
- •Contents at a Glance
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Preface
- •What This Book Is
- •What You Need
- •Developer Options
- •What You Need to Know
- •What’s Different About Coding for iOS?
- •Only One Active Application
- •Only One Window
- •Limited Access
- •Limited Response Time
- •Limited Screen Size
- •Limited System Resources
- •No Garbage Collection, but…
- •Some New Stuff
- •A Different Approach
- •What’s in This Book
- •What’s New in This Update?
- •Are You Ready?
- •Setting Up Your Project in Xcode
- •The Xcode Workspace Window
- •The Toolbar
- •The Navigator View
- •The Jump Bar
- •The Utility Pane
- •Interface Builder
- •New Compiler and Debugger
- •A Closer Look at Our Project
- •Introducing Xcode’s Interface Builder
- •What’s in the Nib File?
- •The Library
- •Adding a Label to the View
- •Changing Attributes
- •Some iPhone Polish—Finishing Touches
- •Bring It on Home
- •The Model-View-Controller Paradigm
- •Creating Our Project
- •Looking at the View Controller
- •Understanding Outlets and Actions
- •Outlets
- •Actions
- •Cleaning Up the View Controller
- •Designing the User Interface
- •Adding the Buttons and Action Method
- •Adding the Label and Outlet
- •Writing the Action Method
- •Trying It Out
- •Looking at the Application Delegate
- •Bring It on Home
- •A Screen Full of Controls
- •Active, Static, and Passive Controls
- •Creating the Application
- •Implementing the Image View and Text Fields
- •Adding the Image View
- •Resizing the Image View
- •Setting View Attributes
- •The Mode Attribute
- •Interaction Checkboxes
- •The Alpha Value
- •Background
- •Drawing Checkboxes
- •Stretching
- •Adding the Text Fields
- •Text Field Inspector Settings
- •Setting the Attributes for the Second Text Field
- •Creating and Connecting Outlets
- •Closing the Keyboard
- •Closing the Keyboard When Done Is Tapped
- •Touching the Background to Close the Keyboard
- •Adding the Slider and Label
- •Creating and Connecting the Actions and Outlets
- •Implementing the Action Method
- •Adding Two Labeled Switches
- •Connecting and Creating Outlets and Actions
- •Implementing the Switch Actions
- •Adding the Button
- •Connecting and Creating the Button Outlets and Actions
- •Implementing the Segmented Control Action
- •Implementing the Action Sheet and Alert
- •Conforming to the Action Sheet Delegate Method
- •Showing the Action Sheet
- •Spiffing Up the Button
- •Using the viewDidLoad Method
- •Control States
- •Stretchable Images
- •Crossing the Finish Line
- •The Mechanics of Autorotation
- •Points, Pixels, and the Retina Display
- •Autorotation Approaches
- •Handling Rotation Using Autosize Attributes
- •Configuring Supported Orientations
- •Specifying Rotation Support
- •Designing an Interface with Autosize Attributes
- •Using the Size Inspector’s Autosize Attributes
- •Setting the Buttons’ Autosize Attributes
- •Restructuring a View When Rotated
- •Creating and Connecting Outlets
- •Moving the Buttons on Rotation
- •Swapping Views
- •Designing the Two Views
- •Implementing the Swap
- •Changing Outlet Collections
- •Rotating Out of Here
- •Common Types of Multiview Apps
- •The Architecture of a Multiview Application
- •The Root Controller
- •Anatomy of a Content View
- •Building View Switcher
- •Creating Our View Controller and Nib Files
- •Modifying the App Delegate
- •Modifying BIDSwitchViewController.h
- •Adding a View Controller
- •Building a View with a Toolbar
- •Writing the Root View Controller
- •Implementing the Content Views
- •Animating the Transition
- •Switching Off
- •The Pickers Application
- •Delegates and Data Sources
- •Setting Up the Tab Bar Framework
- •Creating the Files
- •Adding the Root View Controller
- •Creating TabBarController.xib
- •The Initial Test Run
- •Implementing the Date Picker
- •Implementing the Single-Component Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller As a Data Source and Delegate
- •Implementing a Multicomponent Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller
- •Implementing Dependent Components
- •Creating a Simple Game with a Custom Picker
- •Writing the Controller Header File
- •Building the View
- •Adding Image Resources
- •Implementing the Controller
- •The spin Method
- •The viewDidLoad Method
- •Final Details
- •Linking in the Audio Toolbox Framework
- •Final Spin
- •Table View Basics
- •Table Views and Table View Cells
- •Grouped and Plain Tables
- •Implementing a Simple Table
- •Designing the View
- •Writing the Controller
- •Adding an Image
- •Using Table View Cell Styles
- •Setting the Indent Level
- •Handling Row Selection
- •Changing the Font Size and Row Height
- •Customizing Table View Cells
- •Adding Subviews to the Table View Cell
- •Creating a UITableViewCell Subclass
- •Adding New Cells
- •Implementing the Controller’s Code
- •Loading a UITableViewCell from a Nib
- •Designing the Table View Cell in Interface Builder
- •Using the New Table View Cell
- •Grouped and Indexed Sections
- •Building the View
- •Importing the Data
- •Implementing the Controller
- •Adding an Index
- •Implementing a Search Bar
- •Rethinking the Design
- •A Deep Mutable Copy
- •Updating the Controller Header File
- •Modifying the View
- •Modifying the Controller Implementation
- •Copying Data from allNames
- •Implementing the Search
- •Changes to viewDidLoad
- •Changes to Data Source Methods
- •Adding a Table View Delegate Method
- •Adding Search Bar Delegate Methods
- •Adding a Magnifying Glass to the Index
- •Adding the Special Value to the Keys Array
- •Suppressing the Section Header
- •Telling the Table View What to Do
- •Putting It All on the Table
- •Navigation Controller Basics
- •Stacky Goodness
- •A Stack of Controllers
- •Nav, a Hierarchical Application in Six Parts
- •Meet the Subcontrollers
- •The Disclosure Button View
- •The Checklist View
- •The Rows Control View
- •The Movable Rows View
- •The Deletable Rows View
- •The Editable Detail View
- •The Nav Application’s Skeleton
- •Creating the Top-Level View Controller
- •Setting Up the Navigation Controller
- •Adding the Images to the Project
- •First Subcontroller: The Disclosure Button View
- •Creating the Detail View
- •Modifying the Disclosure Button Controller
- •Adding a Disclosure Button Controller Instance
- •Second Subcontroller: The Checklist
- •Creating the Checklist View
- •Adding a Checklist Controller Instance
- •Third Subcontroller: Controls on Table Rows
- •Creating the Row Controls View
- •Adding a Rows Control Controller Instance
- •Fourth Subcontroller: Movable Rows
- •Creating the Movable Row View
- •Adding a Move Me Controller Instance
- •Fifth Subcontroller: Deletable Rows
- •Creating the Deletable Rows View
- •Adding a Delete Me Controller Instance
- •Sixth Subcontroller: An Editable Detail Pane
- •Creating the Data Model Object
- •Creating the Detail View List Controller
- •Creating the Detail View Controller
- •Adding an Editable Detail View Controller Instance
- •But There’s One More Thing. . .
- •Breaking the Tape
- •Creating a Simple Storyboard
- •Dynamic Prototype Cells
- •Dynamic Table Content, Storyboard-Style
- •Editing Prototype Cells
- •Good Old Table View Data Source
- •Will It Load?
- •Static Cells
- •Going Static
- •So Long, Good Old Table View Data Source
- •You Say Segue, I Say Segue
- •Creating Segue Navigator
- •Filling the Blank Slate
- •First Transition
- •A Slightly More Useful Task List
- •Viewing Task Details
- •Make More Segues, Please
- •Passing a Task from the List
- •Handling Task Details
- •Passing Back Details
- •Making the List Receive the Details
- •If Only We Could End with a Smooth Transition
- •Split Views and Popovers
- •Creating a SplitView Project
- •The Storyboard Defines the Structure
- •The Code Defines the Functionality
- •The App Delegate
- •The Master View Controller
- •The Detail View Controller
- •Here Come the Presidents
- •Creating Your Own Popover
- •iPad Wrap-Up
- •Getting to Know Your Settings Bundle
- •The AppSettings Application
- •Creating the Project
- •Working with the Settings Bundle
- •Adding a Settings Bundle to Our Project
- •Setting Up the Property List
- •Adding a Text Field Setting
- •Adding an Application Icon
- •Adding a Secure Text Field Setting
- •Adding a Multivalue Field
- •Adding a Toggle Switch Setting
- •Adding the Slider Setting
- •Adding Icons to the Settings Bundle
- •Adding a Child Settings View
- •Reading Settings in Our Application
- •Retrieving User Settings
- •Creating the Main View
- •Updating the Main View Controller
- •Registering Default Values
- •Changing Defaults from Our Application
- •Keeping It Real
- •Beam Me Up, Scotty
- •Your Application’s Sandbox
- •Getting the Documents Directory
- •Getting the tmp Directory
- •File-Saving Strategies
- •Single-File Persistence
- •Multiple-File Persistence
- •Using Property Lists
- •Property List Serialization
- •The First Version of the Persistence Application
- •Creating the Persistence Project
- •Designing the Persistence Application View
- •Editing the Persistence Classes
- •Archiving Model Objects
- •Conforming to NSCoding
- •Implementing NSCopying
- •Archiving and Unarchiving Data Objects
- •The Archiving Application
- •Implementing the BIDFourLines Class
- •Implementing the BIDViewController Class
- •Using iOS’s Embedded SQLite3
- •Creating or Opening the Database
- •Using Bind Variables
- •The SQLite3 Application
- •Linking to the SQLite3 Library
- •Modifying the Persistence View Controller
- •Using Core Data
- •Entities and Managed Objects
- •Key-Value Coding
- •Putting It All in Context
- •Creating New Managed Objects
- •Retrieving Managed Objects
- •The Core Data Application
- •Designing the Data Model
- •Creating the Persistence View and Controller
- •Persistence Rewarded
- •Managing Document Storage with UIDocument
- •Building TinyPix
- •Creating BIDTinyPixDocument
- •Code Master
- •Initial Storyboarding
- •Creating BIDTinyPixView
- •Storyboard Detailing
- •Adding iCloud Support
- •Creating a Provisioning Profile
- •Enabling iCloud Entitlements
- •How to Query
- •Save Where?
- •Storing Preferences on iCloud
- •What We Didn’t Cover
- •Grand Central Dispatch
- •Introducing SlowWorker
- •Threading Basics
- •Units of Work
- •GCD: Low-Level Queueing
- •Becoming a Blockhead
- •Improving SlowWorker
- •Don’t Forget That Main Thread
- •Giving Some Feedback
- •Concurrent Blocks
- •Background Processing
- •Application Life Cycle
- •State-Change Notifications
- •Creating State Lab
- •Exploring Execution States
- •Making Use of Execution State Changes
- •Handling the Inactive State
- •Handling the Background State
- •Removing Resources When Entering the Background
- •Saving State When Entering the Background
- •A Brief Journey to Yesteryear
- •Back to the Background
- •Requesting More Backgrounding Time
- •Grand Central Dispatch, Over and Out
- •Two Views of a Graphical World
- •The Quartz 2D Approach to Drawing
- •Quartz 2D’s Graphics Contexts
- •The Coordinate System
- •Specifying Colors
- •A Bit of Color Theory for Your iOS Device’s Display
- •Other Color Models
- •Color Convenience Methods
- •Drawing Images in Context
- •Drawing Shapes: Polygons, Lines, and Curves
- •The QuartzFun Application
- •Setting Up the QuartzFun Application
- •Creating a Random Color
- •Defining Application Constants
- •Implementing the QuartzFunView Skeleton
- •Creating and Connecting Outlets and Actions
- •Implementing the Action Methods
- •Adding Quartz 2D Drawing Code
- •Drawing the Line
- •Drawing the Rectangle and Ellipse
- •Drawing the Image
- •Optimizing the QuartzFun Application
- •The GLFun Application
- •Setting Up the GLFun Application
- •Creating BIDGLFunView
- •Updating BIDViewController
- •Updating the Nib
- •Finishing GLFun
- •Drawing to a Close
- •Multitouch Terminology
- •The Responder Chain
- •Responding to Events
- •Forwarding an Event: Keeping the Responder Chain Alive
- •The Multitouch Architecture
- •The Four Touch Notification Methods
- •The TouchExplorer Application
- •The Swipes Application
- •Automatic Gesture Recognition
- •Implementing Multiple Swipes
- •Detecting Multiple Taps
- •Detecting Pinches
- •Defining Custom Gestures
- •The CheckPlease Application
- •The CheckPlease Touch Methods
- •Garçon? Check, Please!
- •The Location Manager
- •Setting the Desired Accuracy
- •Setting the Distance Filter
- •Starting the Location Manager
- •Using the Location Manager Wisely
- •The Location Manager Delegate
- •Getting Location Updates
- •Getting Latitude and Longitude Using CLLocation
- •Error Notifications
- •Trying Out Core Location
- •Updating Location Manager
- •Determining Distance Traveled
- •Wherever You Go, There You Are
- •Accelerometer Physics
- •Don’t Forget Rotation
- •Core Motion and the Motion Manager
- •Event-Based Motion
- •Proactive Motion Access
- •Accelerometer Results
- •Detecting Shakes
- •Baked-In Shaking
- •Shake and Break
- •Accelerometer As Directional Controller
- •Rolling Marbles
- •Writing the Ball View
- •Calculating Ball Movement
- •Rolling On
- •Using the Image Picker and UIImagePickerController
- •Implementing the Image Picker Controller Delegate
- •Road Testing the Camera and Library
- •Designing the Interface
- •Implementing the Camera View Controller
- •It’s a Snap!
- •Localization Architecture
- •Strings Files
- •What’s in a Strings File?
- •The Localized String Macro
- •Real-World iOS: Localizing Your Application
- •Setting Up LocalizeMe
- •Trying Out LocalizeMe
- •Localizing the Nib
- •Localizing an Image
- •Generating and Localizing a Strings File
- •Localizing the App Display Name
- •Auf Wiedersehen
- •Apple’s Documentation
- •Mailing Lists
- •Discussion Forums
- •Web Sites
- •Blogs
- •Conferences
- •Follow the Authors
- •Farewell
- •Index

606 |
CHAPTER 17: Taps, Touches, and Gestures |
table view cell probably needs to look at that event to see if it contains a swipe gesture. Most table view cells don’t respond to gestures, however. If they don’t respond, the event proceeds up to the table view, and then up the rest of the responder chain until something responds to that event or it reaches the end of the line.
Forwarding an Event: Keeping the Responder Chain Alive
Let’s take a step back to that table view cell in the Mail application. We don’t know the internal details of Apple’s Mail application, but let’s assume, for the nonce, that the table view cell handles the delete swipe and only the delete swipe. That table view cell must implement the methods related to receiving touch events (discussed shortly) so that it can check to see if that event contained a swipe gesture. If the event contains a swipe, then the table view cell takes an action, and that’s that; the event goes no further.
If the event doesn’t contain a swipe gesture, the table view cell is responsible for forwarding that event manually to the next object in the responder chain. If it doesn’t do its forwarding job, the table and other objects up the chain will never get a chance to respond, and the application may not function as the user expects. That table view cell could prevent other views from recognizing a gesture.
Whenever you respond to a touch event, you need to keep in mind that your code doesn’t work in a vacuum. If an object intercepts an event that it doesn’t handle, it needs to pass it along manually, by calling the same method on the next responder. Here’s a bit of fictional code:
-(void)respondToFictionalEvent:(UIEvent *)event { if (someCondition)
[self handleEvent:event];
else
[self.nextResponder respondToFictionalEvent:event];
}
Notice how we call the same method on the next responder. That’s how to be a good responder-chain citizen. Fortunately, most of the time, methods that respond to an event also consume the event, but it’s important to know that if that’s not the case, you need to make sure the event is pushed back into the responder chain.
The Multitouch Architecture
Now that you know a little about the responder chain, let’s look at the process of handling gestures. As we’ve indicated, gestures are passed along the responder chain, embedded in events. This means that the code to handle any kind of interaction with the multitouch screen needs to be contained in an object in the responder chain. Generally, that means we can choose to either embed that code in a subclass of UIView or embed the code in a UIViewController.
So does this code belong in the view or in the view controller?
www.it-ebooks.info

CHAPTER 17: Taps, Touches, and Gestures |
607 |
If the view needs to do something to itself based on the user’s touches, the code probably belongs in the class that defines that view. For example, many control classes, such as UISwitch and UISlider, respond to touch-related events. A UISwitch might want to turn itself on or off based on a touch. The folks who created the UISwitch class embedded gesture-handling code in the class so the UISwitch can respond to a touch.
Often, however, when the gesture being processed affects more than the object being touched, the gesture code really belongs in the view’s controller class. For example, if the user makes a gesture touching one row that indicates that all rows should be deleted, the gesture should be handled by code in the view controller. The way you respond to touches and gestures in both situations is exactly the same, regardless of the class to which the code belongs.
The Four Touch Notification Methods
Four methods are used to notify a responder about touches. When the user first touches the screen, the system looks for a responder that has a method called touchesBegan:withEvent:. To find out when the user first begins a gesture or taps the screen, implement this method in your view or your view controller. Here’s an example of what that method might look like:
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event { NSUInteger numTaps = [[touches anyObject] tapCount]; NSUInteger numTouches = [touches count];
// Do something here.
}
This method, and each of the touch-related methods, is passed an NSSet instance called touches and an instance of UIEvent. You can determine the number of fingers currently pressed against the screen by getting a count of the objects in touches. Every object in touches is a UITouch event that represents one finger touching the screen. If this touch is part of a series of taps, you can find out the tap count by asking any of the UITouch objects. In the preceding example, a numTaps value of 2 tells you that the screen was tapped twice in quick succession, while a numTouches value of 2 tells you the user tapped the screen with two fingers at once. If both have a value of 2, then the user double-tapped with two fingers.
All of the objects in touches may not be relevant to the view or view controller where you’ve implemented this method. A table view cell, for example, probably doesn’t care about touches that are in other rows or that are in the navigation bar. You can get a subset of touches that has only those touches that fall within a particular view from the event, like so:
NSSet *myTouches = [event touchesForView:self.view];
Every UITouch represents a different finger, and each finger is located at a different position on the screen. You can find out the position of a specific finger using the UITouch object. It will even translate the point into the view’s local coordinate system if you ask it to, like this:
www.it-ebooks.info

608 |
CHAPTER 17: Taps, Touches, and Gestures |
CGPoint point = [touch locationInView:self];
You can get notified while the user is moving fingers across the screen by implementing touchesMoved:withEvent:. This method is called multiple times during a long drag, and each time it is called, you will get another set of touches and another event. In addition to being able to find out each finger’s current position from the UITouch objects, you can also discover the previous location of that touch, which is the finger’s position the last time either touchesMoved:withEvent: or touchesBegan:withEvent: was called.
When the user’s fingers are removed from the screen, another event, touchesEnded:withEvent:, is invoked. When this method is called, you know that the user is finished with a gesture.
There’s one final touch-related method that responders might implement. It’s called touchesCancelled:withEvent:, and it is called if the user is in the middle of a gesture when something happens to interrupt it, like the phone ringing. This is where you can do any cleanup you might need so you can start fresh with a new gesture. When this method is called, touchesEnded:withEvent: will not be called for the current gesture.
OK, enough theory—let’s see some of this in action.
The TouchExplorer Application
We’re going to build a little application that will give you a better feel for when the four touch-related responder methods are called. In Xcode, create a new project using the
Single View Application template. Enter TouchExplorer as the Product Name, and select iPhone for Device Family.
TouchExplorer will print messages to the screen, containing the touch and tap count, every time a touch-related method is called (see Figure 17–1).
www.it-ebooks.info
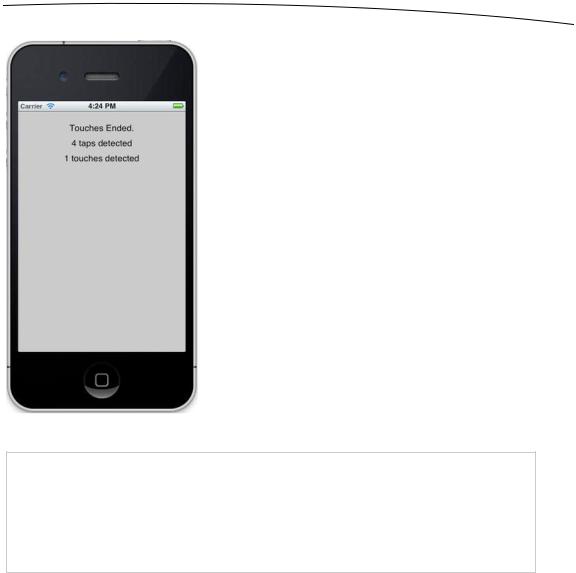
CHAPTER 17: Taps, Touches, and Gestures |
609 |
Figure 17–1. The TouchExplorer application
NOTE: Although the applications in this chapter will run on the simulator, you won’t be able to see all of the available multitouch functionality unless you run them on a real iOS device. If you’ve been accepted into the iOS Developer Program, you have the ability to run the programs you write on your device of choice. The Apple web site does a great job of walking you through
the process of getting everything you need to prepare to connect Xcode to your device.
We need three labels for this application: one to indicate which method was last called, another to report the current tap count, and a third to report the number of touches. Single-click BIDViewController.h, and add three outlets and a method declaration as follows. The method will be used to update the labels from multiple places.
#import <UIKit/UIKit.h>
@interface BIDViewController : UIViewController
@property (weak, nonatomic) IBOutlet UILabel *messageLabel; @property (weak, nonatomic) IBOutlet UILabel *tapsLabel; @property (weak, nonatomic) IBOutlet UILabel *touchesLabel; - (void)updateLabelsFromTouches:(NSSet *)touches;
@end
www.it-ebooks.info

610 |
CHAPTER 17: Taps, Touches, and Gestures |
Now, select BIDViewController.xib to edit the file. Click the View icon in the dock to edit the view if the view editor is not already open. Drag a label onto the view, using the blue guidelines to place the label toward the upper-left corner of the view. Use the resize handle to resize the label over to the right-hand blue guideline. Next, use the attribute inspector to set the label alignment to centered. Finally, hold down the option key and drag two more labels out from the original, spacing them one below the other, leaving you with three labels (see Figure 17–1).
Next, control-drag from the File’s Owner icon to each of the three labels, connecting the top one to the messageLabel outlet, the middle one to the tapsLabel outlet, and the last one to the touchesLabel outlet.
Feel free to play with the fonts and colors if you’re feeling a bit Picasso. When you’re finished placing the labels, double-click each one, and press the delete key to get rid of the text that’s in them.
Next, single-click the View icon in the nib dock and bring up the attributes inspector (see Figure 17–2). On the inspector, go to the View section and make sure that both User Interaction Enabled and Multiple Touch are checked. If Multiple Touch is not checked, your controller class’s touch methods will always receive one and only one touch, no matter how many fingers are actually touching the phone’s screen.
www.it-ebooks.info
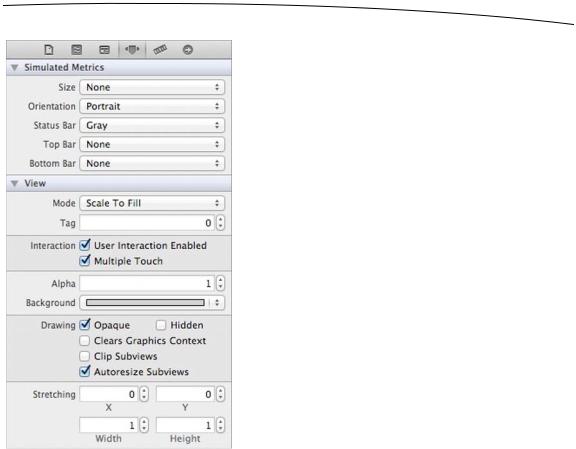
CHAPTER 17: Taps, Touches, and Gestures |
611 |
Figure 17–2. In the View attributes, make sure both User Interaction Enabled and Multiple Touch are checked.
When you’re finished, save the nib. Next, select BIDViewController.m, and add the following code at the beginning of the file:
#import "BIDViewController.h"
@implementation BIDViewController
@synthesize messageLabel; @synthesize tapsLabel; @synthesize touchesLabel;
- (void)updateLabelsFromTouches:(NSSet *)touches { NSUInteger numTaps = [[touches anyObject] tapCount]; NSString *tapsMessage = [[NSString alloc]
initWithFormat:@"%d taps detected", numTaps]; tapsLabel.text = tapsMessage;
NSUInteger numTouches = [touches count];
NSString *touchMsg = [[NSString alloc] initWithFormat: @"%d touches detected", numTouches];
touchesLabel.text = touchMsg;
}
.
www.it-ebooks.info

612CHAPTER 17: Taps, Touches, and Gestures
.
.
Then insert the following lines of code into the existing viewDidUnload methods:
- (void)viewDidUnload { [super viewDidUnload];
//Release any retained subviews of the main view.
//e.g. self.myOutlet = nil;
self.messageLabel = nil; self.tapsLabel = nil; self.touchesLabel = nil;
}
And add the following new methods at the end of the file:
#pragma mark -
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event { messageLabel.text = @"Touches Began";
[self updateLabelsFromTouches:touches];
}
- (void)touchesCancelled:(NSSet *)touches withEvent:(UIEvent *)event{ messageLabel.text = @"Touches Cancelled";
[self updateLabelsFromTouches:touches];
}
- (void)touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event { messageLabel.text = @"Touches Ended.";
[self updateLabelsFromTouches:touches];
}
- (void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event { messageLabel.text = @"Drag Detected";
[self updateLabelsFromTouches:touches];
}
@end
In this controller class, we implement all four of the touch-related methods we discussed earlier. Each one sets messageLabel so the user can see when each method is called. Next, all four of them call updateLabelsFromTouches: to update the other two labels. The updateLabelsFromTouches: method gets the tap count from one of the touches, figures out the number of touches by looking at the count of the touches set, and updates the labels with that information.
Compile and run the application. If you’re running in the simulator, try repeatedly clicking the screen to drive up the tap count, and try clicking and holding down the mouse button while dragging around the view to simulate a touch and drag. Note that a drag is not the same as a tap, so once you start your drag, the app will report zero taps.
You can emulate a two-finger pinch in the iOS simulator by holding down the option key while you click with the mouse and drag. You can also simulate two-finger swipes by first holding down the option key to simulate a pinch, then moving the mouse so the two dots representing virtual fingers are next to each other, and then holding down the shift
www.it-ebooks.info