGRectMake(0, 5, 70, 15);
UILabel *nameLabel = [[UILabel alloc] initWithFrame:nameLabelRect]; nameLabel.textAlignment = UITextAlignmentRight;
nameLabel.text = @"Name:";
nameLabel.font = [UIFont boldSystemFontOfSize:12]; [self.contentView addSubview: nameLabel];
CGRect colorLabelRect = CGRectMake(0, 26, 70, 15);
UILabel *colorLabel = [[UILabel alloc] initWithFrame:colorLabelRect]; colorLabel.textAlignment = UITextAlignmentRight;
colorLabel.text = @"Color:";
colorLabel.font = [UIFont boldSystemFontOfSize:12]; [self.contentView addSubview: colorLabel];
CGRect nameValueRect = CGRectMake(80, 5, 200, 15); UILabel *nameValue = [[UILabel alloc] initWithFrame:
nameValueRect]; nameValue.tag = kNameValueTag; [self.contentView addSubview:nameValue];
CGRect colorValueRect = CGRectMake(80, 25, 200, 15); UILabel *colorValue = [[UILabel alloc] initWithFrame:
colorValueRect]; colorValue.tag = kColorValueTag; [self.contentView addSubview:colorValue];
}
return self;
}
That should be pretty straightforward. We create four UILabels and add them to the table view cell. The table view cell already has a UIView subview called contentView, which it uses to group all of its subviews, much the way we grouped those two switches inside a UIView back in Chapter 4. As a result, we don’t add the labels as subviews directly to the table view cell, but rather to its contentView.
[self.contentView addSubview:colorValue];
www.it-ebooks.info

CHAPTER 8: Introduction to Table Views |
239 |
Two of these labels contain static text. The label nameLabel contains the text Name:, and the label colorLabel contains the text Color:. Those are just labels that we won’t change. But we’ll use the other two labels to display our row-specific data. Remember that we need some way of retrieving these fields later on, so we assign values to both of them. For example, we assign the constant kNameValueTag to nameValue‘s tag field.
nameValue.tag = kNameValueTag;
Now, to put the finishing touches on the BIDNameAndColorCell class, add these two setter methods just before the @end:
- (void)setName:(NSString *)n {
if (![n isEqualToString:name]) { name = [n copy];
UILabel *nameLabel = (UILabel *)[self.contentView viewWithTag: kNameValueTag];
nameLabel.text = name;
}
}
- (void)setColor:(NSString *)c {
if (![c isEqualToString:color]) { color = [c copy];
UILabel *colorLabel = (UILabel *)[self.contentView viewWithTag: kColorValueTag];
colorLabel.text = color;
}
}
You already know that using @synthesize, as we did at the top of the file, creates getter and setter methods for each property. Yet, here we’re defining our own setters for both name and color! As it turns out, this is just fine. Any time a class defines its own getters or setters, those will be used instead of the default methods that @synthesize provides. In this class, we’re using the default, synthesized getters, but defining our own setters, so that whenever we are passed new values for the name or color properties, we update the labels we created earlier.
Implementing the Controller’s Code
Now, let’s set up the simple controller to display values in our nice new cells. Start off by selecting BIDViewController.h, where you need to add the following code:
#import <UIKit/UIKit.h>
@interface BIDViewController : UIViewController
<UITableViewDataSource, UITableViewDelegate>
@property (strong, nonatomic) NSArray *computers;
@end
In our controller, we need to set up some data to use, and then implement the table data source methods to feed that data to the table. Switch to BIDViewController.m, and add the following code at the beginning of the file:
www.it-ebooks.info

240CHAPTER 8: Introduction to Table Views
#import "BIDViewController.h"
#import "BIDNameAndColorCell.h"
@implementation ViewController
@synthesize computers;
.
.
.
- (void)viewDidLoad { [super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
NSDictionary *row1 = [[NSDictionary alloc] initWithObjectsAndKeys: @"MacBook", @"Name", @"White", @"Color", nil];
NSDictionary *row2 = [[NSDictionary alloc] initWithObjectsAndKeys: @"MacBook Pro", @"Name", @"Silver", @"Color", nil];
NSDictionary *row3 = [[NSDictionary alloc] initWithObjectsAndKeys: @"iMac", @"Name", @"Silver", @"Color", nil];
NSDictionary *row4 = [[NSDictionary alloc] initWithObjectsAndKeys: @"Mac Mini", @"Name", @"Silver", @"Color", nil]; NSDictionary *row5 = [[NSDictionary alloc] initWithObjectsAndKeys:
@"Mac Pro", @"Name", @"Silver", @"Color", nil];
self.computers = [[NSArray alloc] initWithObjects:row1, row2, row3, row4, row5, nil];
}
.
.
.
Of course, we need to be good memory citizens, so make the following changes to the existing viewDidUnload method:
- (void)viewDidUnload { [super viewDidUnload];
//Release any retained subviews of the main view.
//e.g. self.myOutlet = nil;
self.computers = nil;
}
Then add this code at the end of the file, above the @end declaration:
.
.
.
#pragma mark -
#pragma mark Table Data Source Methods
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { return [self.computers count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
static NSString *CellTableIdentifier = @"CellTableIdentifier";
BIDNameAndColorCell *cell = [tableView dequeueReusableCellWithIdentifier:
www.it-ebooks.info
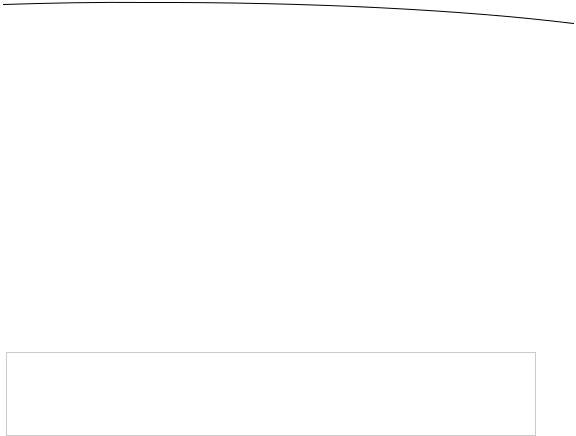
CHAPTER 8: Introduction to Table Views |
241 |
CellTableIdentifier];
if (cell == nil) {
cell = [[BIDNameAndColorCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellTableIdentifier];
}
NSUInteger row = [indexPath row];
NSDictionary *rowData = [self.computers objectAtIndex:row];
cell.name = [rowData objectForKey:@"Name"]; cell.color = [rowData objectForKey:@"Color"];
return cell;
}
@end
This version of viewDidLoad creates a series of dictionaries. Each dictionary contains the name and color information for one row in the table. The name for that row is held in the dictionary under the key Name, and the color is held under the key Color. We stick all the dictionaries into a single array, which is our data for this table.
NOTE: Remember when Macs came in different colors, like beige, platinum, black, and white? And that’s not to mention the original iMac and MacBook series, with their beautiful rainbow
hues assortment. Now there’s just one color: silver. Harrumph.
Let’s focus on tableView:cellForRowWithIndexPath:, since that’s where we’re really getting into some new stuff. The first two lines of code are nearly identical to our earlier versions. We create an identifier and ask the table to dequeue a table view cell if it has one. The only difference here is that we declare the cell variable to be an instance of our BIDNameAndColorCell class instead of the standard UITableViewCell. That’s so we can access the properties that we added specifically to our table view cell subclass.
If the table doesn’t have any cells available for reuse, we need to create a new cell. This is essentially the same technique as before, except that here we’re also using our custom class instead of UITableViewCell. We specify the default style, although the style actually doesn’t matter, because we’ll be adding our own subviews to display our data rather than using the provided ones.
cell = [[BIDNameAndColorCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellTableIdentifier];
Once we’re finished creating our new cell, we use the indexPath argument that was passed in to determine which row the table is requesting a cell for, and then use that row value to grab the correct dictionary for the requested row. Remember that the dictionary has two key/value pairs: one with name and another with color.
NSUInteger row = [indexPath row];
NSDictionary *rowData = [self.computers objectAtIndex:row];
www.it-ebooks.info