
- •Contents at a Glance
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Preface
- •What This Book Is
- •What You Need
- •Developer Options
- •What You Need to Know
- •What’s Different About Coding for iOS?
- •Only One Active Application
- •Only One Window
- •Limited Access
- •Limited Response Time
- •Limited Screen Size
- •Limited System Resources
- •No Garbage Collection, but…
- •Some New Stuff
- •A Different Approach
- •What’s in This Book
- •What’s New in This Update?
- •Are You Ready?
- •Setting Up Your Project in Xcode
- •The Xcode Workspace Window
- •The Toolbar
- •The Navigator View
- •The Jump Bar
- •The Utility Pane
- •Interface Builder
- •New Compiler and Debugger
- •A Closer Look at Our Project
- •Introducing Xcode’s Interface Builder
- •What’s in the Nib File?
- •The Library
- •Adding a Label to the View
- •Changing Attributes
- •Some iPhone Polish—Finishing Touches
- •Bring It on Home
- •The Model-View-Controller Paradigm
- •Creating Our Project
- •Looking at the View Controller
- •Understanding Outlets and Actions
- •Outlets
- •Actions
- •Cleaning Up the View Controller
- •Designing the User Interface
- •Adding the Buttons and Action Method
- •Adding the Label and Outlet
- •Writing the Action Method
- •Trying It Out
- •Looking at the Application Delegate
- •Bring It on Home
- •A Screen Full of Controls
- •Active, Static, and Passive Controls
- •Creating the Application
- •Implementing the Image View and Text Fields
- •Adding the Image View
- •Resizing the Image View
- •Setting View Attributes
- •The Mode Attribute
- •Interaction Checkboxes
- •The Alpha Value
- •Background
- •Drawing Checkboxes
- •Stretching
- •Adding the Text Fields
- •Text Field Inspector Settings
- •Setting the Attributes for the Second Text Field
- •Creating and Connecting Outlets
- •Closing the Keyboard
- •Closing the Keyboard When Done Is Tapped
- •Touching the Background to Close the Keyboard
- •Adding the Slider and Label
- •Creating and Connecting the Actions and Outlets
- •Implementing the Action Method
- •Adding Two Labeled Switches
- •Connecting and Creating Outlets and Actions
- •Implementing the Switch Actions
- •Adding the Button
- •Connecting and Creating the Button Outlets and Actions
- •Implementing the Segmented Control Action
- •Implementing the Action Sheet and Alert
- •Conforming to the Action Sheet Delegate Method
- •Showing the Action Sheet
- •Spiffing Up the Button
- •Using the viewDidLoad Method
- •Control States
- •Stretchable Images
- •Crossing the Finish Line
- •The Mechanics of Autorotation
- •Points, Pixels, and the Retina Display
- •Autorotation Approaches
- •Handling Rotation Using Autosize Attributes
- •Configuring Supported Orientations
- •Specifying Rotation Support
- •Designing an Interface with Autosize Attributes
- •Using the Size Inspector’s Autosize Attributes
- •Setting the Buttons’ Autosize Attributes
- •Restructuring a View When Rotated
- •Creating and Connecting Outlets
- •Moving the Buttons on Rotation
- •Swapping Views
- •Designing the Two Views
- •Implementing the Swap
- •Changing Outlet Collections
- •Rotating Out of Here
- •Common Types of Multiview Apps
- •The Architecture of a Multiview Application
- •The Root Controller
- •Anatomy of a Content View
- •Building View Switcher
- •Creating Our View Controller and Nib Files
- •Modifying the App Delegate
- •Modifying BIDSwitchViewController.h
- •Adding a View Controller
- •Building a View with a Toolbar
- •Writing the Root View Controller
- •Implementing the Content Views
- •Animating the Transition
- •Switching Off
- •The Pickers Application
- •Delegates and Data Sources
- •Setting Up the Tab Bar Framework
- •Creating the Files
- •Adding the Root View Controller
- •Creating TabBarController.xib
- •The Initial Test Run
- •Implementing the Date Picker
- •Implementing the Single-Component Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller As a Data Source and Delegate
- •Implementing a Multicomponent Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller
- •Implementing Dependent Components
- •Creating a Simple Game with a Custom Picker
- •Writing the Controller Header File
- •Building the View
- •Adding Image Resources
- •Implementing the Controller
- •The spin Method
- •The viewDidLoad Method
- •Final Details
- •Linking in the Audio Toolbox Framework
- •Final Spin
- •Table View Basics
- •Table Views and Table View Cells
- •Grouped and Plain Tables
- •Implementing a Simple Table
- •Designing the View
- •Writing the Controller
- •Adding an Image
- •Using Table View Cell Styles
- •Setting the Indent Level
- •Handling Row Selection
- •Changing the Font Size and Row Height
- •Customizing Table View Cells
- •Adding Subviews to the Table View Cell
- •Creating a UITableViewCell Subclass
- •Adding New Cells
- •Implementing the Controller’s Code
- •Loading a UITableViewCell from a Nib
- •Designing the Table View Cell in Interface Builder
- •Using the New Table View Cell
- •Grouped and Indexed Sections
- •Building the View
- •Importing the Data
- •Implementing the Controller
- •Adding an Index
- •Implementing a Search Bar
- •Rethinking the Design
- •A Deep Mutable Copy
- •Updating the Controller Header File
- •Modifying the View
- •Modifying the Controller Implementation
- •Copying Data from allNames
- •Implementing the Search
- •Changes to viewDidLoad
- •Changes to Data Source Methods
- •Adding a Table View Delegate Method
- •Adding Search Bar Delegate Methods
- •Adding a Magnifying Glass to the Index
- •Adding the Special Value to the Keys Array
- •Suppressing the Section Header
- •Telling the Table View What to Do
- •Putting It All on the Table
- •Navigation Controller Basics
- •Stacky Goodness
- •A Stack of Controllers
- •Nav, a Hierarchical Application in Six Parts
- •Meet the Subcontrollers
- •The Disclosure Button View
- •The Checklist View
- •The Rows Control View
- •The Movable Rows View
- •The Deletable Rows View
- •The Editable Detail View
- •The Nav Application’s Skeleton
- •Creating the Top-Level View Controller
- •Setting Up the Navigation Controller
- •Adding the Images to the Project
- •First Subcontroller: The Disclosure Button View
- •Creating the Detail View
- •Modifying the Disclosure Button Controller
- •Adding a Disclosure Button Controller Instance
- •Second Subcontroller: The Checklist
- •Creating the Checklist View
- •Adding a Checklist Controller Instance
- •Third Subcontroller: Controls on Table Rows
- •Creating the Row Controls View
- •Adding a Rows Control Controller Instance
- •Fourth Subcontroller: Movable Rows
- •Creating the Movable Row View
- •Adding a Move Me Controller Instance
- •Fifth Subcontroller: Deletable Rows
- •Creating the Deletable Rows View
- •Adding a Delete Me Controller Instance
- •Sixth Subcontroller: An Editable Detail Pane
- •Creating the Data Model Object
- •Creating the Detail View List Controller
- •Creating the Detail View Controller
- •Adding an Editable Detail View Controller Instance
- •But There’s One More Thing. . .
- •Breaking the Tape
- •Creating a Simple Storyboard
- •Dynamic Prototype Cells
- •Dynamic Table Content, Storyboard-Style
- •Editing Prototype Cells
- •Good Old Table View Data Source
- •Will It Load?
- •Static Cells
- •Going Static
- •So Long, Good Old Table View Data Source
- •You Say Segue, I Say Segue
- •Creating Segue Navigator
- •Filling the Blank Slate
- •First Transition
- •A Slightly More Useful Task List
- •Viewing Task Details
- •Make More Segues, Please
- •Passing a Task from the List
- •Handling Task Details
- •Passing Back Details
- •Making the List Receive the Details
- •If Only We Could End with a Smooth Transition
- •Split Views and Popovers
- •Creating a SplitView Project
- •The Storyboard Defines the Structure
- •The Code Defines the Functionality
- •The App Delegate
- •The Master View Controller
- •The Detail View Controller
- •Here Come the Presidents
- •Creating Your Own Popover
- •iPad Wrap-Up
- •Getting to Know Your Settings Bundle
- •The AppSettings Application
- •Creating the Project
- •Working with the Settings Bundle
- •Adding a Settings Bundle to Our Project
- •Setting Up the Property List
- •Adding a Text Field Setting
- •Adding an Application Icon
- •Adding a Secure Text Field Setting
- •Adding a Multivalue Field
- •Adding a Toggle Switch Setting
- •Adding the Slider Setting
- •Adding Icons to the Settings Bundle
- •Adding a Child Settings View
- •Reading Settings in Our Application
- •Retrieving User Settings
- •Creating the Main View
- •Updating the Main View Controller
- •Registering Default Values
- •Changing Defaults from Our Application
- •Keeping It Real
- •Beam Me Up, Scotty
- •Your Application’s Sandbox
- •Getting the Documents Directory
- •Getting the tmp Directory
- •File-Saving Strategies
- •Single-File Persistence
- •Multiple-File Persistence
- •Using Property Lists
- •Property List Serialization
- •The First Version of the Persistence Application
- •Creating the Persistence Project
- •Designing the Persistence Application View
- •Editing the Persistence Classes
- •Archiving Model Objects
- •Conforming to NSCoding
- •Implementing NSCopying
- •Archiving and Unarchiving Data Objects
- •The Archiving Application
- •Implementing the BIDFourLines Class
- •Implementing the BIDViewController Class
- •Using iOS’s Embedded SQLite3
- •Creating or Opening the Database
- •Using Bind Variables
- •The SQLite3 Application
- •Linking to the SQLite3 Library
- •Modifying the Persistence View Controller
- •Using Core Data
- •Entities and Managed Objects
- •Key-Value Coding
- •Putting It All in Context
- •Creating New Managed Objects
- •Retrieving Managed Objects
- •The Core Data Application
- •Designing the Data Model
- •Creating the Persistence View and Controller
- •Persistence Rewarded
- •Managing Document Storage with UIDocument
- •Building TinyPix
- •Creating BIDTinyPixDocument
- •Code Master
- •Initial Storyboarding
- •Creating BIDTinyPixView
- •Storyboard Detailing
- •Adding iCloud Support
- •Creating a Provisioning Profile
- •Enabling iCloud Entitlements
- •How to Query
- •Save Where?
- •Storing Preferences on iCloud
- •What We Didn’t Cover
- •Grand Central Dispatch
- •Introducing SlowWorker
- •Threading Basics
- •Units of Work
- •GCD: Low-Level Queueing
- •Becoming a Blockhead
- •Improving SlowWorker
- •Don’t Forget That Main Thread
- •Giving Some Feedback
- •Concurrent Blocks
- •Background Processing
- •Application Life Cycle
- •State-Change Notifications
- •Creating State Lab
- •Exploring Execution States
- •Making Use of Execution State Changes
- •Handling the Inactive State
- •Handling the Background State
- •Removing Resources When Entering the Background
- •Saving State When Entering the Background
- •A Brief Journey to Yesteryear
- •Back to the Background
- •Requesting More Backgrounding Time
- •Grand Central Dispatch, Over and Out
- •Two Views of a Graphical World
- •The Quartz 2D Approach to Drawing
- •Quartz 2D’s Graphics Contexts
- •The Coordinate System
- •Specifying Colors
- •A Bit of Color Theory for Your iOS Device’s Display
- •Other Color Models
- •Color Convenience Methods
- •Drawing Images in Context
- •Drawing Shapes: Polygons, Lines, and Curves
- •The QuartzFun Application
- •Setting Up the QuartzFun Application
- •Creating a Random Color
- •Defining Application Constants
- •Implementing the QuartzFunView Skeleton
- •Creating and Connecting Outlets and Actions
- •Implementing the Action Methods
- •Adding Quartz 2D Drawing Code
- •Drawing the Line
- •Drawing the Rectangle and Ellipse
- •Drawing the Image
- •Optimizing the QuartzFun Application
- •The GLFun Application
- •Setting Up the GLFun Application
- •Creating BIDGLFunView
- •Updating BIDViewController
- •Updating the Nib
- •Finishing GLFun
- •Drawing to a Close
- •Multitouch Terminology
- •The Responder Chain
- •Responding to Events
- •Forwarding an Event: Keeping the Responder Chain Alive
- •The Multitouch Architecture
- •The Four Touch Notification Methods
- •The TouchExplorer Application
- •The Swipes Application
- •Automatic Gesture Recognition
- •Implementing Multiple Swipes
- •Detecting Multiple Taps
- •Detecting Pinches
- •Defining Custom Gestures
- •The CheckPlease Application
- •The CheckPlease Touch Methods
- •Garçon? Check, Please!
- •The Location Manager
- •Setting the Desired Accuracy
- •Setting the Distance Filter
- •Starting the Location Manager
- •Using the Location Manager Wisely
- •The Location Manager Delegate
- •Getting Location Updates
- •Getting Latitude and Longitude Using CLLocation
- •Error Notifications
- •Trying Out Core Location
- •Updating Location Manager
- •Determining Distance Traveled
- •Wherever You Go, There You Are
- •Accelerometer Physics
- •Don’t Forget Rotation
- •Core Motion and the Motion Manager
- •Event-Based Motion
- •Proactive Motion Access
- •Accelerometer Results
- •Detecting Shakes
- •Baked-In Shaking
- •Shake and Break
- •Accelerometer As Directional Controller
- •Rolling Marbles
- •Writing the Ball View
- •Calculating Ball Movement
- •Rolling On
- •Using the Image Picker and UIImagePickerController
- •Implementing the Image Picker Controller Delegate
- •Road Testing the Camera and Library
- •Designing the Interface
- •Implementing the Camera View Controller
- •It’s a Snap!
- •Localization Architecture
- •Strings Files
- •What’s in a Strings File?
- •The Localized String Macro
- •Real-World iOS: Localizing Your Application
- •Setting Up LocalizeMe
- •Trying Out LocalizeMe
- •Localizing the Nib
- •Localizing an Image
- •Generating and Localizing a Strings File
- •Localizing the App Display Name
- •Auf Wiedersehen
- •Apple’s Documentation
- •Mailing Lists
- •Discussion Forums
- •Web Sites
- •Blogs
- •Conferences
- •Follow the Authors
- •Farewell
- •Index

210 |
CHAPTER 7: Tab Bars and Pickers |
forComponent:(NSInteger)component reusingView:(UIView *)view {
This method returns one of the image views from one of the five arrays. To do that, we once again create an NSString with the name of one of the arrays. Because component is zero-indexed, we add one to it, which gives us a value between column1 and column5 and which will correspond to the component for which the picker is requesting data.
NSString *arrayName = [[NSString alloc] initWithFormat:@"column%d", component+1];
Once we have the name of the array to use, we retrieve that array using a method called valueForKey:, which is the counterpart to the setValue:forKey: method that we used in viewDidLoad. Using it is the same as calling the accessor method for the property you specify. So, calling valueForKey: and specifying "column1" is the same as using the column1 accessor method. Once we have the correct array for the component, we just return the image view from the array that corresponds to the selected row.
NSArray *array = [self valueForKey:arrayName]; return [array objectAtIndex:row];
}
Wow, take a deep breath. You got through all of it in one piece, and now you get to take it for a spin.
Final Details
Our game is rather fun, especially when you think about how little effort it took to build it. Now let’s improve it with a couple more tweaks. There are two things about this game right now that really bug us:
It’s so darn quiet. Slot machines aren’t quiet!
It tells us that we’ve won before the dials have finished spinning, which is a minor thing, but it does tend to eliminate the anticipation. To see this in action, run your application again. It is subtle, but the label really does appear before the wheels finish spinning.
The 07 Pickers/Custom Picker Sounds folder in the project archive that accompanies the book contains two sound files: crunch.wav and win.wav. Add this folder to your project’s Pickers folder. These are the sounds we’ll play when the users tap the Spin button and when they win, respectively.
To work with sounds, we’ll need access to the iOS Audio Toolbox classes. Insert this line at the top of BIDCustomPickerViewController.m:
#import <AudioToolbox/AudioToolbox.h>
Next, we need to add an outlet that will point to the button. While the wheels are spinning, we’re going to hide the button. We don’t want users tapping the button again until the current spin is all done. Add the following code to
BIDCustomPickerViewController.h:
www.it-ebooks.info

CHAPTER 7: Tab Bars and Pickers |
211 |
#import <UIKit/UIKit.h>
@interface BIDCustomPickerViewController : UIViewController <UIPickerViewDataSource, UIPickerViewDelegate>
@property (strong, nonatomic) IBOutlet UIPickerView *picker; @property (strong, nonatomic) IBOutlet UILabel *winLabel; @property (strong, nonatomic) NSArray *column1;
@property (strong, nonatomic) NSArray *column2; @property (strong, nonatomic) NSArray *column3; @property (strong, nonatomic) NSArray *column4; @property (strong, nonatomic) NSArray *column5;
@property (strong, nonatomic) IBOutlet UIButton *button;
- (IBAction)spin;
@end
After you type that and save the file, click BIDCustomPickerViewController.xib to edit the nib. Once it’s open, control-drag from File’s Owner to the Spin button, and connect it to the new button outlet we just created. Save the nib.
Now, we need to do a few things in the implementation of our controller class. First, we need to synthesize the accessor and mutator for our new outlet. Open
BIDCustomPickerViewController.m and add the following line:
@implementation BIDCustomPickerViewController @synthesize picker;
@synthesize winLabel; @synthesize column1; @synthesize column2; @synthesize column3; @synthesize column4; @synthesize column5;
@synthesize button;
.
.
.
We also need a couple of methods added to our controller class. Add the following two methods to BIDCustomPickerViewController.m as the first two methods in the class:
-(void)showButton { self.button.hidden = NO;
}
-(void)playWinSound {
NSURL *soundURL = [[NSBundle mainBundle] URLForResource:@"win" withExtension:@"wav"];
SystemSoundID soundID;
AudioServicesCreateSystemSoundID((__bridge CFURLRef)soundURL, &soundID); AudioServicesPlaySystemSound(soundID);
winLabel.text = @"WINNING!";
[self performSelector:@selector(showButton) withObject:nil afterDelay:1.5];
}
www.it-ebooks.info
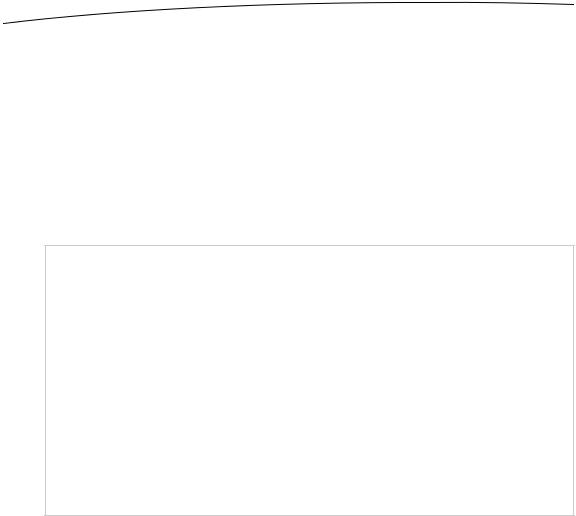
212 |
CHAPTER 7: Tab Bars and Pickers |
The first method is used to show the button. As noted previously, we’re going to hide the button when the user taps it, because if the wheels are already spinning, there’s no point in letting them spin again until they’ve stopped.
The second method will be called when the user wins. The first line of this method asks the main bundle for the path to the sound called win.wav, just as we did when we loaded the property list for the Dependent picker view. Once we have the path to that resource, the next three lines of code load the sound file in and play it. Then we set the label to WINNING! and call the showButton method, but we call the showButton method in a special way using a method called performSelector:withObject:afterDelay:. This is a very handy method available to all objects. It lets you call the method some time in the future—in this case, one and a half seconds in the future—which will give the dials time to spin to their final locations before telling the user the result.
NOTE: You may have noticed something a bit odd about the way we called the AudioServicesCreateSystemSoundID function. That function takes a URL as its first parameter, but it doesn’t want an instance of NSURL. Instead, it wants a CFURLRef structure. Apple provides C interfaces to many common components—such as URLs, arrays, strings, and much more—via the Core Foundation framework. This allows even applications written entirely in C some access to the functionality that we normally use from Objective-C. The interesting thing is that these C components are “bridged” to their Objective-C counterparts, so that a CFURLRef is functionally equivalent to an NSURL pointer, for example. That means that certain kinds of objects created in Objective-C can be pushed over the bridge to use C APIs, and vice versa. This is accomplished by using a C language cast, putting the type you want your variable to be interpreted as inside parentheses before the variable name. Starting in iOS 5, with the use of ARC, the type name itself must be preceded by the keyword __bridge, which gives ARC a hint about how it should handle this Objective-C object as it passes into a C API call.
We also need to make some changes to the spin: method. We will write code to play a sound and to call the playerWon method if the player won. Make the following changes to the spin: method now:
- (IBAction)spin { BOOL win = NO; int numInRow = 1; int lastVal = -1;
for (int i = 0; i < 5; i++) {
int newValue = random() % [self.column1 count];
if (newValue == lastVal) numInRow++;
else
numInRow = 1;
lastVal = newValue;
[picker selectRow:newValue inComponent:i animated:YES]; [picker reloadComponent:i];
www.it-ebooks.info

CHAPTER 7: Tab Bars and Pickers |
213 |
if (numInRow >= 3) win = YES;
}
self.button.hidden = YES;
NSString *path = [[NSBundle mainBundle] pathForResource:@"crunch" ofType:@"wav"];
SystemSoundID soundID; AudioServicesCreateSystemSoundID(
(__bridge CFURLRef)[NSURL fileURLWithPath:path], &soundID); AudioServicesPlaySystemSound (soundID);
if (win)
[self performSelector:@selector(playWinSound) withObject:nil
afterDelay:.5];
else
[self performSelector:@selector(showButton) withObject:nil
afterDelay:.5];
winLabel.text = @"";
if (win)
winLabel.text = @"WIN!";
else
winLabel.text = @"";
}
The first line of code we added hides the Spin button. The next four lines play a sound to let the player know they’ve spun the wheels. Then, instead of setting the label to WIN! as soon as we know the user has won, we do something tricky. We call one of the two methods we just created, but we do it after a delay using performSelector:afterDelay:. If the user won, we call our playerWon method half a second into the future, which will give time for the dials to spin into place; otherwise, we just wait a half a second and reenable the Spin button.
The only thing left to do is to release our button outlet, so make the following changes to your viewDidUnload method:
- (void)viewDidUnload { [super viewDidUnload];
//Release any retained subviews of the main view.
//e.g. self.myOutlet = nil;
self.picker = nil; self.winLabel = nil; self.column1 = nil; self.column2 = nil; self.column3 = nil; self.column4 = nil; self.column5 = nil; self.button = nil;
}
www.it-ebooks.info