
- •Contents at a Glance
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Preface
- •What This Book Is
- •What You Need
- •Developer Options
- •What You Need to Know
- •What’s Different About Coding for iOS?
- •Only One Active Application
- •Only One Window
- •Limited Access
- •Limited Response Time
- •Limited Screen Size
- •Limited System Resources
- •No Garbage Collection, but…
- •Some New Stuff
- •A Different Approach
- •What’s in This Book
- •What’s New in This Update?
- •Are You Ready?
- •Setting Up Your Project in Xcode
- •The Xcode Workspace Window
- •The Toolbar
- •The Navigator View
- •The Jump Bar
- •The Utility Pane
- •Interface Builder
- •New Compiler and Debugger
- •A Closer Look at Our Project
- •Introducing Xcode’s Interface Builder
- •What’s in the Nib File?
- •The Library
- •Adding a Label to the View
- •Changing Attributes
- •Some iPhone Polish—Finishing Touches
- •Bring It on Home
- •The Model-View-Controller Paradigm
- •Creating Our Project
- •Looking at the View Controller
- •Understanding Outlets and Actions
- •Outlets
- •Actions
- •Cleaning Up the View Controller
- •Designing the User Interface
- •Adding the Buttons and Action Method
- •Adding the Label and Outlet
- •Writing the Action Method
- •Trying It Out
- •Looking at the Application Delegate
- •Bring It on Home
- •A Screen Full of Controls
- •Active, Static, and Passive Controls
- •Creating the Application
- •Implementing the Image View and Text Fields
- •Adding the Image View
- •Resizing the Image View
- •Setting View Attributes
- •The Mode Attribute
- •Interaction Checkboxes
- •The Alpha Value
- •Background
- •Drawing Checkboxes
- •Stretching
- •Adding the Text Fields
- •Text Field Inspector Settings
- •Setting the Attributes for the Second Text Field
- •Creating and Connecting Outlets
- •Closing the Keyboard
- •Closing the Keyboard When Done Is Tapped
- •Touching the Background to Close the Keyboard
- •Adding the Slider and Label
- •Creating and Connecting the Actions and Outlets
- •Implementing the Action Method
- •Adding Two Labeled Switches
- •Connecting and Creating Outlets and Actions
- •Implementing the Switch Actions
- •Adding the Button
- •Connecting and Creating the Button Outlets and Actions
- •Implementing the Segmented Control Action
- •Implementing the Action Sheet and Alert
- •Conforming to the Action Sheet Delegate Method
- •Showing the Action Sheet
- •Spiffing Up the Button
- •Using the viewDidLoad Method
- •Control States
- •Stretchable Images
- •Crossing the Finish Line
- •The Mechanics of Autorotation
- •Points, Pixels, and the Retina Display
- •Autorotation Approaches
- •Handling Rotation Using Autosize Attributes
- •Configuring Supported Orientations
- •Specifying Rotation Support
- •Designing an Interface with Autosize Attributes
- •Using the Size Inspector’s Autosize Attributes
- •Setting the Buttons’ Autosize Attributes
- •Restructuring a View When Rotated
- •Creating and Connecting Outlets
- •Moving the Buttons on Rotation
- •Swapping Views
- •Designing the Two Views
- •Implementing the Swap
- •Changing Outlet Collections
- •Rotating Out of Here
- •Common Types of Multiview Apps
- •The Architecture of a Multiview Application
- •The Root Controller
- •Anatomy of a Content View
- •Building View Switcher
- •Creating Our View Controller and Nib Files
- •Modifying the App Delegate
- •Modifying BIDSwitchViewController.h
- •Adding a View Controller
- •Building a View with a Toolbar
- •Writing the Root View Controller
- •Implementing the Content Views
- •Animating the Transition
- •Switching Off
- •The Pickers Application
- •Delegates and Data Sources
- •Setting Up the Tab Bar Framework
- •Creating the Files
- •Adding the Root View Controller
- •Creating TabBarController.xib
- •The Initial Test Run
- •Implementing the Date Picker
- •Implementing the Single-Component Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller As a Data Source and Delegate
- •Implementing a Multicomponent Picker
- •Declaring Outlets and Actions
- •Building the View
- •Implementing the Controller
- •Implementing Dependent Components
- •Creating a Simple Game with a Custom Picker
- •Writing the Controller Header File
- •Building the View
- •Adding Image Resources
- •Implementing the Controller
- •The spin Method
- •The viewDidLoad Method
- •Final Details
- •Linking in the Audio Toolbox Framework
- •Final Spin
- •Table View Basics
- •Table Views and Table View Cells
- •Grouped and Plain Tables
- •Implementing a Simple Table
- •Designing the View
- •Writing the Controller
- •Adding an Image
- •Using Table View Cell Styles
- •Setting the Indent Level
- •Handling Row Selection
- •Changing the Font Size and Row Height
- •Customizing Table View Cells
- •Adding Subviews to the Table View Cell
- •Creating a UITableViewCell Subclass
- •Adding New Cells
- •Implementing the Controller’s Code
- •Loading a UITableViewCell from a Nib
- •Designing the Table View Cell in Interface Builder
- •Using the New Table View Cell
- •Grouped and Indexed Sections
- •Building the View
- •Importing the Data
- •Implementing the Controller
- •Adding an Index
- •Implementing a Search Bar
- •Rethinking the Design
- •A Deep Mutable Copy
- •Updating the Controller Header File
- •Modifying the View
- •Modifying the Controller Implementation
- •Copying Data from allNames
- •Implementing the Search
- •Changes to viewDidLoad
- •Changes to Data Source Methods
- •Adding a Table View Delegate Method
- •Adding Search Bar Delegate Methods
- •Adding a Magnifying Glass to the Index
- •Adding the Special Value to the Keys Array
- •Suppressing the Section Header
- •Telling the Table View What to Do
- •Putting It All on the Table
- •Navigation Controller Basics
- •Stacky Goodness
- •A Stack of Controllers
- •Nav, a Hierarchical Application in Six Parts
- •Meet the Subcontrollers
- •The Disclosure Button View
- •The Checklist View
- •The Rows Control View
- •The Movable Rows View
- •The Deletable Rows View
- •The Editable Detail View
- •The Nav Application’s Skeleton
- •Creating the Top-Level View Controller
- •Setting Up the Navigation Controller
- •Adding the Images to the Project
- •First Subcontroller: The Disclosure Button View
- •Creating the Detail View
- •Modifying the Disclosure Button Controller
- •Adding a Disclosure Button Controller Instance
- •Second Subcontroller: The Checklist
- •Creating the Checklist View
- •Adding a Checklist Controller Instance
- •Third Subcontroller: Controls on Table Rows
- •Creating the Row Controls View
- •Adding a Rows Control Controller Instance
- •Fourth Subcontroller: Movable Rows
- •Creating the Movable Row View
- •Adding a Move Me Controller Instance
- •Fifth Subcontroller: Deletable Rows
- •Creating the Deletable Rows View
- •Adding a Delete Me Controller Instance
- •Sixth Subcontroller: An Editable Detail Pane
- •Creating the Data Model Object
- •Creating the Detail View List Controller
- •Creating the Detail View Controller
- •Adding an Editable Detail View Controller Instance
- •But There’s One More Thing. . .
- •Breaking the Tape
- •Creating a Simple Storyboard
- •Dynamic Prototype Cells
- •Dynamic Table Content, Storyboard-Style
- •Editing Prototype Cells
- •Good Old Table View Data Source
- •Will It Load?
- •Static Cells
- •Going Static
- •So Long, Good Old Table View Data Source
- •You Say Segue, I Say Segue
- •Creating Segue Navigator
- •Filling the Blank Slate
- •First Transition
- •A Slightly More Useful Task List
- •Viewing Task Details
- •Make More Segues, Please
- •Passing a Task from the List
- •Handling Task Details
- •Passing Back Details
- •Making the List Receive the Details
- •If Only We Could End with a Smooth Transition
- •Split Views and Popovers
- •Creating a SplitView Project
- •The Storyboard Defines the Structure
- •The Code Defines the Functionality
- •The App Delegate
- •The Master View Controller
- •The Detail View Controller
- •Here Come the Presidents
- •Creating Your Own Popover
- •iPad Wrap-Up
- •Getting to Know Your Settings Bundle
- •The AppSettings Application
- •Creating the Project
- •Working with the Settings Bundle
- •Adding a Settings Bundle to Our Project
- •Setting Up the Property List
- •Adding a Text Field Setting
- •Adding an Application Icon
- •Adding a Secure Text Field Setting
- •Adding a Multivalue Field
- •Adding a Toggle Switch Setting
- •Adding the Slider Setting
- •Adding Icons to the Settings Bundle
- •Adding a Child Settings View
- •Reading Settings in Our Application
- •Retrieving User Settings
- •Creating the Main View
- •Updating the Main View Controller
- •Registering Default Values
- •Changing Defaults from Our Application
- •Keeping It Real
- •Beam Me Up, Scotty
- •Your Application’s Sandbox
- •Getting the Documents Directory
- •Getting the tmp Directory
- •File-Saving Strategies
- •Single-File Persistence
- •Multiple-File Persistence
- •Using Property Lists
- •Property List Serialization
- •The First Version of the Persistence Application
- •Creating the Persistence Project
- •Designing the Persistence Application View
- •Editing the Persistence Classes
- •Archiving Model Objects
- •Conforming to NSCoding
- •Implementing NSCopying
- •Archiving and Unarchiving Data Objects
- •The Archiving Application
- •Implementing the BIDFourLines Class
- •Implementing the BIDViewController Class
- •Using iOS’s Embedded SQLite3
- •Creating or Opening the Database
- •Using Bind Variables
- •The SQLite3 Application
- •Linking to the SQLite3 Library
- •Modifying the Persistence View Controller
- •Using Core Data
- •Entities and Managed Objects
- •Key-Value Coding
- •Putting It All in Context
- •Creating New Managed Objects
- •Retrieving Managed Objects
- •The Core Data Application
- •Designing the Data Model
- •Creating the Persistence View and Controller
- •Persistence Rewarded
- •Managing Document Storage with UIDocument
- •Building TinyPix
- •Creating BIDTinyPixDocument
- •Code Master
- •Initial Storyboarding
- •Creating BIDTinyPixView
- •Storyboard Detailing
- •Adding iCloud Support
- •Creating a Provisioning Profile
- •Enabling iCloud Entitlements
- •How to Query
- •Save Where?
- •Storing Preferences on iCloud
- •What We Didn’t Cover
- •Grand Central Dispatch
- •Introducing SlowWorker
- •Threading Basics
- •Units of Work
- •GCD: Low-Level Queueing
- •Becoming a Blockhead
- •Improving SlowWorker
- •Don’t Forget That Main Thread
- •Giving Some Feedback
- •Concurrent Blocks
- •Background Processing
- •Application Life Cycle
- •State-Change Notifications
- •Creating State Lab
- •Exploring Execution States
- •Making Use of Execution State Changes
- •Handling the Inactive State
- •Handling the Background State
- •Removing Resources When Entering the Background
- •Saving State When Entering the Background
- •A Brief Journey to Yesteryear
- •Back to the Background
- •Requesting More Backgrounding Time
- •Grand Central Dispatch, Over and Out
- •Two Views of a Graphical World
- •The Quartz 2D Approach to Drawing
- •Quartz 2D’s Graphics Contexts
- •The Coordinate System
- •Specifying Colors
- •A Bit of Color Theory for Your iOS Device’s Display
- •Other Color Models
- •Color Convenience Methods
- •Drawing Images in Context
- •Drawing Shapes: Polygons, Lines, and Curves
- •The QuartzFun Application
- •Setting Up the QuartzFun Application
- •Creating a Random Color
- •Defining Application Constants
- •Implementing the QuartzFunView Skeleton
- •Creating and Connecting Outlets and Actions
- •Implementing the Action Methods
- •Adding Quartz 2D Drawing Code
- •Drawing the Line
- •Drawing the Rectangle and Ellipse
- •Drawing the Image
- •Optimizing the QuartzFun Application
- •The GLFun Application
- •Setting Up the GLFun Application
- •Creating BIDGLFunView
- •Updating BIDViewController
- •Updating the Nib
- •Finishing GLFun
- •Drawing to a Close
- •Multitouch Terminology
- •The Responder Chain
- •Responding to Events
- •Forwarding an Event: Keeping the Responder Chain Alive
- •The Multitouch Architecture
- •The Four Touch Notification Methods
- •The TouchExplorer Application
- •The Swipes Application
- •Automatic Gesture Recognition
- •Implementing Multiple Swipes
- •Detecting Multiple Taps
- •Detecting Pinches
- •Defining Custom Gestures
- •The CheckPlease Application
- •The CheckPlease Touch Methods
- •Garçon? Check, Please!
- •The Location Manager
- •Setting the Desired Accuracy
- •Setting the Distance Filter
- •Starting the Location Manager
- •Using the Location Manager Wisely
- •The Location Manager Delegate
- •Getting Location Updates
- •Getting Latitude and Longitude Using CLLocation
- •Error Notifications
- •Trying Out Core Location
- •Updating Location Manager
- •Determining Distance Traveled
- •Wherever You Go, There You Are
- •Accelerometer Physics
- •Don’t Forget Rotation
- •Core Motion and the Motion Manager
- •Event-Based Motion
- •Proactive Motion Access
- •Accelerometer Results
- •Detecting Shakes
- •Baked-In Shaking
- •Shake and Break
- •Accelerometer As Directional Controller
- •Rolling Marbles
- •Writing the Ball View
- •Calculating Ball Movement
- •Rolling On
- •Using the Image Picker and UIImagePickerController
- •Implementing the Image Picker Controller Delegate
- •Road Testing the Camera and Library
- •Designing the Interface
- •Implementing the Camera View Controller
- •It’s a Snap!
- •Localization Architecture
- •Strings Files
- •What’s in a Strings File?
- •The Localized String Macro
- •Real-World iOS: Localizing Your Application
- •Setting Up LocalizeMe
- •Trying Out LocalizeMe
- •Localizing the Nib
- •Localizing an Image
- •Generating and Localizing a Strings File
- •Localizing the App Display Name
- •Auf Wiedersehen
- •Apple’s Documentation
- •Mailing Lists
- •Discussion Forums
- •Web Sites
- •Blogs
- •Conferences
- •Follow the Authors
- •Farewell
- •Index
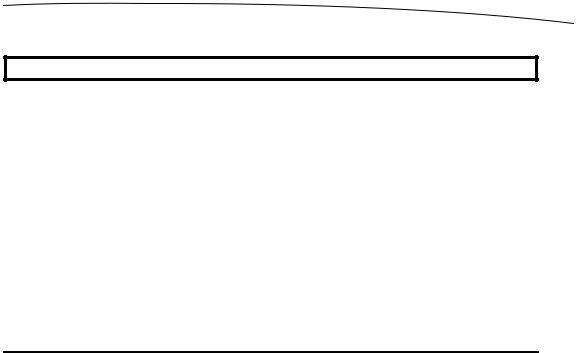
CHAPTER 7: Tab Bars and Pickers |
205 |
Fonts Supported by iOS Devices
Be careful when using the fonts palette in Interface Builder for designing iOS interfaces. The attribute inspector’s font selector will let you assign from a wide range of fonts, but not all iOS devices have the same set of fonts available. At the time of this writing, for instance, there are several fonts that are available on the iPad, but not on the iPhone or iPod touch. You should limit your font selections to one of the font families found on the iOS device you are targeting. This post on Jeff LaMarche’s excellent iOS blog shows you how to grab this list programmatically: http://iphonedevelopment.blogspot.com/ 2010/08/fonts-and-font-families.html.
In a nutshell, create a view-based application and add this code to the method application:didFinishLaunchingWithOptions: in the application delegate:
for (NSString *family in [UIFont familyNames]) { NSLog(@"%@", family);
for (NSString *font in [UIFont fontNamesForFamilyName:family]) { NSLog(@"\t%@", font);
}
}
Run the project in the appropriate simulator, and your fonts will be displayed in the project’s console log.
Adding Image Resources
Now we need to add the images that we’ll be using in our game. We’ve included a set of six image files (seven.png, bar.png, crown.png, cherry.png, lemon.png, and apple.png) for you in the project archive under the 07 Pickers/Custom Picker Images folder. Add all of those files to your project by dragging the entire folder into the Pickers folder in Xcode, just as you did for the tab bar icons. It’s probably a good idea to copy them into the project folder when prompted to do so.
Implementing the Controller
We have a bunch of new stuff to cover in the implementation of this controller. Add the following code at the beginning of BIDCustomPickerViewController.m file:
#import "BIDCustomPickerViewController.h"
@implementation BIDCustomPickerViewController
@synthesize picker; @synthesize winLabel; @synthesize column1; @synthesize column2; @synthesize column3; @synthesize column4; @synthesize column5;
- (IBAction)spin { BOOL win = NO;
www.it-ebooks.info

206 |
CHAPTER 7: Tab Bars and Pickers |
||
|
int numInRow |
= 1; |
|
|
int |
lastVal = -1; |
|
|
for |
(int i = |
0; i < 5; i++) { |
int newValue = random() % [self.column1 count];
if (newValue == lastVal) numInRow++;
else
numInRow = 1;
lastVal = newValue;
[picker selectRow:newValue inComponent:i animated:YES]; [picker reloadComponent:i];
if (numInRow >= 3) win = YES;
}
if (win)
winLabel.text = @"WIN!";
else
winLabel.text = @"";
}
.
.
.
Then, insert the following code into the viewDidLoad method:
- (void)viewDidLoad { [super viewDidLoad];
// Do any additional setup after loading the view from its nib.
UIImage *seven = [UIImage imageNamed:@"seven.png"]; UIImage *bar = [UIImage imageNamed:@"bar.png"]; UIImage *crown = [UIImage imageNamed:@"crown.png"]; UIImage *cherry = [UIImage imageNamed:@"cherry.png"]; UIImage *lemon = [UIImage imageNamed:@"lemon.png"]; UIImage *apple = [UIImage imageNamed:@"apple.png"];
for (int i = 1; i <= 5; i++) {
UIImageView *sevenView = [[UIImageView alloc] initWithImage:seven]; UIImageView *barView = [[UIImageView alloc] initWithImage:bar]; UIImageView *crownView = [[UIImageView alloc] initWithImage:crown]; UIImageView *cherryView = [[UIImageView alloc]
initWithImage:cherry];
UIImageView *lemonView = [[UIImageView alloc] initWithImage:lemon]; UIImageView *appleView = [[UIImageView alloc] initWithImage:apple]; NSArray *imageViewArray = [[NSArray alloc] initWithObjects:
sevenView, barView, crownView, cherryView, lemonView, appleView, nil];
NSString *fieldName =
[[NSString alloc] initWithFormat:@"column%d", i]; [self setValue:imageViewArray forKey:fieldName];
}
srandom(time(NULL));
www.it-ebooks.info

CHAPTER 7: Tab Bars and Pickers |
207 |
}
Next, insert the following new lines into the viewDidUnload method:
- (void)viewDidUnload { [super viewDidUnload];
//Release any retained subviews of the main view.
//e.g. self.myOutlet = nil;
self.picker = nil; self.winLabel = nil; self.column1 = nil; self.column2 = nil; self.column3 = nil; self.column4 = nil; self.column5 = nil;
}
Finally, add the following code to the end of the file:
.
.
.
#pragma mark -
#pragma mark Picker Data Source Methods
- (NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView { return 5;
}
- (NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component { return [self.column1 count];
}
#pragma mark Picker Delegate Methods
- (UIView *)pickerView:(UIPickerView *)pickerView viewForRow:(NSInteger)row
forComponent:(NSInteger)component reusingView:(UIView *)view { NSString *arrayName = [[NSString alloc] initWithFormat:@"column%d",
component+1];
NSArray *array = [self valueForKey:arrayName]; return [array objectAtIndex:row];
}
@end
There’s a lot going on here, huh? Let’s take the new stuff method by method.
The spin Method
The spin method fires when the user touches the Spin button. In it, we first declare a few variables that will help us keep track of whether the user has won. We’ll use win to keep track of whether we’ve found three in a row by setting it to YES if we have. We’ll use numInRow to keep track of how many of the same value we have in a row so far, and we will keep track of the previous component’s value in lastVal so that we have a way
www.it-ebooks.info

208 |
CHAPTER 7: Tab Bars and Pickers |
to compare the current value to the previous value. We initialize lastVal to -1 because we know that value won’t match any of the real values.
BOOL win = NO; int numInRow = 1; int lastVal = -1;
Next, we loop through all five components and set each one to a new, randomly generated row selection. We get the count from the column1 array to do that, which is a shortcut we can use because we know that all five columns have the same number of values.
for (int i = 0; i < 5; i++) {
int newValue = random() % [self.column1 count];
We compare the new value to the previous value and increment numInRow if it matches. If the value didn’t match, we reset numInRow back to 1. We then assign the new value to lastVal so we’ll have it to compare the next time through the loop.
if (newValue == lastVal) numInRow++;
else
numInRow = 1; lastVal = newValue;
After that, we set the corresponding component to the new value, telling it to animate the change, and we tell the picker to reload that component.
[picker selectRow:newValue inComponent:i animated:YES]; [picker reloadComponent:i];
The last thing we do each time through the loop is check whether we have three in a row, and set win to YES if we do.
if (numInRow >= 3) win = YES;
}
Once we’re finished with the loop, we set the label to say whether the spin was a win.
if (win)
winLabel.text = @"Win!";
else
winLabel.text = @"";
The viewDidLoad Method
The new version of viewDidLoad is somewhat scary looking, isn’t it? Don’t worry—once we break it down, it won’t seem quite so much like the monster in your closet.
The first thing we do is load six different images. We do this using a convenience method on the UIImage class called imageNamed:.
UIImage *seven = [UIImage imageNamed:@"seven.png"];
UIImage *bar = [UIImage imageNamed:@"bar.png"];
UIImage *crown = [UIImage imageNamed:@"crown.png"];
UIImage *cherry = [UIImage imageNamed:@"cherry.png"];
www.it-ebooks.info

CHAPTER 7: Tab Bars and Pickers |
209 |
UIImage *lemon = [UIImage imageNamed:@"lemon.png"];
UIImage *apple = [UIImage imageNamed:@"apple.png"];
Once we have the six images loaded, we then need to create instances of UIImageView, one for each image, for each of the five picker components. We do that in a loop.
for (int i = 1; |
i <= 5; i++) |
{ |
|
UIImageView |
*sevenView |
= |
[[UIImageView alloc] initWithImage:seven]; |
UIImageView |
*barView = |
[[UIImageView alloc] initWithImage:bar]; |
|
UIImageView |
*crownView |
= |
[[UIImageView alloc] initWithImage:crown]; |
UIImageView |
*cherryView = [[UIImageView alloc] |
||
initWithImage:cherry]; |
|||
UIImageView |
*lemonView |
= [[UIImageView alloc] initWithImage:lemon]; |
|
UIImageView |
*appleView |
= |
[[UIImageView alloc] initWithImage:apple]; |
After we have the image views, we put them into an array. This array is the one that will be used to provide data to the picker for one of its five components.
NSArray *imageViewArray = [[NSArray alloc] initWithObjects: sevenView, barView, crownView, cherryView, lemonView, appleView, nil];
Now, we just need to assign this array to one of our five arrays. To do that, we create a string that matches the name of one of the arrays. The first time through the loop, this string will be column1, which is the name of the array we’ll use to feed the first component in the picker. The second time through, it will equal column2, and so on.
NSString *fieldName = [[NSString alloc] initWithFormat:@"column%d", i];
Once we have the name of one of the five arrays, we can assign this array to that property using a very handy method called setValue:forKey:. This method lets you set a property based on its name. So, if we call this with a value of "column1", it is exactly the same as calling the mutator method setColumn1:.
[self setValue:imageViewArray forKey:fieldName];
The last thing we do in this method is to seed the random number generator. If we don’t do that, the game will play the same way every time, which gets kind of boring.
srandom(time(NULL));
}
That wasn’t so bad, was it? But, um, what do we do with those five arrays now that we’ve filled them with image views? If you scroll down through the code you just typed, you’ll see that two data source methods look pretty much the same as before, but if you look down further into the delegate methods, you’ll see that we’re using a completely different delegate method to provide data to the picker. The one that we’ve used up to now returned an NSString *, but this one returns a UIView *.
Using this method instead, we can supply the picker with anything that can be drawn into a UIView. Of course, there are limitations on what will work here and look good at the same time, given the small size of the picker. But this method gives us a lot more freedom in what we display, although it is a bit more work.
- (UIView *)pickerView:(UIPickerView *)pickerView viewForRow:(NSInteger)row
www.it-ebooks.info